Mobile Number Validation in Javascript
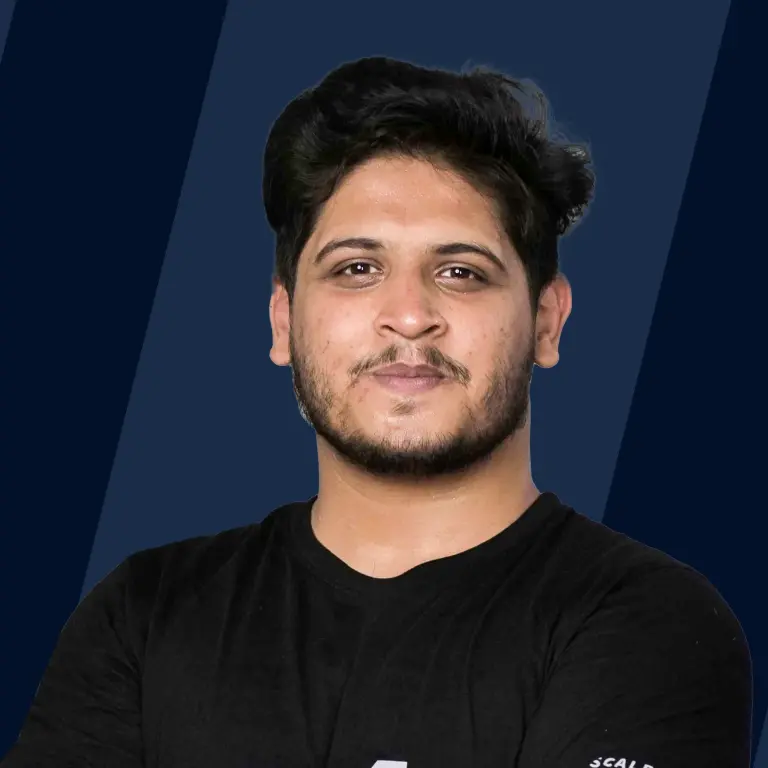
Overview
Whenever we are dealing with forms, or taking data from users on a web page, we require validation of certain fields to ensure that we are dealing with real users. However, this validation is very important when dealing with businesses where we need actual data of users to get back to them.
We can perform validation on almost all the fields. This validation can be validating the length of the name field, validating the correct syntax of the email field, validating the mobile number field, and many more.
In this article, we will be talking about validating the mobile number in a form of a web page in Javascript.
Introduction
The validation of a mobile number is very important in a webpage to ensure that we are dealing with a real user as well as getting back to the user for further purposes. There are different ways in which we can perform mobile number validation in javascript.
However, the main mistakes any user can make could be that they leave the field blank, entering illegal characters in the field, or enter a number whose length is more or less than 10. To check all these conditions, we use the if..else condition in javascript.
However, we can also use a regular expression to validate a phone number. We will write a regular expression and test it using the test() function to validate it in javascript.
How to Validate a Phone Number Using a JavaScript Regex?
The "Javascript Regex" refers to the regular expressions in Javascript. The regular expression is a sequence of characters that is used to specify a search pattern in a text. The text is searched with the pattern specified through regular expressions.
These regular expressions are used to perform certain "find" or "find and replace" operations on a string or for input validation.
For validating a phone number in Javascript, we will be using the following regular expression.
Now, let us break down the regex to understand it better.
- ^ :
It denotes the start of the expression. - d{3} :
It denoted a mandatory match of three digits in the text. - [- ]? :
It is used to denote an optional match of the hyphen and whitespace after the first three characters of the text. - (\d{3})[- ] :
It is the same as the previous expression therefore, it will work the same as the previous one which signifies a total of 6 digits now. - d{4} :
It is used to denote a mandatory of 4 digits after the 6 digits in the text. - $ :
It denotes the end of the expression.
The above regular expression will match all the mobile numbers like , , , and .
Now, let us see how to use this regular expression in Javascript.
Output :
In the above code example, we have made a function validateNumber() that contains the regular expression in a variable re. This regular expression pattern is then searched in the input string using the test() function and hence the output is printed. However, the test() function is used to test if there is a match in a string. If the pattern matches with the string then the test() function returns true otherwise false.
Validate a Phone Number Using a JavaScript Regex and HTML
We will now see how to validate a phone number using a javascript regex and HTML.
First of all, let's make a form that has a phone number field to be validated. After which, as soon as the form is submitted, a function is called to validate the form.
The below code snippet contains the HTML code to make a form.
Now, as soon as the form is submitted a function is called to validate the phone Number field. The function first extracts the input value from the form using the document.getElementById() method and stores it in a variable say number. Now the value stored in this variable is checked if it is valid or not using the regex expression in Javascript that was discussed in the previous subtopic.
However, if the regex function returns true then a message "Validation Successful" appears on the webpage otherwise an alert is popped to "Enter a valid number", after which the message "Validation failed" appears on the screen.
However, there is some CSS that has also been added to the messages appearing on the screen so that they are colored in green and red color.
Below is the full working code combining all the snippets discussed above to validate a phone number in HTML using the Javascript regex.
Output :
Validating a Phone Number with HTML5 “tel” Input
We can also validate a phone number with the HTML5 "tel" input. It is a much better way to validate a phone number as it also creates an input field for international numbers as well. Moreover, using the HTML5 "tel" input, the validation can be performed using a much shorter code.
The HTML5 "tel" input field can be denoted as follows :
As you can see, it uses the type="tel" attribute and a pattern attribute as well that is used while validating a phone number. Moreover, it allows smartphone browsers to optimize the input field and display a keyboard on the screen which will be convenient for entering only the phone numbers into the input field.
The complete code for validating a phone number using the HTML5 "tel" input is as follows :
Output :
Regional Phone Number Formats
It is very important to use the correct formats for the regional phone numbers as using the wrong formats can make your phone numbers data very difficult to handle manually by creating duplicate records, etc.
Therefore, below are some of the most commonly used regional number formats.
Cell Phone Numbers
The cell phone numbers in the united states consist of an 11-digit phone number. This 11-digit phone number is broken down as follows 1-digit country code, 3-digit area code, and the remaining 7 digits represent the telephone number.
North American Variations
The North American format of phone numbers is quite different from others. It consists of a 3-digit area code enclosed with parentheses. Further, you need to give a space and then a three-digit exchange code followed by a hyphen and a four-digit number.
UK Variations
The regional phone number format of the UK starts with a plus('+') symbol followed by the country code. After which you need to add the area code with a space and then the eight-digit phone number.
International Dialing Codes
While dealing with international phone numbers, you need to pay attention to the conventions that are followed in a particular country such as the exit codes, international prefixes, trunk prefixes, and much more.
The trunk prefixes are one of the common errors that a user makes while dealing with international numbers. It is a single-digit code that often starts with 0 and is added before the national subscriber number.
Another common error while dealing with international phone numbers is the area codes. Most countries do not use the area codes in their cell phone numbers instead they follow a unified national dialing format.
Such problems may occur while dealing with international cell phone numbers, therefore, we must take care of them. However, the international standards for the cell phone numbers of various countries have been listed in this Call prefixes article. You may refer to it for more information.
Why You should Validate Phone Number Input?
The validation of a phone number is very important for security reasons. It prevents you from various sign-up scams and fake customer registrations.
Moreover, you may adopt a one-time password verification facility to prevent yourself from any fake users. The OTP system will send a one-time password to the user for checking their accessibility and ensuring genuine users to your websites.
Validating Phone Number with an API
We can also validate a phone number with an API. It is a much easier way to validate the phone numbers as we are not required to write any code from scratch for the validation.
However, we just need an API key to validate a phone number. You can try using the NumVerify API for validating a phone number. It is a real-time REST API supporting almost 232 countries.
You just need the API key that will be assigned to you as soon as you sign up for the website of Numverify. To make an API request, you need to write the below statement :
However, the field YOUR_ACCESS_KEY is the API key itself. If the phone number validation request is successful then the result will look like this :
As you can see in the above output, the valid field is set as true which depicts that the number is validated otherwise it would have been set to false.
Conclusion
- The mobile number validation in javascript is a very important step to ensure security from scams and frauds.
- We can do mobile number validation in various ways : using javascript regular expressions, using HTML with javascript, using the HTML5 "tel" input, and using an API as well.
- However, using an API to validate a number makes your work easier and faster as you are not required to write any code from scratch.
- We must take care of the various regional code conventions and international dialing codes while validating a phone number.