Menu Driven Program in Java
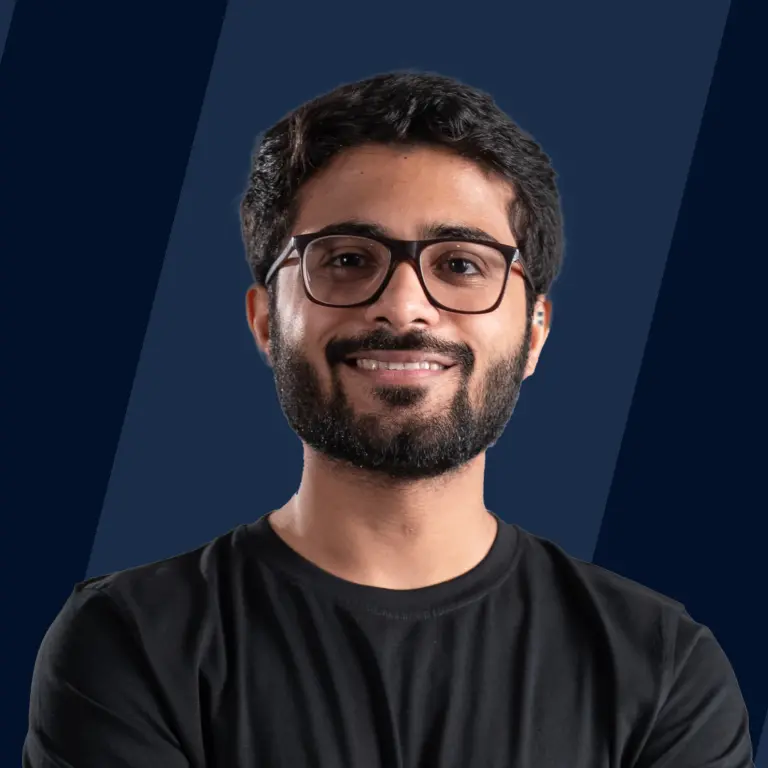
Overview
Java's menu-driven programs are programs that display a menu and then ask the user to select an option from the menu. Depending on the user's selection, further processes are done. Programs of this kind interact with users a lot and thus are user-friendly.
Introduction To Menu Driven Program In Java
A menu-driven program takes user input by presenting a list of options, known as the menu, from which the user can select one. Typical systems that process Menu-Driven programs include washing machines with microprocessors and automated teller machines (ATMs).
A menu-driven system has two advantages: First, input is done with just one keystroke, thereby reducing the chances of a system that is too prone to error. The second point is that Menu-Driven Systems limit the characters that can be entered, thus rendering the input unambiguous. Because of these two characteristics, the interface is intuitive and easy to use.
How do you Write an Algorithm for a Menu-driven Program
The algorithm for a menu-driven program is shown below. Do have a look at it
Menu Driven Program In Java Examples
Let us look at various implementations of menu-driven programming by having an example of each
Menu Driven Program in Java for Calculator
In this example, we will develop a menu-driven program for calculating 4 essential math functions: addition, subtraction, multiplication, and division. Let us look at an example
Example:
Explanation:
A switch case is used to select an operation. Using math formulas, we perform addition, subtraction, multiplication, and division. This first case involves adding up two numbers. In the second case, we calculate the difference between two numbers. The third case involves finding the product of two numbers. The fourth case involves the division of two numbers. The fifth case involves ending the program.
Flow Diagram:
Output:
Menu Driven Program in Java for String Operations
This is a menu-driven Java string computation program. It compares, trims, concatenates, and computes the length of two strings. Users can determine which operation they want to execute by pressing the appropriate number. Let us look at an example
Example:
Explanation:
String operations are performed according to the user's choice based on the strings inputted. Our system displays a menu and reads the choice selected by the user. The first case involves trimming the strings. The second case involves comparing two strings. In the third case, we determine how long a string is. In the fourth case, we concatenate strings. By using the break statement in the fifth case block, we exit the switch-case block, and we demand the user enter a valid choice
Output:
Menu Driven Program in Java using While Loop
Let's look at how to use a while loop to create a menu-driven program in Java.
Example:
Explanation:
The above program uses a Java while loop whose condition statement evaluates to true. The loop displays the menu and reads the user's choice. We display the menu and read the choice selected by the user in the loop. String computation is done based on the string provided (which is also entered by the user) as per the user's choice. The first case involves trimming the strings. The second case involves the comparison of two strings. The third case involves computing the length of the string. The fourth case performs string concatenation. We then use the break statement in the fifth case block to exit from the switch-case block, and we insist the user enter a valid choice.
Output:
Menu Driven Program in Java to Compute Areas of Different Geometrical Shapes
This is a menu-driven Java area calculator program. It is used to find the areas of different geometric shapes. Users are free to select whichever operation they wish to perform Let us look at an example
Example:
Explanation:
Based on the dimensions of geometric shapes (which are also entered by the user), we calculate the area of the shapes based on the user's choice. Because our while loop ends once the user enters the number 7, we label the loop, which forces the loop to end once the loop condition evaluates to true. Using the break statement in the seventh case block, we can exit both the switch-case block and the while loop by using the loop label.
Output:
Menu Driven Program in Java Using Functions
In this program when the user selects a particular option, the program will call a particular function within the switch case.
Let me illustrate my point by calculating the areas of a square, a circle, and a rectangle. The Square's area will be computed when the user selects it first. The area of the circle will be evaluated if the user makes a choice second. In case the user chooses a third, then the rectangle's area will be evaluated
Example:
Explanation:
A menu is presented inside the main method and the user is asked to select one. When option one has clicked the method to calculate the area of the rectangle will be called. If the user selects 2, then the method to calculate the area of the square will be called. If the user selects 3, then the method to calculate the area of the circle will be called and If the user selects 4, then the program will quit, and if the user selects an invalid choice, then the default case will be called. Separate methods are created to calculate the area of a rectangle, square, and circle, which will be called when a user makes his/her proper selection.
Output:
Menu Driven Program In Java Using do-while Loop
It is generally mandatory to execute the body of a menu loop at least once in a menu-driven program.
For example,
Here,
- We execute the body of the loop first, then evaluate the Expression.
- If the expression evaluates to true, we execute the do statement again.
- We evaluate Expression again.
- If the Expression evaluates to true, we run the do statement again.
- If the Expression evaluates to false, we repeat the process. After this, the loop ends.
For creating a menu-driven program in this case, the do-while loop is very helpful. Let us look at an example
Explanation:
To choose an operation, we use a switch case. We then use math formulas for addition, subtraction, multiplication, and division. When dividing, we keep in mind that if the numerator is zero then it should print an error. In the first case, we are calculating the sum of two numbers. The second case is for finding the difference between the two numbers. The third case is for finding the product of two numbers. The fourth case is for computing the division of two numbers. In the fifth case, we are asking to end the program. The do-while loop will repeatedly ask the user whether he wants to continue.
Output:
How to Exit from a Menu-Driven Program
You can use the predefined method System.exit(int) in order to exit a menu-driven program, which you can invoke by putting a case in the switch case. Through the exit() method of the System, one can terminate the current Java virtual machine running on the system. It takes a status code as its argument.
Conclusion
- A menu-driven application provides users with a list of options. Through the menu displayed, a user can pick and choose options according to his or her preferences and do whatever they wish.
- With menu-driven systems, inputs are made through single keystrokes, so user error is less likely
- A menu-driven program can only accept a limited number of characters so the methods of entering data become unambiguous. This makes the system easier for users to use.