Convert JSON String to Java Object
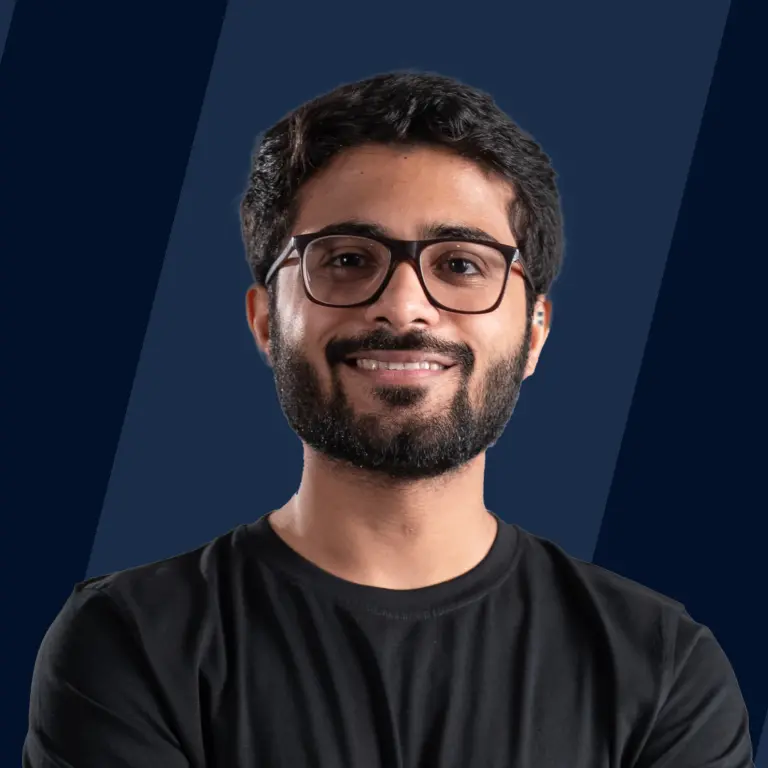
JSON format is an industry-wide standard for sharing data between web services or data applications. It is used extensively for passing information from front-end to back-end systems. When consuming JSON at back-end systems at the deserialization layer (conversion of byte stream sent over the network to an object), it becomes important to convert the JSON to a Java object. Various open-source libraries help us achieve this.
Converting JSON String to Java Object
There are some standard steps that we should follow to convert JSON to Java Object.
Creating a Java Class
To begin with, we must design a Java class mirroring the structure of the JSON object. Each field in the class should correspond to a key in the JSON object. Libraries such as Jackson, Gson, or JSON-B can automate mapping JSON objects to Java objects.
Here's an illustration showcasing a Java class reflecting a JSON object:
Choosing a JSON Parsing Library
The next step is to select a JSON parsing library for converting a JSON string into a Java object. Several widely used options in Java include Jackson, Gson, and JSON-B. Each of these libraries offers its own set of features and benefits, so your choice will depend on your project's specific requirements and preferences.
Parse the JSON String
After selecting a JSON parsing library, you'll utilize its API to transform a JSON string into a Java object. The specific steps vary depending on the chosen library.
For instance, with the Jackson Library:
In the above code, the ObjectMapper class from the Jackson library decodes the JSON string and maps it to a Person object.
Handle Exceptions
When converting a JSON string into a Java object, it's crucial to account for potential exceptions that might arise during the process. JSON parsing libraries typically raise exceptions if the JSON string is malformed or if there's an issue with mapping the JSON structure to the corresponding Java class.
Let's illustrate this with an example using the Jackson Library:
In the above code, objectMapper.readValue(jsonString, Person.class) attempts to convert the JSON string into a Person object. Suppose any issues arise during this process, such as malformed JSON or mismatched mappings. In that case, an exception is thrown and caught in the catch block, where you can handle the exception accordingly, perhaps by logging the error or taking other appropriate actions.
Best Practices and Alternative Ideas
-
We should start by validating the JSON string's structure using a JSON validator or schema. This step ensures the JSON conforms to expected patterns, reducing parsing errors later.
-
JSON-B, a standardized JSON binding library introduced in Java EE 8, is another good option. It is especially beneficial for Java EE projects. JSON-B offers a consistent and standardized approach to JSON parsing, promoting interoperability and maintainability.
-
We should opt for libraries like Jackson or Gson, which offer annotation support. Annotations enable customized mapping between JSON objects and Java classes, streamlining the parsing process while providing flexibility.
-
We should prioritize immutability for converted Java objects to maintain integrity. Immutable objects are inherently thread-safe and prevent unintended modifications post-creation, ensuring consistency and stability in the application's state.
-
JSON parsing can be resource-intensive, particularly with large strings. We should employ streaming APIs from libraries to process JSON incrementally, minimizing memory usage and enhancing performance, especially in memory-constrained environments.
Real-World Applications and Beyond
It is always essential to learn dealing with JSON objects because it processes and stores the data quickly, there are different real-world applications where JSON conversion is essential as listed below:
-
Web Development and JSON: In web development, JSON is a fundamental medium for data exchange between clients, typically web browsers and servers. This interchange necessitates transforming JSON data into Java objects on the server side to facilitate processing.
-
Data Analysis with JSON: Within the domain of data analysis, JSON emerges as a prevalent format for data interchange. Data analysts frequently encounter the need to convert JSON data into Java objects to execute diverse operations, such as filtering, sorting, and aggregation.
-
Engaging with APIs: Numerous web APIs furnish data in JSON format. When interfacing with these APIs within a Java environment, converting the retrieved JSON data into Java objects is essential. This underscores the significance of mastering JSON to Java object conversion in API development and integration.
Conclusion
- In this article, we learned how to convert JSON to Java objects.
- There are some critical steps to follow while converting the JSON to a Java object.
- We can use Jackson, Gson, and JSON-B as JSON parsing libraries.
- We can avoid JSON parsing exceptions using the standard try-catch blocks.
- There are different real-world applications where we require JSON to object conversion.