Left Shift Operator in C++
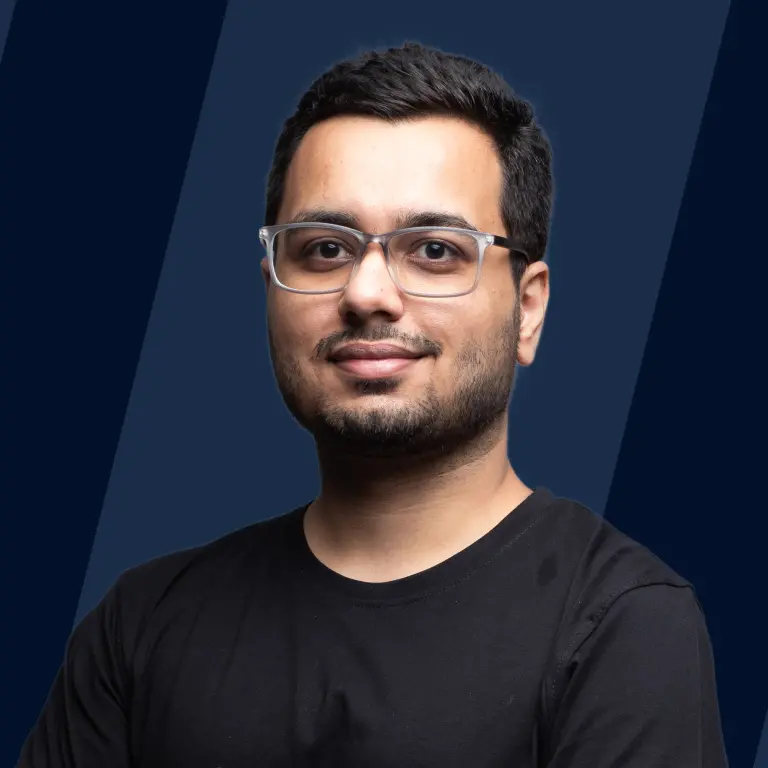
Overview
The left shift operator << is essential in C++ programming. It's a handy tool that allows you to transfer the bits of a number to the left, much like changing beads on an abacus. When you use this operator, you multiply the integer by 2, raised to the power of the shift amount. For example, if you have the number 5 (101 in binary), shifting it left by two places results in 20 (10100 in binary). This operator is valid for encoding, decoding, and optimizing algorithms.
Left Shift(<<) Operator in C++
In C++, the left shift operator (<<) is a powerful tool for bit manipulation, operating at the binary level. It works by shifting the bits of the left operand to the left by the specified number of places and filling the newly opened positions with zeros. This operator requires two operands: the value to be shifted and the number of moving positions.
Commonly used for optimizing multiplication or division by powers of two, the left shift operation accelerates processes like multiplication by 2, 4, 8, etc. However, excessive shifting can lead to unintended consequences or overflow issues. Understanding its binary nature is essential for effectively harnessing the operator's benefits while avoiding pitfalls. Careful implementation ensures the left shift operator becomes a valuable shortcut without introducing unforeseen complications.
Syntax
The Left Shift Operator (<<) in C++ is used to shift a given value's bits to the left by a specified number of places. The syntax is easy to understand:
In this case, value is the original number whose bits are being shifted to the left, numPositions is the number of places the bits should be pushed to the left, and result is the value after the left shift operation.
For example, if you take the number 5 (binary: 0101) and move it left by two points, you get 20. (binary: 10100).
Example
In C++, the Left Shift Operator (<<) is a powerful bit manipulation tool. It is used to shift a number's bits to the left by a given number of places. This procedure multiplies the number by two multiplied by the shift count. For example, if you have the value 5 (binary 101) and move it left by two locations, you will receive '20' (binary 10100). The syntax in C++ is as follows:
Output:
Important Points to Remember
Here are key considerations when working with the left shift operator:
- Initial Syntax: The left shift operator is structured as operand numPositions. The operand represents the value to shift, and numPositions indicates the count of positions to shift.
- Bit Multiplication: Left shifting is akin to multiplying the operand by two raised to numPositions. This facilitates quick integer multiplication by powers of two, a valuable technique for optimizing code and calculations.
- Boundary Checks: Care is needed when shifting beyond the bit capacity of a data type. Going a 32-bit integer left by 35 places can yield unexpected outcomes. Ensure shifts align with data type limits.
- Data Loss: Left shifts may lead to data loss if shifted bits exceed data type boundaries. Bits falling outside the data type are discarded.
- Practical Applications: Left shifts find wide use in generating bit masks, optimizing code, and packing multiple values into a single integer. For instance, creating flags or options using individual bits within an integer.
- Avoid Negative Shifts: Negative shifts are nonsensical and often result in undefined behaviour. Always use non-negative integers for shifts.
- Combining with Other Operators: Left shifts can be combined with operators like bitwise OR or AND for advanced tasks such as setting or clearing specific bits.
Conclusion
- The Left Shift Operator is a critical component of C++ programming that allows you to modify individual bits within integers in an efficient manner.
- The primary function of the left shift operator is to shift the bits of a given integer to the left by a defined number of places. The integer is multiplied by two raised to the power of the shift count in this operation.
- The left shift operator is a quick and easy technique to accomplish multiplication by powers of two. The left shift operator is often used for bit mask creation and low-level bitwise operations.
- Left-shifting a signed integer may result in unexpected behaviour if the result exceeds the range that the data type can represent.