How to Shuffle an Array in JavaScript?
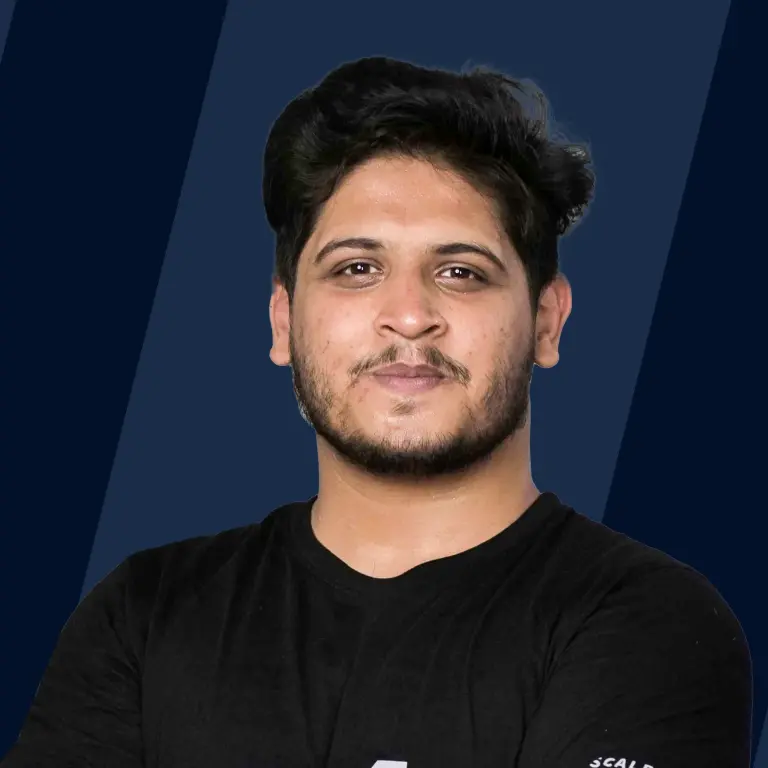
Overview
Shuffling is one of the oldest problems in computer science. Randomly arranging the elements of an array is called shuffling. In this article, we will see how to implement shuffling in JavaScript.
Introduction
Shuffling an array means randomly arranging the elements of an array. It is one of the oldest problems in computer science.
Ways To Shuffle An Array In Javascript
There are two ways of performing shuffling in JavaScript.
-
Custom sort: We pass a custom function as the comparator in the sort function. The sort function accepts a custom function that outputs a value between -0.5 and 0.5. Let's see an example to understand this better.
Output
Explanation We declare and initialize an array arr. We then call the sort function with a custom function as the comparator as shown in line 2.
A comparator represents a compare function determining which element comes before the other. Given two inputs a and b , if the output of the comparator function is positive, a comes before b in the sorted array. If the output of the comparator function is negative, b comes before a in the sorted array.
The range of the Math.random function is [0,1). So the range of our comparator function becomes (-0.5,0.5]. Therefore, using a random function in our comparator function, we can randomize the sequence of the elements.
-
The Fisher-Yates algorithm: This algorithm has the following steps.
- Traverse the elements of the array, one by one(from the end to the start).
- For each index i, generate a random integer j from 0 to i-1.
- Swap the element of index i and index j.
Output
Explanation
An array arr is declared and initialized in line 1. Then we traverse the element from the last index to the first, swapping the element at the current index with a random index element. We print the shuffled element in the console.
Conclusion
- Shuffling an array means randomly arranging the elements of the array.
- There are two ways of shuffling in JavaScript - custom sort and Fisher-Yates algorithm.
- The sort function accepts a custom function that outputs a value between -0.5 and 0.5.
- In the Fisher-Yates algorithm, we traverse the element from the last index to the first, swapping the current index element with a random index element of the array.