JavaScript Symbol
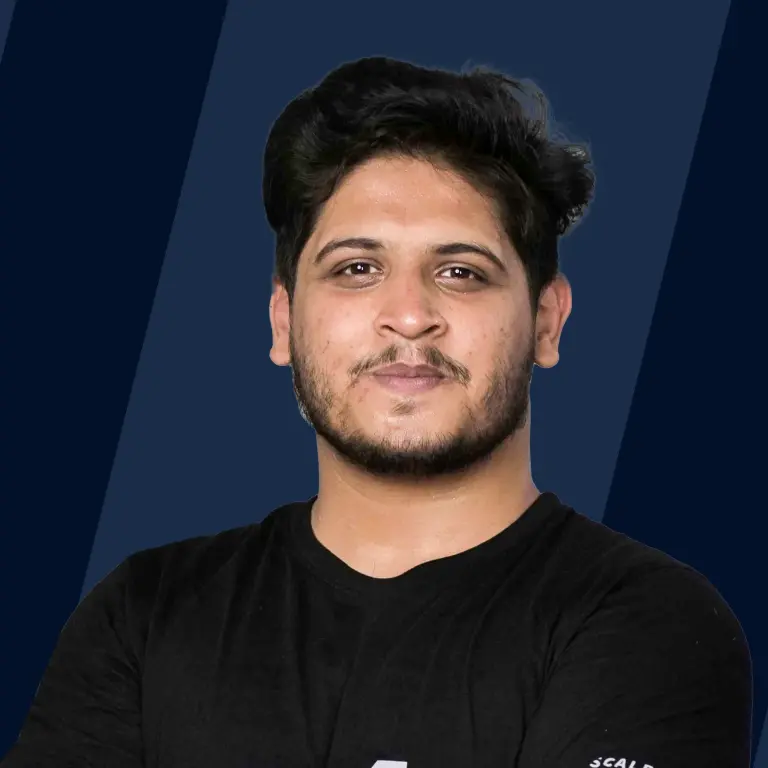
Overview
In this article, we are going to discuss JavaScript Symbol with Examples. JavaScript Symbol is a new primitive data type introduced in ES6 JavaScript. Along with integer, Boolean, string, null, and undefined, it is a primitive data type. By the end of this article, you will have a thorough understanding of the following points.
Introduction to JavaScript Symbol
Symbols in JavaScript are built-in objects whose constructor returns a symbol primitive that is guaranteed to be unique — also known as a Symbol value or simply a Symbol. JavaScript Symbol is frequently used to add unique property keys to an object that will not conflict with keys added by other code and that are hidden from any methods other code will usually use to access the object. This allows for a type of weak encapsulation or information hiding.
In each JavaScript Symbol() call will always return a unique Symbol. For any given value of key every Symbol.for("key") call will always return the same Symbol. Whenever Symbol.for("key") is called, it returns a Symbol with the given key if one is found in the global Symbol registry. Otherwise, a new Symbol is created, added with the given key in the global Symbol registry, and returned.
Syntax
Parameter
It is entirely optional. As an argument, we can use string.
Features of JavaScript Symbol
- A Symbol value is a one-of-a-kind identifier.
- Each symbol value is unique and unchangeable. Each symbol value is associated with a value of [description], which can be either undefined or a string.
- Symbol is a new primitive type in JavaScript introduced in ES6.
- var mySymbol = Symbol(), allows us to create our own symbols.
- For debugging purposes, we can add a description, such as var mySymbol = Symbol('JavaScript').
- Symbols are unchangeable and unique. Symbol() and Symbol('JavaScript') are distinct functions.
- Because symbols are of the type of symbol, typeof Symbol() ==='symbol'
- We can also use Symbol.for(key) to create global symbols.
- If a symbol with the supported key already existed, we retrieve it. Otherwise, a new symbol is created, with the key also serving as the description.
- Symbol.keyFor(symbol) is the inverse function, which takes a symbol and returns its key.
- Global symbols are as global as it gets, with global accessibility. A single registry is used to search these symbols throughout the runtime.
- There are also "popular" symbols.
- ES6 provides predefined symbols that already exist and are used internally by JavaScript, known as well-known symbols, which contain JavaScript's common behavior. Each well-known symbol is a Symbol object's static property.
- For array-like objects, use Symbol.iterator, and for string objects, use Symbol.search.
- Iterating over symbol properties is difficult, but not impossible, and it is certainly not private.
- Before ES6 Symbols are accessible via Object, they are hidden to all "reflection" methods.
- Object.getOwnPropertySymbols provides access to symbols.
JavaScript Symbol Property
Static Properties
Every static property has a well-known symbol. The semantics of an object's method, with this Symbol serving as the method name, is described by terminology like "Symbol.hasInstance," which does not describe the value of the Symbol itself.
Property | Description |
---|---|
Symbol.asyncIterator | Returns the object's default AsyncIterator and is used by await...of. |
Symbol.hasInstance | A method that determines whether or not a constructor object recognizes an object as its instance. Instanceof makes use of this. |
Symbol.isConcatSpreadable | A Boolean value that indicates whether or not an object's array elements must be flattened. Array.prototype.concat() uses this method. |
Symbol.iterator | A method that returns the object's default iterator. Used by for...of. |
Symbol.match | A method for matching against a string, as well as determining whether an object can be used as a regular expression. String.prototype.match() makes use of it. |
Symbol.matchAll | A method that returns an iterator containing regular expression matches against a string. String.prototype.matchAll() makes use of this. |
Symbol.replace | A method that replaces a string's matched substrings. String.prototype.replace() makes use of it. |
Symbol.search | This method returns the index within a string and matches the regular expression by using String.prototype.search() |
Symbol.split | A method for splitting a string at indices matching a regular expression. String.prototype.split() makes use of it. |
Symbol.species | A constructor function for creating derived objects. |
Symbol.toPrimitive | A method for transforming an object into a primitive value. |
Symbol.toStringTag | A string value used as an object's default description. Object.prototype.toString() uses this method. |
Symbol.unscopables | An object value whose own and inherited property identities are not included in the environment bindings of the linked object. |
Instance Properties
This method returns the index within a string and matches the regular expression by String.prototype.search()
Property | Description |
---|---|
Symbol.prototype[@@toStringTag] | The string "Symbol" is set as the @@toStringTag property's default value. This property is used in Object.prototype.toString (). Nevertheless, because Symbol has its own function toString() method, However, this property is only operative when Object.prototype.toString.call() is called with the symbol thisArg. |
Symbol.prototype.description | A read-only string containing the Symbol's description. |
JavaScript Symbol Methods
Static Methods
Method | Description |
---|---|
Symbol.for(key) | If an existing Symbol with the given key is found, it is returned. Otherwise, a new Symbol with key is formed in the global Symbol registry. |
Symbol.keyFor(sym) | Retrieves a shared Symbol key from the global Symbol registry for the given Symbol. |
Instance Methods
Method | Description |
---|---|
Symbol.prototype.toString() | The description of the Symbol is returned as a string. The method Object.prototype.toString() is overridden. |
Symbol.prototype.valueOf() | The Symbol is returned. Object.prototype.valueOf() method is overridden. |
Symbol.prototype[@@toPrimitive]() | Returns the Symbol. |
JavaScript Symbol Examples
A) Using the typeof operator with Symbols:
Symbols can be identified using the typeof operator.
B) Symbol type conversions: Some things to keep in mind when working with Symbol type conversion.
- When attempting to convert a Symbol to a number, a TypeError (e.g. +sym or sym | 0) will be thrown.
- Object(sym) == sym returns true when using loose equality.
- Symbol("foo") + "bar" raises a TypeError (Symbol cannot be converted to a string). This restricts you mutely from generating a new string property name from a Symbol silently.
- The "safer" String(sym) conversion works with Symbols in the same way that Symbol.prototype.toString() does, but new String(sym) will throw.
C) Symbols and for...in iteration:
In for...in iterations, symbols are not enumerable. Furthermore, Object.getOwnPropertyNames() does not return Symbol object properties; however, Object.getOwnPropertySymbols() does.
Output:
D) Symbols and JSON.stringify():
When using JSON.stringify(), symbol-keyed properties are completely ignored:
E) Symbol wrapper objects as property keys:
When a Symbol wrapper object is used as a property key, it is bound to the wrapped Symbol:
JavaScript Symbol Use Cases
When inheritance hierarchies exist in JavaScript, there are two types of properties (created either through classes, a purely prototypal approach):
Public: Clients of the code can see public properties.
Private: Private properties are used internally within the inheritance hierarchy's components (e.g. classes, objects).
String keys are typically used for public properties to improve usability. Unexpected name clashes can be a problem for private properties with string keys. Symbols are thus an excellent choice.
Creating JavaScript Symbol
A) Using symbols as unique values:
If you use a string or a number in your code, replace them with symbols. For instance, in the task management application, you must manage the status.
Before to ES6, you'd use strings like open, in progress, completed, canceled, and on hold to represent different task statuses. Symbols in ES6 can be used as follows:
B) Using symbol as the computed property name of an object:
Symbols can be used as computed property names. Consider the following example:
The Object.keys() method returns all of an object's enumerable properties.
The Object.getOwnPropertyNames() method is used to get all properties of an object, whether they are enumerable or not.
To get all of an object's property symbols, use the method, which was added in ES6.
The Object.getOwnPropertySymbols() method returns an array containing an object's own property symbols.
Well-Known Symbols in JavaScript
ES6 includes predefined symbols known as well-known symbols. The well-known symbols represent the most common JavaScript behaviors. Each well-known symbol is a Symbol object's static property.
Symbol.hasInstance
The symbol.hasInstance method checks whether a constructor object recognizes an object as its instance.
Output:
Symbol.iterator
The Symbol.iterator property determines whether a function returns an iterator for an object.
Output:
Symbol.isConcatSpreadable
Symbol.isConcatSpreadable specifies whether an object should be flattened to its array elements.
Example:
Output:
Symbol.toPrimitive
Symbol.toPrimitive is used to convert an object into its primitive value.
Example:
Output:
Symbol.split
Symbol.split splits a string at the indices specified by the regular expression.
Example:
Output:
Advantages and Disadvantages of Using Symbols in Object
Advantages of Using Symbols in Object:
If the same code snippet is used in multiple programs, use Symbols in the object key. Because you can avoid duplication by using the same key name in different codes. As an example,
If the person object in the preceding program is also used by another program, you wouldn't want to add a property that another program could access or change. As a result, by using Symbol, you create a unique property that you can use.
If the other program also needs to use a property named id, simply add a Symbol named id to avoid duplication. As an example,
Even if the same name is used to store values in the preceding program, the Symbol data type will have a unique value.
If the string key had been used in the preceding program, the later program would have changed the value of the property. As an example,
Disadvantages of Using Symbols in Object:
- Symbols lack the extensive collection of instance methods that the String class has.
- The majority of string usage involves modification, which symbols don't allow for (at least directly).
Browser Compatibility
Browser | Version |
---|---|
Google Chrome | 38 and higher |
Opera | 25 |
Firefox | 36 and higher |
Safari | 9 and higher |
Microsoft Edge | 13 and higher |
Deno | 1.0 |
Node.js | 0.12.0 |
Samsung Internet | 3.0 and higher |
Internet Explorer | 10 |
Conclusion
- A unique value is always returned by the Symbol() method.
- A symbol value can be used to identify object properties.
- Symbols, like numbers and strings, are immutable.
- Primitive data types cannot be typecasted to symbols.
- Many system symbols are used by JavaScript and are accessible as Symbol. We can use them to change some of the built-in behaviors.
- Symbols are created by calling Symbol() and providing an optional description (name).