C Program to Check Leap Year
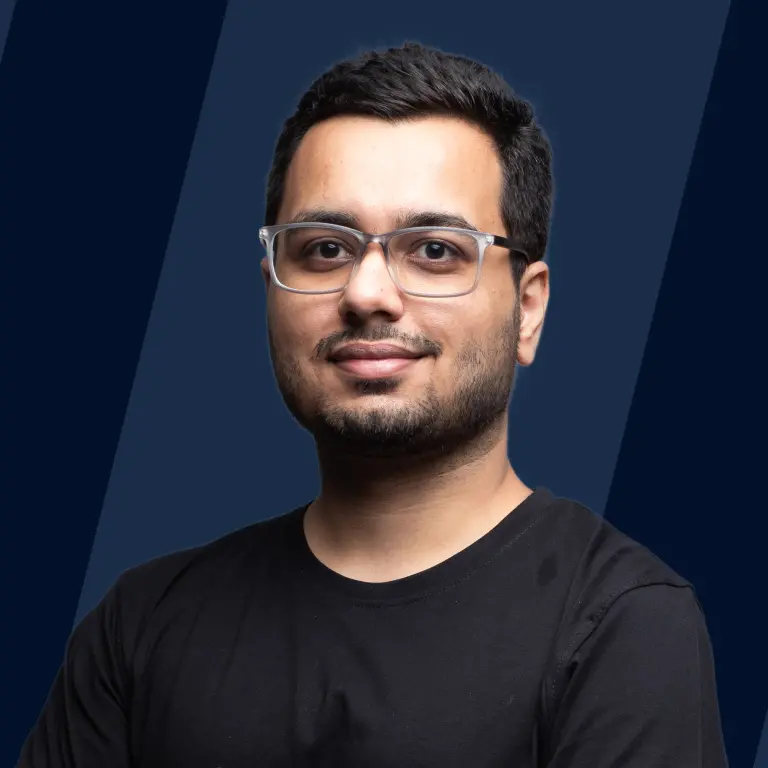
Overview
In C, leap year identification hinges on conditional checks using the modulus operator to test for divisibility criteria specific to leap years. The program utilizes logical conjunctions and disjunctions (&&, ||) to apply the rule that years divisible by 4 and not by 100, or divisible by 400, are leap years. This logic is encapsulated within an if-else block, which is the cornerstone for control flow in C programming. The resulting program is a succinct demonstration of applying arithmetic operations and control structures to solve a calendrical problem. Mastery of such conditional logic is crucial for C programmers tackling a variety of algorithmic challenges.
What is a Leap Year?
Have you heard of anyone having their birthday on 29th February and teasing them about their age?
This is what we call a leap year that has 366 days instead of 365 days. One extra day is added in February.
For example - , etc.
There are a few conditions to check whether a given year is a leap year. A year is a leap year if the following conditions match:
- If the given year is multiple of but not multiple of .
- Or the given year is multiple of .
Not every four years is a leap year. As a rule, leap years are skipped if the year is divisible by and not by .
Algorithm
To check a given year, you must see if the above conditions are satisfied. The leap year in C can be explained as:
- Suppose you have a year in a variable.
- Check if the year is divisible by 4 but not by 100 using if-else statements and logical operator and.
- Also check if the variable is divisible by 400 using the logical operator or.
- If either of the two conditions is satisfied, then print that the given year is a leap year. Otherwise not.
Flow diagram
The Leap Year program in C can be explained using the flowchart.
Pseudocode
Following is the pseudo-code of the leap year program in C:
Explanation:
- The given year must be valid.
- If the given year is divisible by then the first "if" statement executes.
- Otherwise, the next else-if condition is checked, and if the given year is divisible by , then it is not a leap year.
- If the above else-if statement doesn't execute, then the year is not divisible by and goes to the next. The next else-if checks if it is divisible by . If yes, then the given year is a leap year.
- If none of the above is true, then the else part executes.
Implementation
The code below is an implementation of the above algorithm for leap year in C.
Output
If you put year=1999, then the output will be:
Output
How to Check if a given Year is a Valid Year?
You can check the data type of the input and compare whether it is an integer or not.
Explanation
The above code can be explained using the example . You can see that is divisible by but not by . Hence, it is a leap year. But, is neither divisible by nor by . Hence, it is not a leap year.
C Program To Check Leap Year
#include <stdio.h>
int main() { int year;
// Prompt the user to enter a year
printf("Enter a year: ");
scanf("%d", &year);
// Check if the year is a leap year
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
printf("%d is a leap year.\n", year);
} else {
printf("%d is not a leap year.\n", year);
}
return 0;
}
Examples
Example 1: Check A Leap Year By Taking Input From User
The program provided in the previous section allows the user to input a year, and then it checks and displays whether that year is a leap year. The example below demonstrates how this works:
Usage Example:
Example 2: Find The Leap Years Between The Range Of Two Years
The following program finds and displays all the leap years in a given range, such as between 2000 and 2020:
Usage Example:
In this program, the user is asked to enter the start and end years. The program then iterates over each year in the range, checks if it is a leap year, and prints it if it is.
Conclusion
- A leap year has days unlike normal years having days.
- It comes every year.
- Leap year program in C can be written by keeping certain conditions in mind. A leap year is always divisible by and not by , or it must be divisible by .
- Adding an extra day every four years aligns our calendar correctly with the astronomical seasons.