Math.floor() in Java
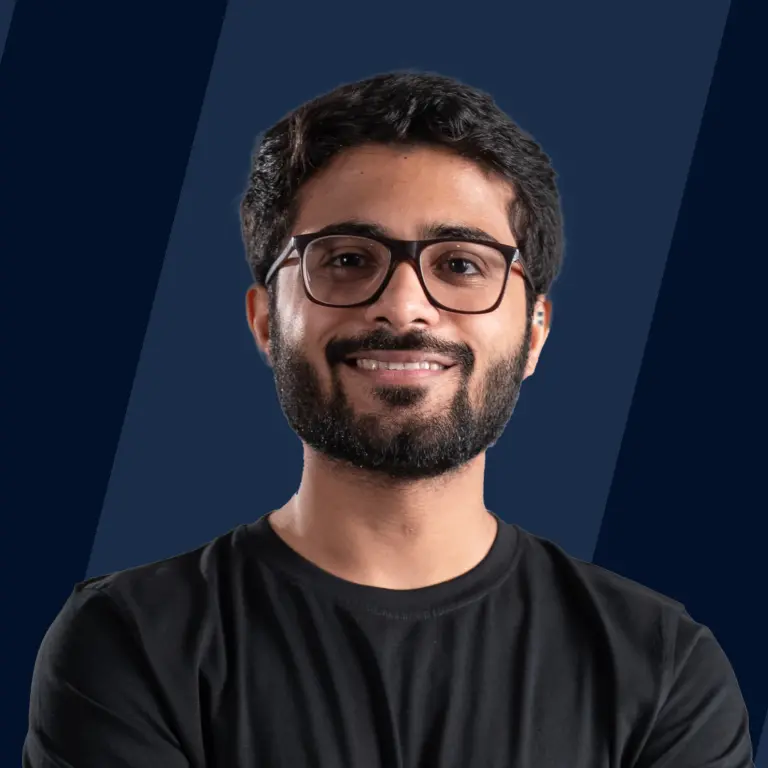
The java.lang.Math.floor() is a mathematical function in Java. This function returns a double value that is less than or equal to the passed argument and is equal to the nearest mathematical integer.
Syntax of Math floor() in Java
Syntax of Math floor() function in Java is:
A double value is passed to the Math floor() function.
Parameters of Math floor() in Java
The Math floor() function in Java has a single parameter, and that is a double value. A double value is a number with a decimal place. It is a 64-bit floating data type.
Return Values of Math floor() in Java
The return value of the Math floor() function in Java is a double value equal to the integer value immediately smaller than the floating number passed to it.
Critical Arguments
- This function returns "Infinity" if a double value divided by 0 is passed to it.
- If the argument is an integer, Infinity, NaN, positive or negative zero, the result is same as the argument.
Exception of floor() in Java
The floor() method generally doesn't throw an exception until an invalid data type is passed as an argument such as when a String is passed as an argument to floor() function, it throws compile time error as a String cannot be converted to a double value.
Example for Math floor() in Java
Code
Output
In the above example, we are removing the decimal places of the given number a = 3.8, using the Math floor() function. The result is a double value (3.0) in the output.
What is Math floor() function in Java?
The Java Math floor() is a mathematical function available in Java Math Library. This function returns the closest integer value (represented as a double value) which is less than or equal to the given double value.
For example, if we pass 5.67 to the Math floor() function, we will get 5.0 as the output.
More examples
Example 1: Math.floor() in Java
Code
Output
In the above example, we are rounding off the double value a=5.987654 to its immediate smaller integer (as a double value) 5.0 using the Java Math floor() function.
Example 2: Using Java Math floor() function with Array
Code
Output
In the above example, we are iterating over the elements of the array myArray, then we are using the Math floor() function to round off the element in an array (double values) to their immediate smaller integer as a double value.
Example 3: Using Java Math.floor() function with Arraylist
Code
Output
Explanation:
- In the above example, we are importing java.util.ArrayList to use the ArrayList data structure.
- Then we are using the ArrayList.add() function to add values to the array list.
- We are then iterating over the arrayList and using Math floor() function to round off the double values in an array to their immediate smaller integer (as a double value).
Conclusion
- The java.lang.Math.floor() function returns a double value equal to the nearest integer value, which is less than or equal to the double value passed to it as an argument.
- This function returns "Infinity" if a double value divided by 0 is passed to it.
- If the argument is an integer, Infinity, NaN, positive or negative zero, the result is same as the argument.
- Using the Math floor() function, we can find the floor values of all the numeric values inside an array or an ArrayList.