Multiple Inheritance in Java Using Interface
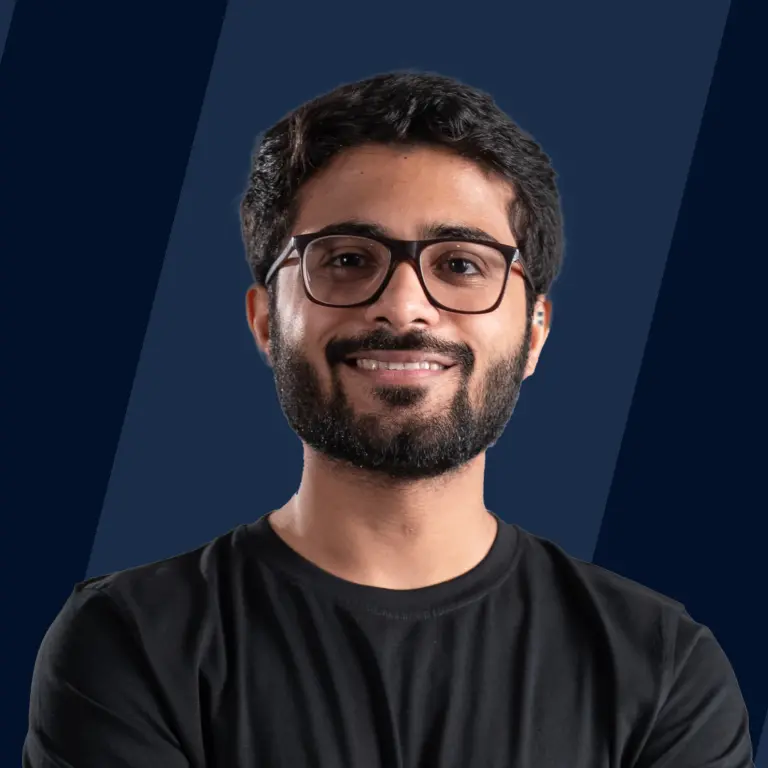
Overview
Interface is the blueprint of a class that provides a way to achieve abstraction in Java. It includes a set of abstract methods that specifies the optional capability you would want your class to implement. Interfaces are used to achieve multiple inheritance in Java.
Multiple Inheritance in Java Using Interface
Java is an object-oriented programming language that supports inheritance functionality. Inheritance is a process using which one class acquires the properties and behavior of another class.
One special case of inheritance is known as multiple inheritance. Multiple inheritance is a special type of inheritance in which a class can inherit properties of more than one parent class. It allows a class to have more than one superclass as shown in the figure given below:
Here, the subclass is inheriting the properties and behaviors from two parent classes, namely SuperClass1 and SuperClass2. This type of inheritance is known as multiple inheritance. For some reason, multiple inheritance of classes is not supported in the Java programming language. Let's look at an example and try to find out why Java doesn't support multiple inheritance of classes.
Why Multiple Inheritance is Not Supported Through Class in Java?
Consider a scenario where we have to model three variants of cars namely CNG, Petrol, and Hybrid cars. To model these real-world entities into object-oriented software, we need four classes: Car, CNG_Car, Petrol_Car, and Hybrid_Car. These classes can be coupled together via inheritance as shown in the figure given below:
In the above diagram, we can notice that the four classes are forming a diamond as the CNG and petrol cars inherit the properties of a car, whereas the Hybrid car inherits the properties of both the CNG as well as Petrol car. This formation of diamond in the application structure increases the complexity and make rise to a compile-time ambiguity.
Ambiguity: The Hybrid class extends both the CNG as well as Petrol Car classes. Both of the parent classes contain a drive() method which is overridden in the Hybrid class. Now, when we try to call the drive() method of the Hybrid car, the compiler will get confused about which method to call as both the parent classes (CNG_Car and Petrol_Car) have the same signature name method (drive() method). This results in a compile-time error due to diamond problem ambiguity. Because of this ambiguity, Java doesn't support multiple inheritance of classes. Despite this fact, we can indirectly implement multiple inheritance in Java using an interface that is discussed further in the article.
Interface Inheritance
An interface is a fully abstract class i.e., it includes a set of abstract methods that specifies what a class must do and not how to do it. Hence, if a class implements an interface, it inherits all the abstract methods of the interface and provides an implementation for each of them.
Interfaces are non-instantiable and provide a representation of what a class should implement i.e., interfaces do not contain detailed instructions for their behaviors. Because of this feature, a class can implement any number of interfaces without any ambiguity as the implementation is provided by the class itself.
Now, we can effectively bypass the diamond problem and implement multiple inheritance in Java using the interface. Let's revisit our Car example and instead of using two classes (Petrol_Car and CNG_Car), let's use two interfaces and implement the drive() method in our Hybrid_Car class as shown in the figure below:
Hence, we can implement multiple inheritance in Java using Interface. The above application structure can be implemented in Java programming language using the code given below:
Code:
Output:
In the above program, we are declaring two interfaces Petrol_Car and CNG_Car using the interface keyword. These interfaces represent the additional capability (abstract methods) that must be implemented by the implementation class (Hybrid_Car). Here, multiple inheritance is achieved as the Hybrid_Car class implements both these interfaces.
Conclusion
- Multiple inheritance is a special type of inheritance in which a class can inherit properties of more than one parent class.
- Java doesn't support multiple inheritance of classes due to the diamond problem ambiguity.
- Diamond problem ambiguity arises when the same signature exists in both the superclasses and the compiler cannot determine which signature to call in the subclass.
- We can indirectly implement multiple inheritance in Java using the interface.
- An interface is a blueprint of a class that provides a set of abstract methods that a class must implement.
- Multiple inheritance by interface occurs if a class implements multiple interfaces or also if an interface itself extends multiple interfaces.