C++ Program to Check Leap Year
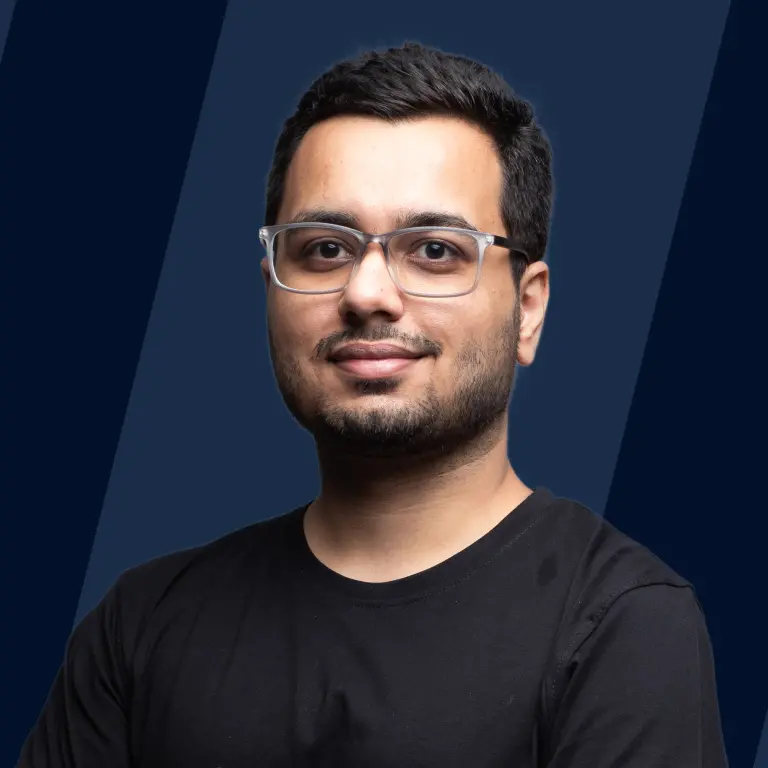
Overview
A leap year is a calendar year with an extra day than normal years, i.e., it consists of 366 days. The general idea behind identifying if a given year is a leap year is to check if the given year is divisible by both 4 and 400. If this is the case, then the given year is a leap year. Otherwise, it is checked if the given year is divisible by 4 but not by 100 or if the given year is not divisible by both 4 and 100 but it is divisible by 400. The given year is not a leap year in these two cases.
Introduction
A leap year is a calendar year with an extra day than a usual year (, i.e., it has 366 days instead of 365 days). The leap year occurs every four years; the extra day is added to February.
The intuition for identifying if a year is a leap year:
- If the given year is divisible by 4 and 400, then the given year is a leap year.
- If the given year is divisible by 4 but not by 100, then the given year is not a leap year.
- If the given year is not divisible by both 4 and 100 but divisible by 400, then the given year is not a leap year.
Prerequisites
Before we get into the writing program to identify if the given year is a leap year, let us learn about some prerequisites.
C++ if, if...else and Nested if...else
The if, if...else, and nested if...else statements, also known as conditional statements, are a method for the program to make certain decisions based on the provided conditions.
C++ if Statements:
An if statement in C++ is a conditional statement that executes the code or task within its block only if the provided statement is true.
Syntax:
pictorial representation:
C++ if...else Statement:
An if...else statement in C++ is an extension of if statements. It is a conditional statement that executes the code or task within its block when the condition provided in the if statement is false.
Syntax:
pictorial representation:
C++ Nested if...else
An if...else statement in C++ is an extension of if-else statements. In nested if else another if statement or if else statement is nested within an if or else statement.
Syntax:
C++ Operators
Operators in C++ are defined as the symbol used to perform certain operations on given variables or values.
There are mainly five types of operators in C++:
- Arithmetic operator
- Relational operator
- Logical operator
- Bitwise Operator
- Assignment Operator
What is a Leap Year?
As discussed in the above section, A leap year is a calendar year with an extra day than a usual year (also known as a tropical year).
This is because a normal year (or a tropical year) consists of 365.24219 no. of days precisely. But in the calendar, a tropical year is only shown 365 days. The value 4*0.24219, whose value is 0.96876, which roughly rounds up to 1, gets added at the end of every fourth year hence making the count of the days 366.
Leap Year Program in C++
Using if -else ladder
In this section, we will write a leap year program in C++ using the if-else ladder that will take a year value as an input and print if the given year is a leap year or not.
Input:
Output:
Explanation of the example:
In the above example, the checkLeapyear function takes an integer as an input and first checks the condition n % 400 == 0; if the expression is true, then the program will display that the 'given year is leap year', otherwise it checks the conditions (n % 100 != 0) and (n % 4 == 0). If both the conditions are true, the program will also display that the 'given year is a leap year'; otherwise, it will display that the 'given year is not a leap year'.
Using nested if
In this section, we will write a leap year program in C++ using the nested if-else ladder that will take a year value as an input and print if the given year is a leap year or not.
Input:
Output:
Explanation of the example:
In the above example, the checkLeapyear function takes an integer as input and first checks the condition (n % 4 == 0); if it returns true, then it checks the condition (n % 100 != 0); if it returns true then it checks the condition (n % 400 == 0).
- If (n % 400 == 0) is false, the program will display that 'the given year is not leap'.
- If (n % 100 != 0) is false, the program will display 'the given year is leap'.
- If (n%4==0) is false, the program will display that 'the given year is not leap'.
Program to Check Leap Year Using Logical Operators in C++
In this section, we will write a leap year program in C++ using ternary operators that will take a year value as an input and print if the given year is a leap year.
Input:
Output:
Explanation of the example:
In the above example, the checkLeapyear function takes an integer as an input and then checks the condition ((n % 4 == 0 && n % 100 !=0 ) || (n % 400 == 0)) using ternary operator.
The expression ((n % 4 == 0 && n % 100 != 0) || (n % 400 == 0))** is evaluated, and if either (n % 4 == 0 && n % 100 != 0) or (n % 400 == 0) is valid for the given input then the result is stored in boolean value isValid as true, otherwise it is stored as false. If the value of isValid is true, then the program will display that the 'given year is leap year'; otherwise, it will display that the 'given year is not a leap year'.
Conclusion
- A leap year is a calendar year with an extra day than normal years (i.e., 366 days instead of 365).
- The leap year in C++ is identified with the use of conditional statements or with the use of operators.
- If a given year is divisible by both 4 and 400 then it is a leap year.
- If the given year is divisible by 4 but by 100 then the given year is not a leap year.
- If the given year is not divisible by both 4 and 100 but it is divisible by 400 then the given year is not a leap year.