Modulus Operator in C
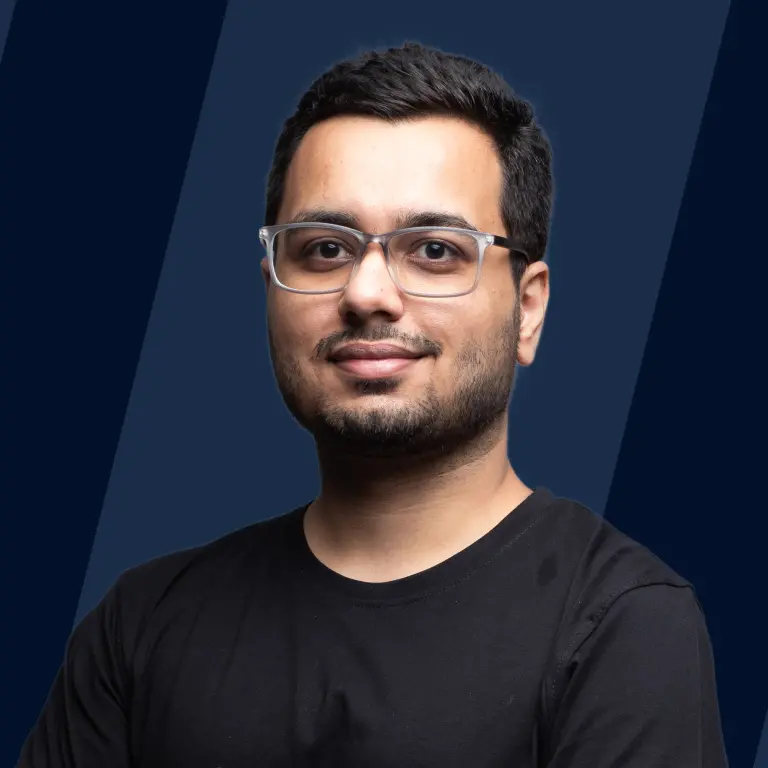
Introduction
Modulus operator, also called as modulo operator, is a binary arithmatic operator in C language, denoted using % (percentile) symbol Binary means two, that means, it is operated with two operands, both being integers only
Modulo operator is majorly used to get the remainder when two integers are divided.
For Example:
If on division no remainder is there, then it returns 0 as the remainder
Syntax of Modulus Operator in C
As we have seen above, modulo operator is only applicable with integer operands, so if we have two integers a and b, then we can apply modulo operator as -
Return Values for Modulus Operator in C
When we are doing a % b (read as a percentile b or a modulo b), the result can vary depending upon the values we are having in a and b
Let's have a look at that.
Possible return values of a % b
- If a and b are non-integer values, then it will result in an error as invalid operands to binary %
- if a is not perfectly divisible by b then it will return the remainder of a divided by b ie, some non-zero value
- If a is completely divisible by b, then it will return 0
- If a is a number and b is 0, then it will result in a warning as division by zero and the program will exit abruptly.
Example
Let's now look at some examples and their outputs to understand the concept better.
You may think how the result is 4 in the second printf() statement, let's understand this by breaking it down using simple division law 4 % 5 should give the remainder, when 4 is divided by 5, but as 4 is smaller than 5, so it will take 0 as the possible quotient, and hence will break as -
This is why we will have 4 as the remainder in the second case
How Does Modulus Operator Work in C?
As we know modulus is a binary operator so it works on two opearands, more specifically two integer operands So when we apply the modulus on two integers, it divides the first integer by the second integer and return the remainder obtained
For Example:
- 5 % 4 will return 1, as when we divide 5 by 4, we get 1 as the quotient and 1 as the remainder
- 8 % 5 will return 3, as when we divide 8 by 5, we get 1 as quotient and 3 as remainder
- 12 % 6 will return 0, as 12 is completely divisible by 6, and hence the remainder will be 0
- 7.4 % 4 will result in an error as modulo operator only works on integer operands
- 5 % 0 will result in an warning of division by 0 and the program will terminate
Note - A common mistake made by beginners is, sometimes, when they read the code, they mistakenly think of modulus as quotient instead of the remainder. Always remember the modulus operator returns the remainder of two integers.
Calculation of Modulus Operator in C
We know that modulus returns the remainder of two integer operands, but have you ever wondered how the compiler processes it internally, how the operation works?
Let's have a look at that. Whenever we write a % b it will be broken down by the compiler as a - (a / b) * b ie, saying "a % b" is equivalent to saying "a - (a / b) * b"
For Example:
- Lets have a = 5 and b = 4, then
- for a = 12 and b = 5
this how the internal instructions of modulus operator works
Examples to Implement Modulus Operator in C
Now let's see various examples and cases where we will be implementing the modulus operator.
Example 1 - Remainder for Integer Numbers
Input:
Output:
Example 2 - Remainder with Float Numbers
Output:
As we have discussed above, that modulus operator works between only the integer operands
Example 3 - Remainder for Numerator Float and Denominator Int
Output:
The modulus operator needs both the operands as integers only, even if one is a non-int value, then also it will throw an error
Example 4 - Remainder for Numerator Int and Denominator Float
Output:
The modulus operator needs both the operands as integers only, even if one is a non-int value, then also it will throw an error
Example 5 - Remainder with Zero Denominators
Input:
Output:
Some Methods of How to Use the Modulus Operator in C
The modulus operator is not only limited to simply calculating the remainder of divisions, but we can also use that to do some more complex tasks
For Example:
Use of % Modulo Operator to Implement Leap Year Checking Function in C
Input:
Output:
Use of % Modulo Operator to Generate Random Numbers in the Given Integer Range in C
Input:
Output:
Some Exceptional Restrictions of the Modulo Operator in C
Along with all the usage of the modulus operator we have seen till now, it has some restrictions as well
- It can not be used with Floating Point numbers Modulus operator works only on integer operands, if we try to operate it with float or double datatype, it will result in a compile time error (as we have seen above)
The below program will result in an error
-
While working with negative operands, the sign of result of modulus operator is machine dependent. ie, when we apply the modulus operator on negative integers, then the sign or result being positive or negative depends on the machine on which the code is running. It is because the sign of the result is dependent on the result of overflow or underflow of the specific machine it is running on.
On some compilers, it will result in a positive numbers, and on some, it will be a negative number.
Chaining of Modulus Operator
We can chain the modulus operator to execute some complex instructions and to calculate the remainder of multiple operands at once.
For Example:
Quick Explanation - Here the result is computed as per the order of precedence ie
Learn more
- To learn more about Operators in C, visit here
Conclusion
- Modulus is a binary arithmetic operator in C language, denoted using % symbol
- Syntax : a % b , it gives the remainder of, when a is divided by b
- It is only appliable to integer operands; using it with float or double will give compile time error
- Internally it breaks down as a % b = a - (a / b) * b
- Apart from finding normal remainders, we can use it in various other things like- checking if a year is a leap year, generating random numbers in a given range etc.
- We can chain the modulus operator to perform some complex calculations as well Learn more about Arithmetic Operators in C