List Iterator in Java
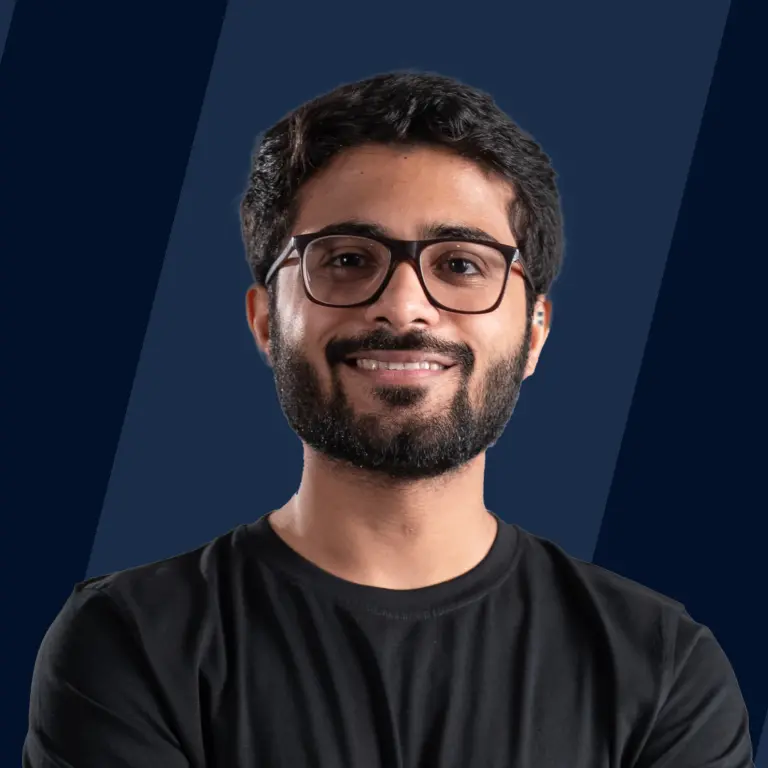
Overview
List Iterator in java was introduced first in Java 1.2. It is used for list traversal in both forward and backward directions. ListIterator is one of the four supported java cursors. The other cursors in Java are Enumerator, Iterator, and SplitIterator.
A list iterator is used to iterate over list objects and the list iterator object is created by using the ListIterator() method.
What is a List Iterator in Java?
List iterator is an interface that is a member of the Java Collections framework. It extends the iterator interface and provides the functionality of accessing all the objects of a list. It is used to iterate over a list of implemented objects one by one. Unlike other iterators, it supports CRUD operations and is bidirectional.
Note :
Create,Read, Update and Delete operations are collectively known as CRUD operations.
The list iterator in java does not point to any current element in the list. Its cursor will always lie between the previous and the next element where the previous() function will return the previous element and the next() will give the next element. It has n+1 cursor positions for n elements in the list.
How to Use List Iterator in Java?
ListIterator in java is used to iterate over the list elements. Since it extends the Iterator interface in java, it is implemented as follows :
Syntax :
Here E signifies the generic type.
Usage :
Let us see how ListIterator works using a linked list.
Output :
Here, the elements of a list are traversed using the next() method through the listiterator.
Methods of List Iterator in Java
List Iterator has methods that allow performing CRUD operations on the list. Let us discuss those methods with examples.
1. E next() :
This function returns the next element present in the list and also advances the cursor position.
This method is used to repeatedly access the next elements of the list. It does not return any value.
Usage :
Output :
The function would return the next element.
2. void add(E e) :
This function is used to add or insert an element into the list. The element e would be inserted immediately before the element that would be returned by the next() function. This method does not have a return type.
Let us have a look at the functionality with an example.
Output :
The add() method of list iterator inserted the element before the next() element. The next() was pointing at the starting location and it would return 10 as output if called. Since the add() method adds immediately before the next() pointing element, therefore, a new element got inserted in the beginning.
3. void remove()
This method removes the element from the list that was returned last by the previous() or next() function. Two things are to be kept in mind for using this method.
- The remove() call can be made once concerning one call made for the previous and next element.
- This method cannot be called if add() method is not called after the last previous() or next() method.
Following is the example :
Output :
By calling the next() method, the cursor points at the 0th index. The remove() operation will remove the element in this cursor. Therefore, "Paul" will be removed from the list.
4. void set(E e)
This method is used to replace the last element returned by the previous or the next call by the specified element. This function can only be called if neither add() nor remove() function is called after the last previous() or next() call.
It takes an element as an input parameter and does not have any return type. Let us understand the working of the function with an example.
Example :
Output :
The function replaces the name "Alex" with "None".
Bi-directional Methods of Listiterator in Java
Unlike iterators, list iterators in java are bidirectional. These are used to access elements of the list in both forward and backward directions.
Let us look at both the iteration one by one.
1. Forward Direction Iteration
This iteration refers to moving and accessing the elements of the list in the forward direction.
The methods used in forwarding direction iteration are :
- hasNext() :
The return type of the function is boolean. It returns true if the list has elements present in the forward direction. - next():
This function does not have any return type. It returns the next element present in the list. - next index() :
The return type of the function is an integer. This function is used to return the next index after a subsequent call to the next().
Let us understand the use of these three methods in the forward iteration of the list.
Example :
Output :
2. Backward Direction Iteration
This refers to moving and accessing the elements of a list in the backward direction.
The methods used in the backward direction iteration are as follows :
- hasPrevious() :
The return type of this function is boolean. It returns true if there is an element present in the backward direction call in the list. - previous() :
The return type of this function is E i.e. generic type, It returns the previous element from the list. - previous index() :
The return type of this function is an integer. It returns the previous index that would be returned on the previous() call.
Let us understand the usage of these functions in the list iterator.
Example :
Output :
Differences between Iterator and ListIterator
Though both iterator and listIterator are used for list iterations, there are a few differences between them.
Following are the points of dissimilarities between the two.
Iterator | ListIterator |
---|---|
It is used to traverse any type of collection | It is used to traverse only list collections. |
It supports traversal in only a forward direction. | It supports traversal in both forward and backward directions. |
Iterator object is created by calling the iterator() method. | ListIterator object is created by calling ListIterator() method. |
Read and delete operations are supported. | CRUD operations are supported. |
Replacement and modification of elements are not supported. | Replacement and modification are allowed in the case of ListIterators. |
Index of the traversed element cannot be accessed. | Both the previous and next indexes can be accessed. |
Advantages of ListIterator in Java
- CRUD operations like Create, Read, Update and Delete are supported in ListIterator.
- The list of iterators is bidirectional.
- List iterator methods return the index of the previous and next elements in the list.
Limitations of ListIterator in Java
- List Iterator traverse only lists but not other collections.
- It does not allow parallel iteration of elements.
- It is not a universal cursor.
Conclusion
After a detailed analysis of Java list iterators, it can be summarized as follows.
- List Iterators were introduced in Java 1.2 and are one of the cursors supported in Java.
- It has some methods like hasNext(),set(E e),add(E e), etc to allow list operations.
- It is bidirectional and supports CRUD operations.
- List Iterator works only for lists.
This article is a complete guide on List Iterators in java. for example, Running the code snippets and making use of List Iterators while coding will enhance one's knowledge of the topic.