How to Find the Sum of Array in JavaScript?
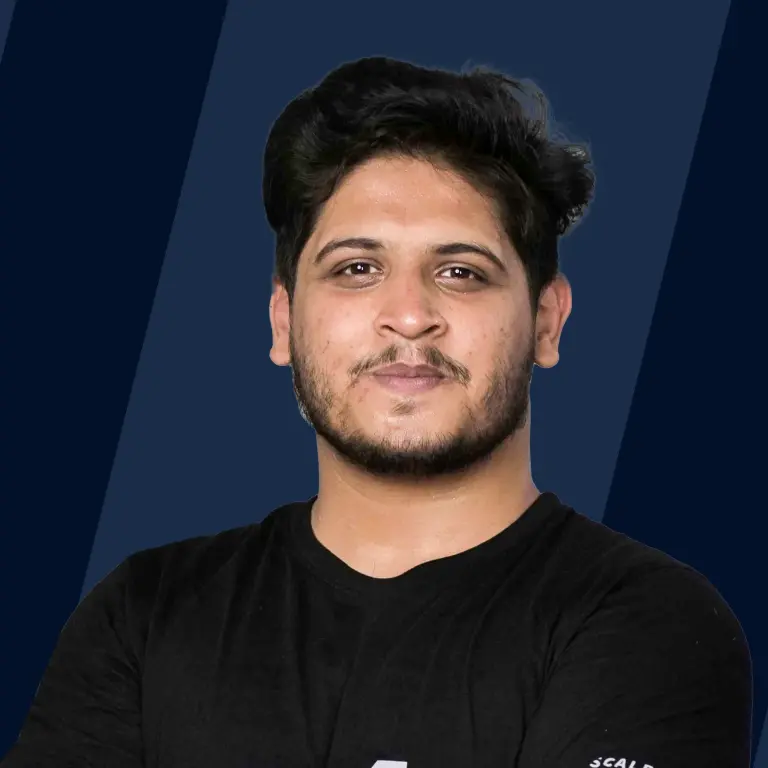
Overview
There are many different methods by which we can calculate a Javascript sum array. Some of the standard ways to retrieve sum array Javascript include traversing the array with For loop, For-of Loop, or the For-each method, which goes through each item in the array. These are in the order of linear time complexity. An advanced way is using the reduce() Method. For increased convenience and to make the code even shorter, we use the Iodash Library to calculate the sum of the array Javascript. In this article, we shall discuss all such methods and understand them with examples.
Scope
- This article covers the different methods to calculate the sum of the array in Javascript.
- They include For loop, forEach method, reduce method, for..of the loop, and Lodash library.
Introduction
Calculating the sum of array elements is a standard programming task in all languages. We need to know how it works to solve more complex problems in real-life software engineering. For now, your problems might be straightforward like calculating the average marks in a particular subject. If we represent the marks of all students in that subject with the help of an array, then the sum of all elements in that array divided by the number of students will give you the average. In the upcoming sections, we shall explore the different methods used to calculate the javascript sum array.
JavaScript Program to Find the Sum of Array Using For Loop
We can use the For Loop to iterate an array in Javascript. The loop would run from 0 to the end of the array. We use a sum variable, which is incremented inside the for loop. Here is the syntax:
Steps to be followed while calculating the sum using For Loop:
- We declare a sum variable and initialize it to 0.
- We traverse the array using a For Loop which runs from 0 to the end of the array
- We set the sum variable to the summation of its current value and the current element in every iteration.
- We exit the loop and our required sum is stored in the Sum variable.
Let us see the code for the same.
Upon executing the above code we get the following output:
JavaScript Program to Find the Sum of Array Using forEach Method
We can use the forEach method to calculate the Javascript sum array. It is a built-in array-like method in Javascript. Here is the syntax:
Here are the steps to be followed while calculating the sum using forEach:
- We declare a sum variable and initialize it to 0.
- We go through each item in the array using the forEach method.
- We set the sum variable to the summation of its current value and the current element in every iteration.
- Our required sum is stored in the Sum variable.
Let us see the code for the same.
Upon executing the above code we get the following output:
JavaScript Program to Find the Sum of Array Using reduce() Method
We use the reduce() method in Javascript to run a reducer function for array elements. The method returns a single value. This value is the total result accumulated by the function. Let us see the syntax of reduce() method in Javascript:
- function - This is the function that is run for individual elements in the array.
- total - This variable is used to store the total result, once the reducer function runs for all elements. This is a required parameter. Thus we must pass it while using the reduce method in Javascript. It contains the returned value from the final invocation of the reduce() method or the InitialValue if specified.
- currentValue - This is a required parameter. It represents the current element, for which the reducer function runs.
- currentIndex - This is an optional parameter. It represents the current index, for which the reducer function runs. The value of currentIndex is zero if initialValue` is specified. Else its value is one.
- initialValue - This is an optional parameter. It represents an initial value to be passed to the function. If this is not specified, then the first element will be used as the total value at the beginning and its value will get skipped for currentValue.
- return value - The accumulated value returned by the reducer function.
Let us see how we can use the reducer method to calculate the sum of the array javascript.
Upon executing the above code we get the following output:
JavaScript Program to Find the Sum of Array Using for..of
For..of in Javascript allows us to traverse an array, string, map, etc. It's syntax is as follows:
Here we assign the value of the property in every iteration as the variable. An Iterable is an object that contains iterable properties.
We can use the for..of the loop to find the sum of the array in Javascript.
Here are the steps to be followed while calculating the sum using for..of Loop:
- We declare a sum variable and initialize it to 0.
- We go through each item in the array using for..of loop and assign them in a variable.
- We set the sum variable to the summation of its current value and the variable in every iteration.
- Our required sum is stored in the Sum variable
Upon executing the above code we get the following output:
JavaScript Program to Find the Sum of Array Using the lodash Library
We can calculate the sum of Array Javascript using an in-built library called the Lodash Library. The Lodash Library is used in Javascript to work with arrays, strings, numbers, etc. This Library has a sum method, which helps to calculate array sum easily.
Using the Lodash Library we can calculate the sum of array in just a single line. Let us see the syntax for the same.
Upon executing the above code we get the following output:
Conclusion
- We can calculate the sum of the array in Javascript using many different methods.
- Some of the standard ways are traversing the array with loops and calculating the sum.
- A more advanced and efficient way is using the reduce() Method.
- For increased convenience and to make the code even shorter, we use the Lodash Library.