How to handle KeyError Exceptions in Python?
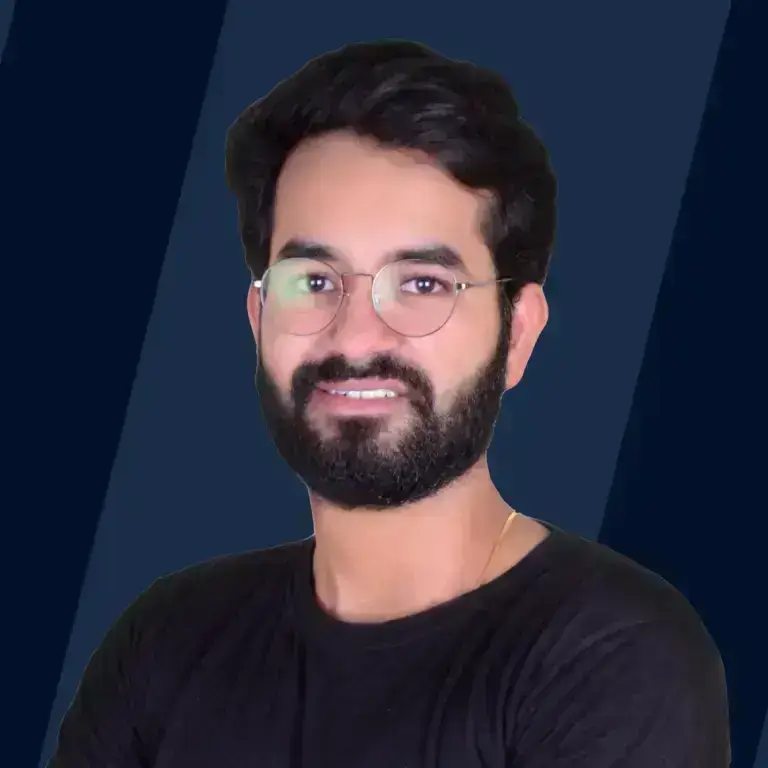
KeyError in Python occurs when one tries to access a key in a Python dictionary that is not present in the dictionary. Let's see an example where a KeyError Python gets raised while accessing a key in the Python dictionary.
Python KeyError Example
In the above-defined dictionary, you can access the vehicle model by its key model. Let's see how we can access the vehicle model by its key.
OUTPUT
Now, let's try to access a key that is not present in the above dictionary.
OUTPUT
As in the above example, we are trying to access the vehicle speed by its key speed which is not present in the above Python dictionary, KeyError Python is raised as we are trying to access the key which is unavailable in the dictionary.
KeyError in Python can occur when user input is required and a value is retrieved from the dictionary based on user input. As the user is unknown of the keys in the dictionary KeyError can occur while trying to access an unavailable key in the dictionary.
In this article, we will see how to fix KeyError in Python dictionaries.
When to Raise a Python KeyError in Your Own Code
Raising a KeyError in your own Python code can be a strategic choice to ensure your program behaves correctly and provides clear feedback under certain conditions. Here are some points on when it might be appropriate to raise a KeyError:
- Raise KeyError for missing keys in dictionaries to alert users of input issues.
- Use KeyError for input validation, especially for essential dictionary keys.
- Ensure API consistency by mimicking dictionary access behavior with KeyError.
- Detect programming errors early by raising KeyError for unintended missing keys.
- In custom classes with dictionary-like behavior, use KeyError to maintain Python's expected behavior.
How to Fix the Dictionary KeyError in Python?
There are two methods by which we can fix KeyError in Python :
- Using .get() method
- The in Keyword
- Using Try Except Block
Fix the KeyError in Python Using .get() method
To fix a KeyError Python issue, especially when trying to access a value from a dictionary with a key that might not exist, you can use the .get() method of dictionaries. This method provides a safer way to access values by returning None (or a default value you specify) if the key is not found, instead of raising a KeyError.
Syntax:
- dictionary is your dictionary object.
- key is the key you're trying to access.
- default_value is optional; if not specified, None is returned when the key is missing. This value is returned if the key isn't found in the dictionary.
Example Code:
Explanation:
- When accessing the key 'name', the .get() method returns the value associated with the key, which is 'John'.
- When attempting to access 'address', a key that does not exist in my_dict, the .get() method returns None, preventing a KeyError.
- By providing a default value, such as 'No address provided', the .get() method returns this default value instead of None when the key 'address' is not found. This approach avoids a KeyError and can provide clearer feedback in the absence of expected data.
How to Fix the KeyError in Python Using the in Keyword?
Using the in keyword you can check if the key exists in the dictionary or not. If it exists you can give the corresponding value of the key stored in the dictionary else you can give the error message to the user.
Let's take an example to understand how you can use the in keyword to resolve the KeyError Python dictionary.
Let's break down the code into smaller pieces and try to understand what is happening :
Firstly, we created a dictionary named vehicle which has three items that are model, color, and average.
Next, we take user input using the input() function and use that input as a key to get the value from the dictionary.
Then we created an if-else block to check if the key entered by the user is present in the dictionary defined at the top. If the value corresponding to the particular key is found in the dictionary then we output the value to the user else we show the message to the user to input the correct value which is present in the dictionary.
The above complete code can be run for both existing and non-existing keys in the dictionary to get the appropriate output without any KeyError.
How to Fix the KeyError in Python Using a try-except Keyword?
The try-except block is similar to the try-catch block used in other programming languages for error handling. The try block checks for any error and the except block handles the error that occurs in the try block.
Let's take an example to understand the try-except block
Just like in the previous section in this try-except block method also we are first creating a dictionary named vehicle with three keys model, color, and average.
Then we are taking user input similarly using the input() function from the user.
In the try block, we are directly giving the output if the value corresponding to the input key is present. If the value is not present then except block handles the KeyError Python and prints the appropriate message to the user to input the correct key.
Conclusion
- What is keyError in Python? KeyError in Python occurs when one tries to access a key in a Python dictionary that is not available in the dictionary.
- KeyError Python can be resolved using keyword in Python.
- KeyError Python can also be resolved using try-except block in Python.
- in keyword in Python is used to check if the key is present in the dictionary or not and accordingly show the Output.
- In try-except block try block checks for any error and except block handles the error if the error occurs in the try block.
Learn More
Learn more about KeyError Python handling and other types of error in python