How to Convert a List to Dictionary in Python?
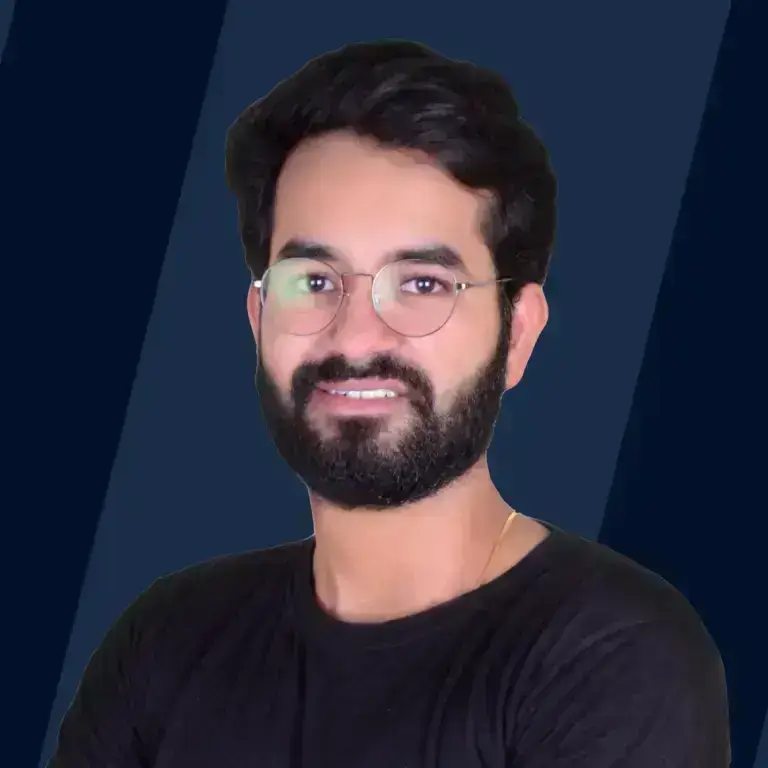
Abstract
List and Dictionary in Python, which help us to store the data, perform operations, and analyze the data as well. In List, the data is stored in the form of an array, whereas in a dictionary, the data is stored in the form of a key-value pairs. When we want to convert our list into dictionary, we need a list which can be convertable into dictionary, like a list can be a list of tuple, in which we can identify the key-value pair, or list can be list of dictionary, so that we can convert the list into single dictionary.
If the list don't show any key-value pair, then there must be atleast 2 list, so that we can tell this must be in key-value pair, for conversion of dictionary. In that case, we can use Counter(), zip(), and enumerate() method, for our conversion.
Introduction to List to Dictionary in Python
As per the title, List to Dictionary, one can think of that here we will convert our list into dictionary using Python. But moving further, lets look at some use cases for this conversions:
-
Let us consider we have a list that contains various nouns, pronouns, adjective and other english grammer words, and we need something like, we need to count the frequency of each word appearing in the list, so here we convert our list into dictionary. We will discuss the same question in the later section.
-
Next case, most of us know about ASCII Code, if don't, have a look at this link. Now, we are having a list of alphabets, and a second list that contains its ascii values, and we need to make a pair wise mapping for the same. So, here we will create a dictionary from both the list, one of the list is the key, and other is the value.
In this article, we will convert our python list to python dictionary, one might think, list stored data in the form of array, but in dictionary, we store data in the form of key-values pair. In this conversion method from list to dictionary, we will define the list as in the key-value pair.
Code:
We need to make a program, like this it will convert our list into dictionary. As previously mentioned there are several techniques/methods to do so. Let's start it. Now moving forward we will see first Method Using Dictionary Comprehension.
Examples of List and Dictionary
List examples :
Input:
This example, show the list of alphabets, and displaying them.
This example, show the list of english grammer letters, and displaying them.
This example, show the list of tuples, that contains alphabets and its ascii codes, and later displaying thmem.
In this example, we have created a list of dictionary, which contains alphabets as their keys, and its ascii code as their values.
Output:
Code:
Dictionary Examples:
Input:
In this example of dictionary, we have created a dictionary of ascii codes, which have alphabets have dictionary keys, and ascii codes are dictionary values.
In this example, we have created a dictionary of a candidate.
This example, contains, a number and its square value.
Output:
Code:
Method 1 : Dictionary Comprehension
Dictionary Comprehension is a very powerful method that is used for tranformation one dictionary to another dictionary. During this method, while transforming one dictionary into another, we can conditionally included the data or exclude the data into the new dictionary.
Write a program to create a dictionary that contains number as its key, and square of that number as its value.
Explanation:
- Line 1 : It will read the number 'n'.
- Line 2 : using dictionary comprehension method, we are creating a dictionary that contains, number as its keys, and square of a number as its value.
- Line 3 : It is printing the dictionary.
Output:
Code:
Now, lets come to our main task i.e., to convert our list into dictionary.
So, in this we will define our list
Now, lets define our dictionary comprehension, for our conversion, but before that what we can see is, in list first 2 elements are number and its square and so on. So, this case is similar to previous one.
Output:
Code:
Now, we have created our dictionary by using comprehension, the statement states that loop from 0th index to nth index, with take the gap of 2, i.e., (0,2,4,...,n), and make the pair of number and its square.
Method 2: zip() function
zip() function, this function is used to create a zip(which is an iterator of tuples where the first item in each passed iterator is paired together, and then the second item in each passed iterator are paired together) object. It takes the parameter an iterator object(list, tuple or any other iterable object). If the iterators are of different lengths, the iterator of small length, will decide the length of the object.
Syntax: zip(iterator1, iterator2, iterator3, ...)
Parameters: iterator1, iterator2, iterator3, ... : Iterator objects that will be joined together
It will return a zip object, which can later be type cast into dictionary or any other data type.
Output:
Code:
Method 3 - dict.fromkeys()
dict.fromkeys(), is a method that returns a new dictionary with the key mapped and its specific value. It will take input as an sequence(list), and a value which help us to mapped with the sequence, and will return a dictionary with the values as 'None', if no value is assigned to it.
Syntax: dict.fromkeys(seq, val)
seq: The sequence that is to be transformed into dictionary
val: The value to be specified as its values in the dictionary.
By using this method, we will create a sequence of the list, to which we want to convert into dictionary, and for the value, we will also create list, which will match the sequence.
Steps to follow to convert list to dictionary in python:
-
Create a sequence(list) that contains the data, in our case it will states of a country
-
For our conversion from list to dictionary while using dict.fromkeys(), we need a value for mapping for this, we have assign the value to it.
-
Call the dict.fromkeys() method, and pass list and the value pass as the parameter to it.
-
Last, print our new dictionary.
Output:
Code:
In this method, we have a created a sequence of states in which the states of India are mentioned, and we want to assign the country "India" as their value, so we have use this method to create a dictionary using list, list in this case is our "state".
Method 4 - Using Tuples
In this method of conversion from list to dictionary in python we will be using tuple,
in this case, we will create the list of tuple, in that each tuple contains (key, value) in its tuple, and later on will convert it into dictionary by using type casting method that will convert our list of tuples into a single dictionary.
This is simplest among all the methods that we have studied till now.
Output:
Code:
Method 5 - Converting a List of Alternative Key, Value Items to a Dictionary
This method is bit complicated, and confusing too. In this method, first we will create a list of alternative key-value pair, and later we will match first key to third value, second key with forth value, and so-on. Or you can consider them as we will match all even-even index elements and all odd-odd index elements.
Steps:
- Create a list in which alternative key-values are present.
- Lets perform some slicing in the list to perform our task.
- Next, we will perform our previous technique that we learned is dictionary comprehension.
Output:
Code:
Method 6 - Converting a list of Dictionaries to a Single Dictionary
In this method of conversion of list to dictionary is, we have a list of dictionaries, and we have to combine all those dictionaries present in the list, and make a single dictionary. Now lets have a look of input and output list and dictionaries.
Input:
Output:
In the above example we have seen that in the list of ascii codes, we have a seperate dictionary, or we can say we have a list of dictionary, so we need to make a single dictionary from the given list.
Steps:
- Create the list(ascii_code)
- Using dictionary comprehension method, we will convert it to a single dictionary.
Output:
Code:
Method 7 - Converting a List Into a Dictionary Using enumerate()
When dealing with iterators, we often need to keep a count the number of iterations, that has been done. So, here comes in the picture one of the built-in function in Python enumerate(). Enumerate() method adds a counter to our iterator, and returns a enumerator object. This enumerate() can be directly used in loops to convert our list to dictionary by using dict method.
Syntax: enumerate(iterable, start=0)
Parameters:
Iterable: any object that supports iteration, like list, dict
Start: the index value from which the counter is to be started, by default it is 0.
Input:
Output:
Using this method, we can convert a list to dictionary. First of all, we need a list, list can of any data type, because in this list data type doesn't matter because we only using enumerate method to keep a counter to our list for the conversion of list to dictionary.
Steps:
- Create a list of any data type of any data mentioned in it, we have created a list of capital alphabets.
- Now, we have created a list of alphabets, and now we have to call enumerate method, and pass our list of alphabets to it, and as already mentioned in steps, 2nd parameters we have to pass is "start", so we can pass "1", as the second parameter to it.
- After creating a enumerate object, we should convert our enumerate object into dictionary, for conversion of list to dictionary. This can be done by using typecasting, and we can convert our enumerate object into dictionary.
- At last, we can print the dictionary, and we can see that input we have pass list and output we got is dictionary, and we have done our list to dictionary conversion.
Output:
Code:
Method 8 - Converting a List to a Dictionary Using Counter()
Counter() class is a special type of object that is provided in the collections module in Python. Collections module provides the user with specialized container datatypes(an alternative of built-in datatypes in Python like list, tuple, dict). It is sub-class for counting hashable objects. It will result as items of list as the dictionary keys, and the frequency of each element is the value of the dictionary key.
In this method of conversion from list to dictionary, we will using Counter() method that is available in collections module of Python. Now, lets take a look at steps to perform while doing this task, and later we will focus on code part:
Steps:
- Create a list of english grammer wordsm and init it with a variable(It is not compulsory to use english grammer words, we can use any other data).
- After creating our list, next is to import our Counter() class from collections module.
- Now, its time to call, Counter() class. After calling the Counter(), we have to some our defined list in step 1 as its parameter, and it will return a counter object, and after seeing the result of counter class, we can see that it is a kind of dictionary that is available in collections module.
- After receiving our counter object, we just have typecast our object into dictionary class, so that we can get our dictionary. Now, we converted our list to dictionary.
Output:
Code:
Summary of The Article
- In this article, we have seen brief introducton on list and dictionary in python. See some examples in it. The main focus in this article is to how to convert the list into dictionary
- First, we define some examples of list and dictionaries.
- Next, we have seen 8 methods for conversion of list to dictionary. First we have dictionary comprehension methdod, which is easy and most used by programmers for conversion, as it save time of writing codes.
- Later, we can see some built-in methods that are available in Python like zip(), enumerate() and dict.fromkeys().
- At last, we encounter with collections module for conversion of list to dictionary. From this module, we have use Counter() method to perform our task. Counter class is a special type of object provided in collections module. collections module provides us an alternative of built-in datatype in python.