LinkedHashMap in Java
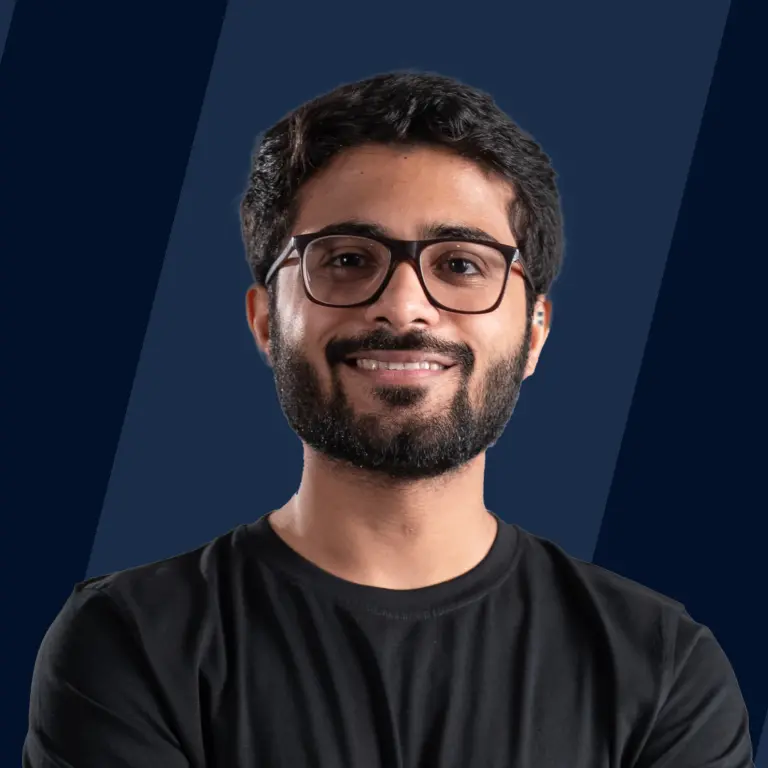
LinkedHashMap in Java is similar to that of HashMap, with an additional feature that maintains the order of elements inserted. it will be slower than HashMap for adding and removing elements but you can expect faster iteration with a LinkedHashMap.
The main advantage of using LinkedHashMap is that it maintains and tracks the order of insertion where elements can be inserted and accessed in their order.
Important Features
- It contains values based on keys and implements the map interface by extending the HashMap class.
- It only contains unique elements
- It might have one key, which is null, and multiple null values
- It is non-synchronized
- It is the same as HashMap but with a difference in maintaining the insertion order. For instance, when a code containing HashMap is executed, different orders of elements are shown in the output.
Declaration
Parameters
Here, K is the key type of object, and V is the value of the Object
- K – The type of the keys in the map.
- V – The type of values mapped in the map
The Map<K, V> interface is implemented, and HashMap
class is extended. The hierarchy of LinkedHashMap is shown below:
Constructors of Java LinkedHashMap Class
For creating a LinkedHashMap in Java, an object must be created to access the class. It contains various constructors that make it possible to create the array list. The following are the constructors available:
- LinkedHashMap(): It is used to construct the default constructor.
- LinkedHashMap(int capacity): Used for initializing a LinkedHashMap with a specified capacity.
- LinkedHashMap(Map<? extends K,? extends V> map): Used for initializing a LinkedHashMap with specified map elements.
- LinkedHashMap(int capacity, float fillRatio, boolean Order): Used to initialize both the capacity and the fellatio along with the insertion order.
Methods of LinkedHashMap
Method | Description |
---|---|
contains value(Object value) | If the map maps one or more keys to a specified value, it returns true. |
entrySet() | Returns a view that is set and contains mapping |
get(Object key) | It returns a value to which a key specified is mapped, returns null if the map contains no mapping key |
keySet() | Returns a view that is set and contains mapping |
removeEldestEntry(Map.Entry<K,V> eldest) | It returns true if map removes entry which is eldest |
values() | It returns a collection view of all the values contained in the map |
Example of LinkedHashMap in Java
Output:
Various Operations on Java LinkedHashMap Class
Operation 1: Inserting Elements to Linkedhashmap
To insert elements in Linkedhashmap, we use put() method.
Output:
Explanation:
The LinkedHashMap is created, and elements are inserted using the function put(). This function allows us to add elements in the LinkedHashMap as per the given order.
Operation 2: Changing/Updating Elements in Linkedhashmap
Output:
Explanation:
The LinkedHashMap is created, and elements are inserted using the function put(). This function allows us to add elements in the LinkedHashMap as per the given order. The method replace is used to update the previous value with the new value.
Operation 3: Removing Element from Linkedhashmap
Output:
Explanation:
In above example, The LinkedHashMap is created, and elements are inserted using the function put(). This function allows us to add elements in the LinkedHashMap as per the given order. The remove method discards the element from LinkedHashMap completely.
Operation 4: Iterating through the LinkedHashMap
Output:
Explanation: In the above example, the LinkedHashMap in Java is created, and elements are inserted using the function put(). This function allows us to add elements in the LinkedHashMap as per the given order. In this example, it's string and int.
Conclusion
- The LinkedHashMap in Java maintains insertion order (or, optionally, access order).
- LinkedHashMap in Java is not synchronized by default. If thread safety is required, it can be achieved by explicitly synchronizing the map using Collections.synchronizedMap() or ConcurrentHashMap.
- LinkedHashMap consumes slightly more memory than HashMap due to the additional overhead of maintaining the linked list.
- The performance of operations like insertion, deletion, and retrieval is slightly lower than HashMap due to the extra overhead of maintaining the linked list for preserving order.