Max Function in Java
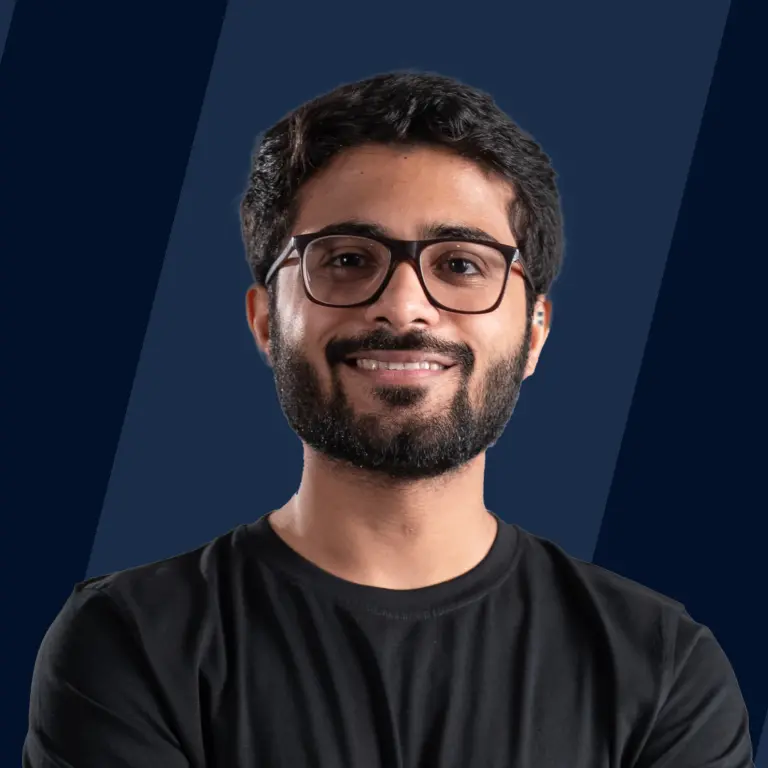
Overview
The max() method is an inbuilt method of Math class which is present in java.lang package that is used to find the maximum of two numbers. The max() method takes two inputs that are of types numbers, i.e., int, long, float, double, and returns the maximum of the given numbers.
The max() method does not throw any exception. However, both the arguments in the Math.max() method must of same type, otherwise compile-time error is thrown.
Syntax of Math.max()
The Math.max() method takes two parameters that can of int, long, float, and double data types. However, both the inputs must be of same type, otherwise compile-time error is thrown.
Parameters of Math.max()
The max() method takes two parameters of any valid datatypes. For example, Math.max(int a, int b) is the valid syntax, whereas Math.max(String a, int b) is not a valid syntax because the max() is valid for the numeric datatypes only.
Valid Syntax of the Math.max() method
- Math.max(int a, int b)
- Math.max(long a, long b)
- Math.max(float a, float b)
- Math.max(double a, double b)
The above Syntax is the valid syntax of the max() method.
Return type of Math.max()
The return type of the Math.max() is determined by the type of the parameters. If the parameters are of int, long, float, and double type, the return type of max() will be int, long, float, and double respectively.
Exceptions of Math.max()
The Math.max() method does not throw any exceptions.
What is max() Method?
Let's suppose you have two numbers, and you want to find which number is the maximum. How can you find the maximum of two real numbers? The first answer is you compare both real numbers using comparison operators like greater-than (>) or less-than (<) operators and find the maximum number.
The second method is to take the help of the inbuilt max() method of Math class in Java that takes two numbers as input and returns the maximum of two numbers. This one is easy compared to the first method. Let's understand the max() in Java with the help of examples.
Examples
We will discuss few examples to understand max() method in Java.
Example 1: Java Math.max()
The simple example finds maximum value between two integers.
Output:
We get 45 because the maximum of 4 and 45 is 45.
Example 2: Get a maximum value from an array
Let's understand how to get a maximum value from an array using Math.max() method.
In the below example, we are iterating on the elements of the given array. Using the Math.max() method, we are finding the maximum value and updating the max value in the 'maxValue' variable.
In the starting, we initialized the maxValue variable to Integer.MIN_VALUE because Integer.MIN_VALUE is the smallest value that can be present in an integer array.
Output:
Since, 4 is the maximum value present in the array, it gets printed.
Example 3: Find the maximum of the two double numbers
Let's understand how to find the maximum of the two double numbers passed by the user using an example.
In the below example, We are initializing two double variables with appropriate double datatype values, and we are finding the max of two double values using the Math.max() method. The return type of this method is double because both parameters are of double datatype.
Output:
The 3.5 is the maximum among 2.0 and 3.5 values and hence, Math.max() method picks that value.
Example 4: Find the maximum of the two integer numbers accepted by the user
Let's understand how to find the maximum of the two integer numbers accepted by the user from the user using an example.
In the below example, We are taking two input values using the Scanner class, finding the max of two values similarly as we did in the above case.
Output:
The two inputs given to the program are 1 and 2, and using the Math.max() method, We get the maximum value that is2 here.
Finding Maximum of Three Numbers using max()
We have three numbers, and we have to find which number is the maximum number among all three. How can we find the maximum number using the max() method?
The answer is we have to use the nested max() method, i.e., max() method inside another max() method, because max() method can take only two numbers; that's why to find the maximum of three numbers, we have to use nested max() method.
Let's understand how to use nested max using an example.
Output:
The answer is 11 because 11 is the maximum number.
Example where Error is thrown
Example 1:
Error:
error: no suitable method found for max(int,String)
int max = Math.max(a, c);
^
method Math.max(int,int) is not applicable
(argument mismatch; String cannot be converted to int)
method Math.max(long,long) is not applicable
(argument mismatch; String cannot be converted to long)
method Math.max(float,float) is not applicable
(argument mismatch; String cannot be converted to float)
method Math.max(double,double) is not applicable
(argument mismatch; String cannot be converted to double)
1 error
Explanation:
We get a mismatch exception because we passed a String parameter in the max() method that is invalid.
Example 2:
Error:
Conclusion
- The Math.max() method is the inbuilt method of the Math library.
- The Math.max() method takes two parameters as an argument and returns the maximum of two.
- The return type of the Math.max() method depends upon the type of the arguments.
- Math.max() does not throw any exception. However, if the inputs are invalid or do not match the required syntax, compile-time error is thrown.
- We can use nested Math.max() to find the maximum of three numbers.