List Input In Python
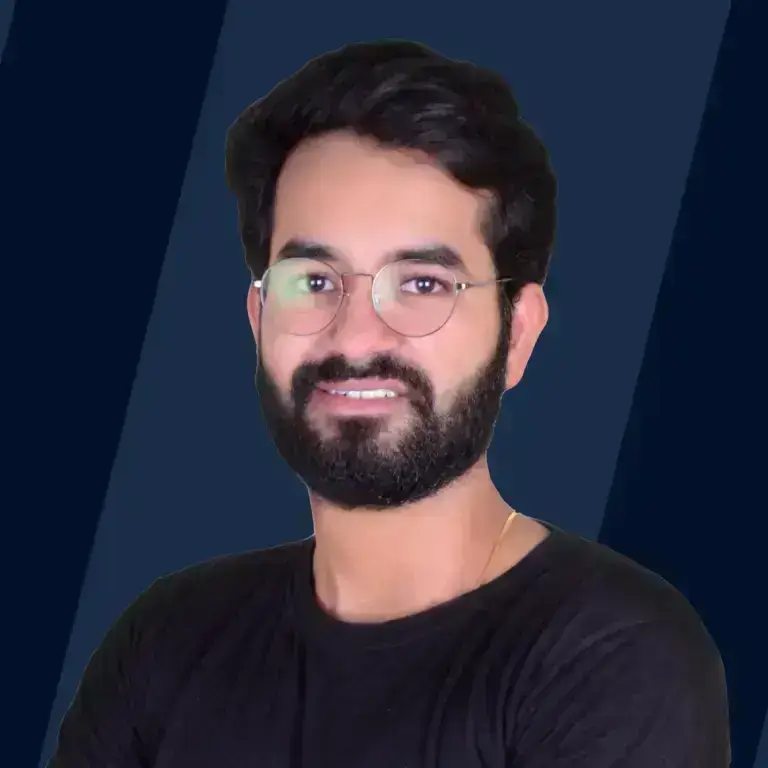
Overview
Lists are the dynamically sized arrays (similar to the vector of C++ and the ArrayList of Java). Taking list input in python is not as simple as taking input of an integer, floating-point number. It requires some set of statements to accept the user input in the right way.
How to Input a List in Python?
We often encounter scenarios, when we want to take input of multiple integers/floats/strings from the user, and storing all of them using distinct variable names is not a fair idea. In these situations, we use one of the built-in python data types i.e list.
Lists in python are used to store multiple data items (possibly of different types) under a single variable.
Generally, to take the user input in python we use the input() function, but when we take list input in python the input() function is used in a slightly different manner.
Input a List using Loops
When you Take the Number of Elements to be Filled in List Initially
If we know the number of elements to be taken as input from the user we can follow the below-given points to take the input from the user -
- Firstly, take the input of the number of elements in the list and store the input value in a variable n.
- Then, declare an empty list using the following syntax list = [].
- Iterate from 0 to n-1 using a for loop and do the following -
- Take the input of an integer from the user, and store the input value in a variable x.
- Append x to the list using the following syntax list.append(x). Here appending x means inserting x next to the rightmost position of the list.
Code
Output
Without Taking Number of Elements as Input
If we do not know the number of elements provided as the input for the list, we can accept the input as a string and split them using the built-in split() function, which by default splits the string provided by blank white space. Let's see how this works in python -
Output -
From the above output, we can say that when we use the split() function to take the list input in python, the elements are of string type. Also, if we want to split our input using ',' or any other thing, we can pass that as an argument of the split function. For example split(",") or split("_")
If we want only a certain type of element in the list we can use exception handling. Let's say we want integer type elements in our list, so we will use a loop by using a loop till we do not encounter any non-integer type input.
So, we will use try and except statements in our code, if you are not aware of these terms then we would strongly suggest you read this article to get detailed insights about the exception handling in python.
Code
Output -
Input a List Using the Map Function
We can take list input in python using the map() function in Python also. The idea is to use the map() function to wrap all the user-entered numbers in it, and then convert them into the desired data type.
Below are the steps used to take list input in python -
- First, take input of the size of the list.
- Now, take the input of all the elements as a string.
- Then, use the map() function to wrap each element present in the string and convert them to desired data type (say float).
Code -
Output -
Note that [:n] in the above code means elements first n all the elements.
Input a List Using List Comprehension & Typecasting
List input in python using a list comprehension is similar to taking the list input using the map function. When taking input using the map() function we were using the map to convert the input to the integers, but here we will simply use the int() function instead of the map() function. Note that it is not limited to int(), we can use other data types also.
We can take the input of a list in python by following the below-given steps -
- First, take input of the size of the list.
- Now, take the input of all the elements as a string.
- Then, use the map() function to wrap each element present in the string and convert them to the desired data type (say int).
Code -
Output -
List of Lists as Input
To take input of a list of lists (nested list) in python, we need to follow the below-given steps -
- Find the number of lists to be taken as input, let it be n.
- Declare an empty list, let it be list.
- Iterate for n times and do the following -
- Declare an empty list, let it be tempList.
- Take input and store it in tempList using any of the methods described in previous sections.
- Append tempList to list.
Let's see how we can implement this -
Output -
Conclusion
- Lists in python can accommodate different data types in them i.e they can contain heterogeneous values.
- List input in python can be taken in various ways such as using loops, split() function, map() function, etc.
- Exception handling can be used to take only the desired data type in the list.
- Taking the input of a list of lists is also simple, which can be easily done by adding one additional for loop.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.