jQuery UI - Effects
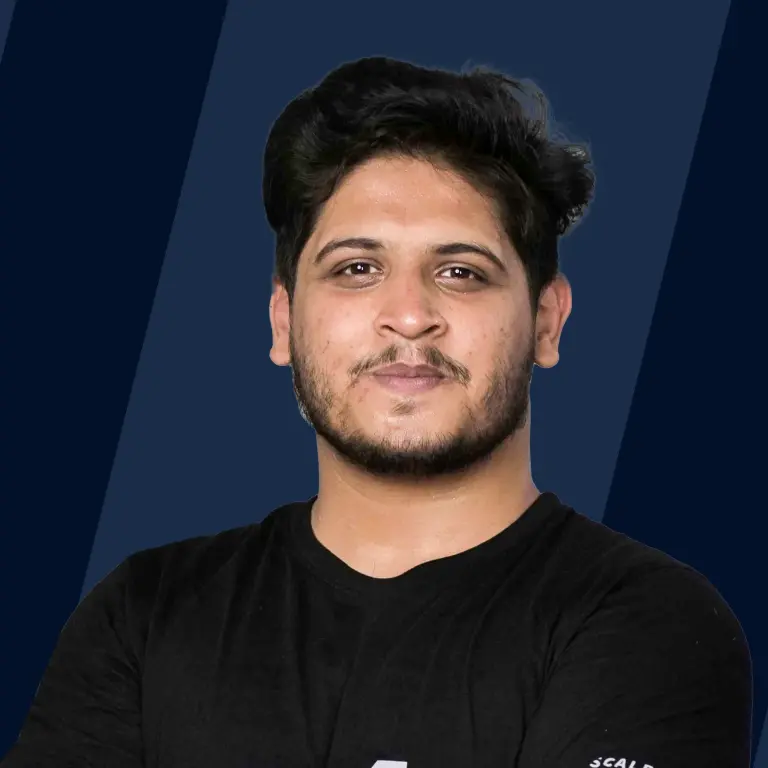
Overview
The effect() method, one of the methods used to handle jQueryUI visual effects, will be covered in this article. Without having to show or hide the element, the effect() method provides an animation effect.
Introduction to jQuery Effects
Six animation effects— hide(), show(), fadeOut(), fadeIn(), slideUp(), and slideDown() — as well as their toggle counterparts— toggle(), fadeToggle(), and slideToggle() — are all provided by jQuery.
That's a respectable set of effects to work with, but the jQuery UI team evidently didn't think so, and they've crammed at least 14 more animations into the Effects category.
Applying a jQuery UI Effect
Let's examine how to use the animations on an element before looking at the ones that are offered by jQuery. Using jQuery UI's effect() method is the simplest method.
Here is the syntax:
- selector: A jQuery selector that identifies the component of the web page you wish to interact with.
- effect: A string describing the jQuery UI effect you want to use on the element.
- options: A literal object containing one or more property-value pairs that identify the effect choices you want to apply. The most popular option, which sets the softening function, varies depending on the effect. More than 30 easings (functions specifying the speed at which an animation progresses at different points) are available in jQuery UI.
- duration: The milliseconds the effect lasts. Additionally, you can use the terms slow (600ms) or quick (equivalent to 200ms). 400ms is the default duration.
- After the effect has finished, jQuery UI calls the callback function function().
For instance, $('#my-div') applies the bounce effect from jQuery UI with a modest duration to the element with the specified id value.
When an effect does something to an element while leaving it in place, the effect() function performs well (such as bouncing the element).
It is preferable to use the extensions to jQuery's hide(), show(), and toggle() methods provided by jQuery UI if you wish to hide or show an element.
Similar to the effect() method, these have the following syntax:
About all jQuery effects
jQuery UI Hide Method
To control jQuery UI visual effects, utilize the jQuery hide() method. It applies an animation effect to components that are hidden.
Syntax:
A hide method's parameters are:
- effect: This option describes the transition effects that are employed.
- options: These are used to select a particular setting and to soften the impact. There are different alternatives for every impact.
- duration: This details the length of time and the effect's millisecond duration. The default setting is 400.
- complete: This method is a callback. As soon as the effect is finished for each element, it gets called.
Let's look at a jQuery UI shake effect with the hide() method as an example.
jQuery UI show Method
To control jQuery UI visual effects, utilize the jQuery show() method. It is designed to use the indicated effect to display an item. The elements that are contained within the designated effects are given a visibility specification.
Syntax:
The parameters of this method are:
- effect: It describes the transition effects that are employed.
- options: These are used to specify the effects' ease and particular setting. There are different alternatives for every impact.
- duration: The duration parameter details the length of time and the effect's millisecond duration. The default setting is 400.
- complete: This is a callback method. As soon as the effect is finished for each element, it gets called.
To illustrate the jQuery UI show() method, let's look at an example.
jQuery UI Toggle Method
Depending on whether an element is hidden or not, the show() or hide() function is toggled using the jQuery toggle() method.
Syntax:
These are the parameters of the **toggle()** method.
- effect: This option describes the transition effects that are employed.
- options: Options are used to select a particular setting and to soften the impact. There are different alternatives for every impact.
- duration: This details the length of time and the millisecond duration of the effect. The default value is 400.
- complete: It is a callback method, to be precise. As soon as the effect is finished for each element, it is called.
Let's look at an example for implementing the toggle() method in jQuery UI:
JQuery UI addClass Method
The CSS property changes can be animated using the jQuery addClass() technique. While animating all style changes, it adds the provided classes to the matching elements.
The addClass() method of the jQuery UI has the fundamental syntax (Version 1.0) as follows:
Parameters of addClass() method:
- className: This string contains one or more classes from the CSS language. (If there are other CSS classes, a space is used to separate them.)
- duration: In milliseconds, this is used to specify the time period. The element is taken instantly to the new style with no progress when the value is 0. The default value for the parameter is 400.
- easing: It is a string type that describes how to advance the effect. Swing is its default value.
- finished: When the effect for this element is finished, a callback method is called for each element.
jQuery's addClass method syntax (Added in version 1.9):
The parameters in addClass() Version 1.9 function are as follows:
- className: This string contains one or more classes from the CSS language. (If there are other CSS classes, a space is used to separate them).
- options: To define all animation settings, use this. Each property has an optional use. Its potential values include:
- duration: This sort of number or string describes how long the effect will last. In milliseconds, it is measured. The element is taken directly into the new style when the value is 0, without any progression. The default value is 400 for this parameter.
- easing: It's a string. therefore it's easy. It outlines how to advance the impact. Swing is its default value.
- complete: When the effect is finished for this element, a callback method called complete is called for each element.
- children: The animation should be applied to all ancestors of the matching elements, according to this Boolean type, which determines whether it should. False is its default value.
- queue: This defines whether to add the animation to the effects queue and can be of the string or Boolean type. TRUE is the default value.
To illustrate the jQueryUI addClass() method (Passing a single class), let's look at an example:
jQuery UI removeClass Method
The jQuery removeClass() method is used to control the appearance of jQueryUI. The elements' applied classes are removed.
The removeClass() method animates all style changes while removing the provided classes from the matched components.
The removeClass() method of jQueryUI has the fundamental syntax listed below (Added in jQueryUI version 1.0):
The removeClass() method's parameters are:
- className: This string contains one or more classes from the CSS language. (If there are other CSS classes, a space is used to separate them.
- duration: In milliseconds, this is used to specify the time period. The element is taken instantly to the new style with no progress when the value is 0. The default value is 400.
- easing: It is a string type that describes how to advance the effect. Swing is its default value.
- callback: When the effect for this element is finished, a callback method is called for each element.
The jQuery removeClass method's syntax is as follows (Added in version 1.9):
The children option in the new jQueryUI version 1.9 additionally supports animating descendant components.
- className: This string contains one or more classes from the CSS language. (If there are other CSS classes, a space is used to separate them).
- options: To define all animation settings, use this. Each property has an optional use. Its potential values include:
- duration: This kind of number or string denotes time for which the effect will last. In milliseconds, it is measured. The element is taken directly into the new style when the value is 0, without any progression. The default value is 400.
- easing: It's a string that shows the way to progress in the effect. It outlines how to advance the impact. Its default value is "swing".
- callback: When the effect is finished for this element, a callback method called Complete is called for each element.
- children: The animation should be applied to all ancestors of the matching elements, according to this Boolean type, which determines whether it should. False is its default value.
- queue: This defines whether to add the animation to the effects queue and can be of the string or Boolean type. TRUE is the default value.
Example of jQuery UI removeclass:
jQuery UI switchClass Method
To transition from one CSS class to another CSS class while animating the change from one state to another, use the jQuery UI switchClass() method.
This method helps in switching between classes using removeClassName and addClassName with duration and ease.
The option parameters in this method are similar to the addClass and removeClass methods.
Now, let's look at an example code to see how this method works.
UI Color Animation with jQuery
To expand the usefulness of the animation features, jQuery UI adds a few methods to the base jQuery code. For an element, you can animate many transitions. Color animation is also supported by jQuery UI. One or more CSS attributes that specify an element's colours can be animated here.
The CSS properties that support the animation technique are listed below:
CSS property | Description |
---|---|
backgroundColor | It is used to change the element's background colour. |
borderTopColor | It is used to specify the colour for the element's border's top side. |
borderBottomColor | This property is used to provide the colour of the element border's bottom side. |
borderLeftColor | This property is used to provide the colour of the element's border's left side. |
borderRightColor | This property is used to specify the colour of the right-side border of an element. |
colour | It is used to change the text element's colour. |
outlineColor | It is used to specify the colour of the element's outline and to draw attention to it. |
jQuery UI's animation method syntax is as follows:
Now let's use a simple example to see how the addClass() method can be used to illustrate the animate method.
Conclusion
- In this article, we've seen how jQuery UI effects work, using the different jQuery methods and their types and use cases in detail.
- Practice more and write your own code to understand the concepts in depth.