What is Window Location href in JavaScript?
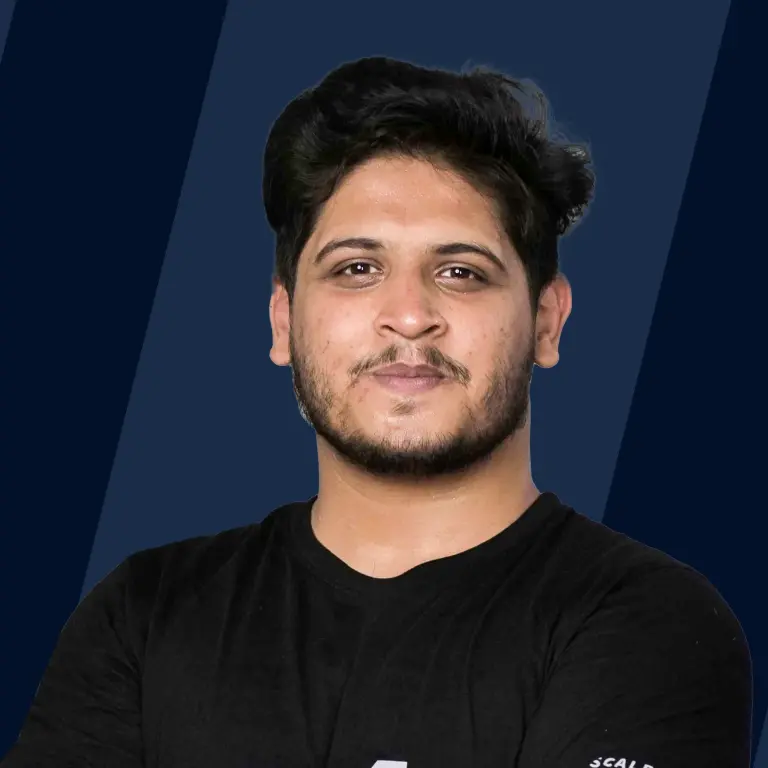
The location object in JavaScript has many different properties. The window.location.href property in JavaScript returns the URL of the currently open web page. This property can even be used to update the URL of a web page.
Syntax
Following is the syntax to display the current URL:
Following is the syntax to update the URL:
Here url is a string variable containing the new URL.
The Location Object
The location object is a property of the window object in JavaScript. The location object holds the information about the current URL of a web page. We can access the location object using window.location or just the keyword location.
The Location object has the following properties: hash, href, host, hostname, pathname, origin, port, search, and protocol. It also has three methods, namely assign(), replace(), and reload().
In this article, we will learn about the href property of the location object.
Getting the Current Page URL
The window.location.href property gives us the current page URL.
For example:
Output:
In the above example, we used window.location.href to store the current URL in a variable named url. We then used this variable to show the current URL.
We can even use window.location.href to load a new web page.
For example:
Output:
In the above example, we created a button that calls the openUrl() function once it is clicked. In the openUrl() function, we assigned the current URL a new URL, https://google.com. Whenever the button gets clicked, the current URL changes to https://google.com, and Google gets loaded on our web page.
Getting Different Parts of a URL
The href property of the location object gives us the complete URL of a web page. However, if we want specific components of a URL (like the hostname), we can use the other properties of the location object.
For example:
Output:
In the above example, we used different properties of the location object to display different components of a URL. The URL in this example did not have a search query or a fragment identifier, that's why these components remained empty in the output.
Loading New Documents
The assign() and the replace() methods in the location object are used to redirect the current web page to a new web page. Both these methods take one argument as input - the URL of the new web page.
The difference between the assign() and replace() methods is that if we use the replace() method to open a new URL, we can not go back to the old web page. The assign() method, on the other hand, lets us go back to the old web page if a new web page is opened.
Example 1: Using the replace() method
Output:
In the above example, we created a button with an onclick event. Upon clicking the button, the openUrl() function gets called. Then, the replace() method, defined inside the openUrl() function gets executed, and google.com is opened. Because we had defined a replace() method, once Google was open, we could not go back to the previous web page.
Example 2: Using the assign() method
Output:
In the above example, we created a button with an onclick event. Upon clicking the button, the openUrl() function gets called. Then, the assign() method, defined inside the openUrl() function gets executed, and google.com is opened. Because we had defined an assign() method, once Google was open, we were able to navigate back to the previous web page.
Reloading the Page Dynamically
The reload() method in the location object can be used to reload a page dynamically. This method works like the refresh button in web browsers. This method does not take any arguments. Use this Online Online JavaScript Formatter to format your code.
For example:
Output:
In the above example, we created a button, clicking upon which calls the openUrl() function. The openUrl() function contains the reload() method. Whenever we click the button, this reload() method gets called and the web page is reloaded.
Conclusion
- The location object comes with multiple properties that can be used to extract different components of a URL.
- The properties used to extract different elements of a URL are hash, href, host, hostname, pathname, origin, port, search, and protocol.
- The window.location.href property is used to extract the current URL from a webpage.
- The location object has three methods - assign(), replace(), and reload().
- The assign() and replace() methods are used to open a new URL on the web page.
- The reload() method is used to reload the web page.