Math.sqrt() in Java
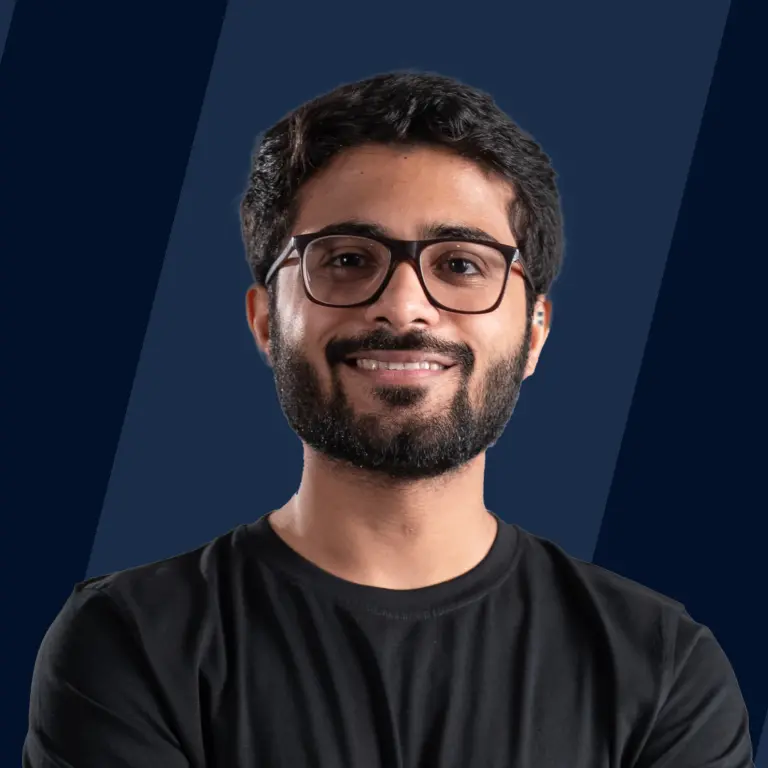
Math.sqrt() method of java.lang package is used to find a given number's correctly rounded and positive square root in Java.
This method takes double as an argument and returns a double-type variable. There are various special cases where it returns particular values as per the input. Also, we can implement our own square root method using a mathematical formula and using the Math.pow() method.
We can also find the square root of BigInteger, whose range is -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807, with the help of the respective class's inbuilt sqrt()` method.
Introduction
The Math.sqrt() in Java is present in java.lang.Math class and is used to calculate the square root of the given number. It returns the double value that is correctly rounded to the positive square root of a given double value. In the case of negative number input, it returns NaN(Not a Number) as the square root of a negative number is not real but an imaginary number. Since it is a static method, we can call it using its class name, such as Math.sqrt().
Method Declaration of Math.sqrt() Method in Java
Math.sqrt() method in Java is declared as follows:
It is a static method, i.e., it can be directly called using the Math class.
Syntax of Math.sqrt() in Java
To find the square root of a number we use the following syntax, pass the number num to sqrt() method. Also, num can be of the type int,float, or double. Lastly, we can store the result in another variable of Double type.
Parameters of Math.sqrt() in Java
Math.sqrt() method takes a single variable of double data type whose square root we are supposed to find.
Return Values of Math.sqrt() in Java
The Math.sqrt() method returns a double value that is the square root of the given value and a correctly rounded positive number. If the argument is NaN or a negative number, it returns NaN, i.e. Not a Number.
Examples of Math.sqrt() Method in Java
Let us see an example of finding the square root of the user's entered number.
Output:
Explanation:
We store the user-entered value into the num variable of double type, which is passed to the Math.sqrt() method. The Math.sqrt() method returns a double value stored in the squareRoot variable and printed.
Example: Special Cases
There are various special cases where we might expect a different value, such as the square root of infinity or a negative number.
Note : NaN stands for Not a Number in Java. It is a special floating-point value that denotes overflows and errors. It is generated in cases where a floating-point number is divided by zero or the square root of a negative number is computed as the square root of negative numbers, resulting in imaginary numbers.
Let us consider these five special cases:
- Zero(0) - It returns 0.0 (zero as a double value) as a square root of 0.
- Negative Integer - It returns NaN as the square root of the negative number is a complex number.
- Positive Infinity - It returns Infinity.
- Negative Infinity - As it is infinity but still a negative number, it returns NaN.
- NaN - Square root of NaN is returned as NaN itself.
Let us verify the outputs using a Java program:
Output:
As we can see in the above example, it returns NaN for NaN or negative numbers or negative Infinity as arguments. It returns 0.0 for 0 as an argument and Infinity for Double.POSITIVE_INFINITY as an argument.
Working of Math.sqrt() in Java
The square root of a number in Java is calculated using simple arithmetic operations such as addition, subtraction, multiplication, and division. Lastly, we get the desired answer with the help of loops. The mathematical formula used to find the square root precisely is given below.
Algorithm for Finding Square Root of a Number in Java
We first take two variables, num and half. num is left uninitialized, and half is initialized as value/2. We run a do-while loop; inside the do-while loop, we initialized num=half each time the loop runs and half = (num + value/num), where the value is a user-entered number. We run this loop until num is not equal to half. As this condition satisfies, half is the required square root of value.
Let us apply it to the Java code.
Output:
Square root using pow() method
There are other ways to find the square root of a number in Java except for the Math.sqrt() method. The first one we saw in the previous example was when we implemented our method that calculates the square root of a number. Another way is using the Math.pow() method, located in the same package as Math.sqrt(), i.e. java.lang.
The square root is nothing but a number to the power 1/2 (0.5 or half). We can pass 0.5 or 1/2 as the power to Math.pow() to get the square root of a number.
Let us see this example to compare Math.pow() and Math.sqrt() methods in Java.
Output:
Explanation:
In the example, we first ask for user input, which we later pass to both methods, Math.sqrt and Math.pow(), with power being 0.5. We can see that both methods return the same answer.
BigInteger sqrt() Method
BigInteger in Java is used where big integer calculations are required that are outside the range of integer or long data types. The java.math.BigInteger.sqrt() method returns a BigInteger that is the square root of the given BigInteger. It is just the same as floor(sqrt(num)), i.e., it returns a floor value unlike Math.sqrt(), which returns a double(numbers after decimal).
Syntax
The syntax of sqrt in Java of BigInteger is slightly different from that of Math.sqrt(). It takes no parameter, can only be called using objects of the BigInteger class, and returns the floor value of the square root of the respective BigInteger.
The below example illustrates the sqrt() method of the BigInteger class.
Output:
Explanation:
- The num variable is of the BigInteger type, which has 19 digits. The value of the num variable cannot be stored in an Integer or Long type variable.
- num.sqrt() returns a BigInteger that is the square root of num and is stored in squareRoot.
- Again, the square root of the given number is big enough that it cannot be stored in int data type.
Conclusion
- Math.sqrt() in Java is a method that uses precision to find the square root of any number of double types.
- It is present in java.lang.Math class.
- The sqrt() method in Java takes a double type variable and also returns a positive double value, which is the square root of the argument and is correctly rounded.
- It returns NaN when the argument is Negative or NaN. It returns 0.0 and infinity for arguments 0 and positive infinity, respectively.
- Java provides two other ways to calculate the square root of a number, one of which is using the Math.pow() method with power being 0.5. We can also implement our own method for finding the square root using simple arithmetic operations and loops.
- We can also find the square root of a BigInteger. The java.math.BigInteger class contains the sqrt() method, which returns the floor value of the BigInteger type, which is the square root of the given BigInteger.