Loop Through an Object in JavaScript – How to Iterate Over an Object in JS?
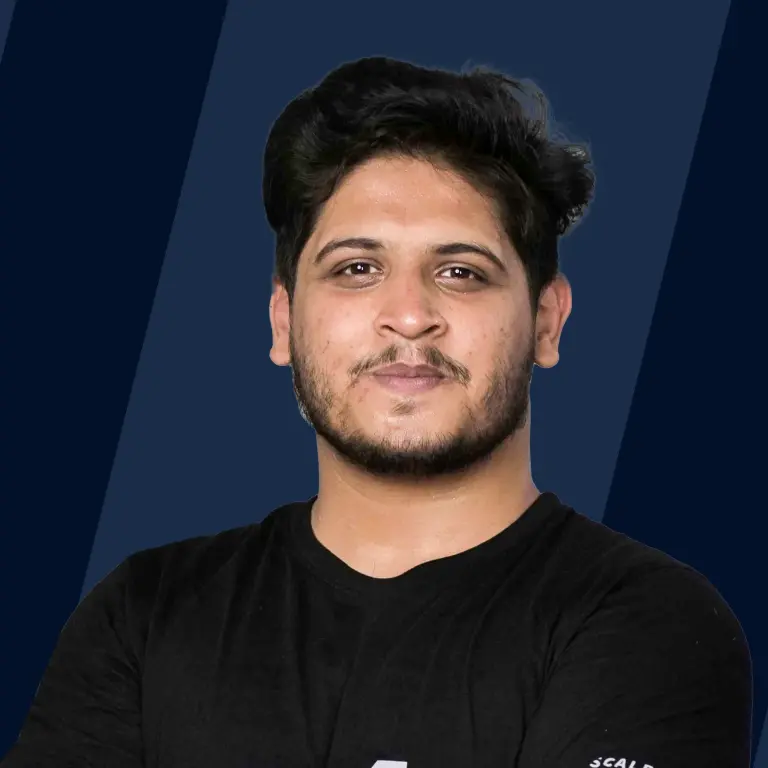
This article will teach us how to loop through object javascript. if you want to run the same code multiple times, each time with a different value then it is easy to use loops. So, we’ll see a lot of methods to loop through object javascript.
What is an Object in JavaScript?
In JavaScript, an object is a data type that allows you to store key-value pairs. It's a collection of properties where each property has a name (key) and a corresponding value. Objects are one of the fundamental building blocks of JavaScript, and they are used extensively to represent complex data structures.
Example
In this example, person is an object with four properties: firstName, lastName, age, and email.
Looping Through an Object
In JavaScript, iterating through objects empowers developers to interact with each key-value pair. This traversal is facilitated by diverse methods tailored to specific requirements. By navigating arrays generated via Object.keys(), Object.values(), or Object.entries(), developers efficiently iterate over object javascript. Some Common Methods we will discuss are:
- Using a for...in loop
- Object.keys() Method
- Object.values() Method
- Object.entries()
- Using Lodash and _forOwn() method
How to loop through an object in JavaScript with a for…in loop
- The for...in the statement is the easiest way to loop through an object's properties.
- The for...in statement pair iterates (loops) across an object's properties.
Syntax:
Parameters:
Parameter | Description |
---|---|
iterator | Required. A variable to iterate over the properties. |
object | Required. The object to be iterated |
Example
Let's take a code to understand the concept of for...in the loop to loop through the object in javascript:
Output:
How to loop through an object in JavaScript with object static methods
1. Object.keys() and forEach() method
The Object.keys() method returns an array whose elements are strings that directly correspond to the enumerable properties found on an object. Object.keys() accepts an object as a parameter and produces an array of strings that represent all of the enumerable properties of the given object.
Syntax:
Parameter:
key: It is the object whose enumerable properties are to be returned.
Return:
Object.keys() returns an array of strings representing the object's enumerable properties.
Example
Let's take a code to understand the concept of the object.keys() method:
Output:
2. Object.values()
- Object.values()method creates an array with the values of all the properties in an object.
- Object.values() method returns an array whose elements are the enumerable property values found on the object.
- When a loop is performed to the properties, the ordering of the properties is the same as that specified by the object manually.
- Object.values() accepts as an argument the object whose enumerable own property values should be returned and it returns an array containing all of the object's enumerable property values.
Syntax:
Parameters:
key: It is the object from which the enumerable property values will be returned.
Return:
Object.values() returns an array containing all of the object's enumerable property values.
Example
Let’s take a code to understand the concept of the object.values() method:
Output:
3. Object.entries() and map()
- The Object.entries() method returns an array of the object's enumerable property [key, value] pairs that are passed as a parameter.
- An array of arrays is created by calling Object.entries(). There are two items in each inner array. The property is the first item, and the value is the second.
- The properties are ordered in the same way that they would be if you looped through the object's property values manually.
Syntax:
Parameters:
key: It is the object to which the enumerable property [key, value] pairs should be returned.
Return:
Object.entries() returns an array containing the object's enumerable property [key, value] pairs.
Example
Let’s take a code to understand the concept of the object.entries() method:
Output:
4. Using Lodash and _forOwn() method
The Lodash method _.forOwn() iterates over an object's keys, invoking a function for each property. This function receives three arguments: the property value, key, and object. Early termination of iteration is possible by explicitly returning false from the iterate function.
Syntax:
Parameters:
- object: This is the object to find in.
- iteratee_function: the function that is invoked per iteration.
Example
Output:
In the above program, the output reflects each key-value pair in the car object being printed to the console, with the key followed by an equals sign (=) and then the corresponding value.
Conclusion
- for...in loop is the simplest way to loop through object javascript.
- hasOwnProperty() method is used to see if the object has the specified property as its own.
- Object.keys() method is used to create an array containing an object's properties.
- The Object.values() method creates an array containing the values of every property in an object.
- Object.entries() method creates an array of arrays, where the inner array has two items. The first item is the property, and the second is the value.