How to Take Multiple Input in Python?
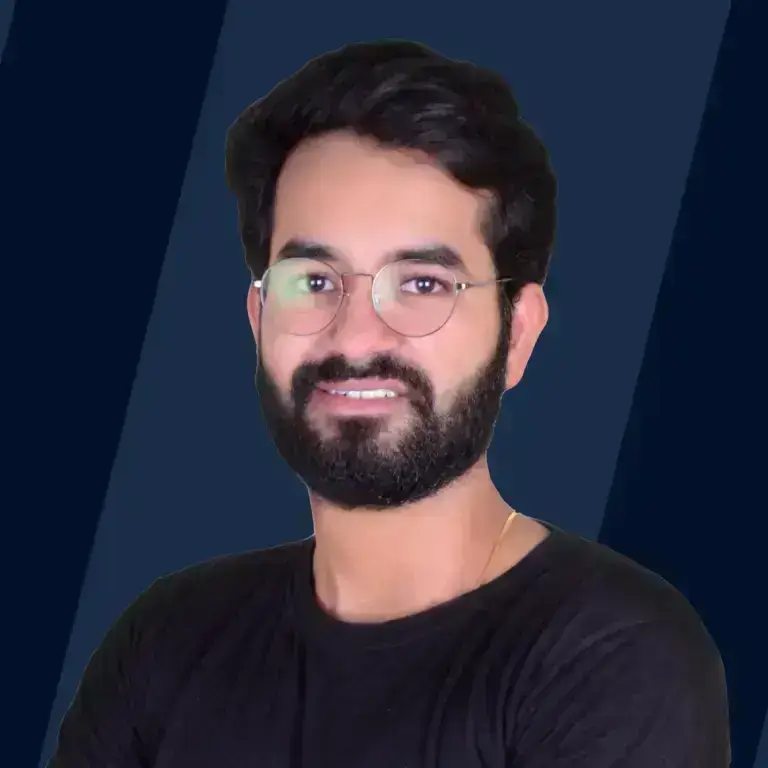
Overview
The below module expands our knowledge when we encounter scenarios where it is a mandatory task to give multiple input in Python. To avoid the repetitive use of the inbuilt functions from Python which eventually makes the program heavy we have three functions in Python split(), list comprehension, and map() function in python to help us resolve the problem where we are required to give multiple input in Python.
How to take Multiple Inputs in Python?
While writing code for programs, we may come across scenarios where we may need to provide multiple input in Python to make our programs easier to understand and reduce the load on the file memory. For doing so, you may perform several operations on the input provided by the user where you might have worked with the inbuilt methods provided by Python and segregate the input as raw_input () and input (). While this may help you to take multiple input in Python, it is important to note that when we use the same method or function, again and again, in a program it tends to make the code complex and increase the heaviness of file memory.
To solve this issue and resolve our scenario, we shall understand the concepts around multiple input in Python. We may have heard of the scanf() function from the C/ C++ programming language but we do have the function in python as well which can help us to take multiple input in Python that too in one single line.
With the below article, we shall expand our knowledge and understanding to study methods where we can take multiple input in Python in a single line and which will help us to write short and efficient code by learning the following three methods:
- Using the split() method
- Using the List Comprehension
- Using Map() function
Let us understand each method as we proceed with this article.
Taking Multiple Input in Python Using the split() Method
Introduction
To give multiple input in Python, the split() function in Python helps to split the multiple input fed by the user into single individual values for each variable. The method takes two parameters as part of its syntax that is, the separator and the maxsplit. When we input values in a single line for our multiple variables, the split function splits the input identifying it with the specific separator. When the separator is not given then white space is taken as a default separator.
The programmers implement the split() function to split the Python string, but we can also use it to get multiple values.
Syntax
The syntax for the split() function is as below:
Where we give separator and maxsplit as the parameters.
The input can be given by the user in a single line for multiple variables depending on the scenario.
Parameter
The parameter for the split(` function is as below:
- The separator: The separator acts as a delimiter which is considered as whitespace if undefined or else whatever is defined is taken as the separator. The values we input for the individual variables get splitting at this defined separator.
- The maxsplit: The maxsplit is the number that tells us to divide the string where the number mentioned is the maximum of the number of times. By default, -1 is considered which means there is no limit as such.
Both parameters are optional that is, if none are mentioned then the default values of both parameters are taken for splitting the multiple input in Python.
Code:
Below code, we are exploring the split() function for multiple input in Python which lets us input values to variables in a single line. We have considered three scenarios where we are exploring the split() function when we input two inputs, three input, and unlimited inputs ins a single line and obtain individual values getting assigned to each variable.
Output
Explanation: As seen in the above example we have considered three scenarios where we implement the logic of the split() function that we just studied where we input the values to variables in a single line as a multiple input in Python. In case 1 we are talking about asking the user to input two values of two variables in a single line separated by space as we have not mentioned the delimiter. Similarly, we obtain the input for three variables in case 2. In both these cases, we use the split function to obtain the individual values for each variable by inputting the value as a single line. Also, we can notice that the input is given in any form: int, string, float, etc. as we have not mentioned it explicitly and so whitespace is considered as a delimiter by default.
For case 3, we have given the delimiter as "," where we see that even if we don't define the max split, the outputs are unaffected.
Taking multiple inputs in Python Using List Comprehension
Introduction
To create or define an elegant and efficient list in Python we can always implement List comprehension. Here the usage of list comprehension helps us take multiple input in Python and create a list as easily as mathematical statements that too in a single line.
To take multiple input in Python from the user for assigning individual values to multiple variables separately, we can also use the list comprehension method that allows us to take values and efficiently convert them into a list by using either the split() or the map() function.
Using the List Comprehension via split() function
We shall be studying the use of the split() function to take multiple input in Python by the list comprehension method.
Syntax The syntax for list comprehension to feed multiple input in Python is as below:
where we use the square bracket to represent the list and implement the split function to divide the multiple input in Python into individual variables.
Code: Below code we are exploring how we can use the list comprehension along with implementing the split() function to feed multiple input in Python which lets us input values to variables in a single line. We have considered three scenarios where we are exploring the list comprehension methods in addition to the implementation of the split() function when we input two inputs, three input, and unlimited inputs ins a single line and obtain individual values getting assigned to each variable.
Output
Explanation: As seen in the above example, we have considered three scenarios where we implement the logic of list comprehension in addition to the implementation of the split() function that we just studied, where we input the values to variables in a single line as a multiple input in Python. In case 1, we are asking the user to input two values of two variables in a single line separated by space as we have not mentioned the delimiter.
Similarly, we obtain the input for three variables in case 2 where we give white space as a delimiter in subcase 1 and the delimiter as "," in subcase 2 to take multiple input in Python.
In both these cases, we use the list comprehension and represent it by using the square brackets and for loop in addition to the split function to obtain the individual values for each variable by inputting the value in a single line. Also, we can notice that the input here is restricted to int where we have the flexibility to assign any form: int, string, float, etc. For case 3, we have given the delimiter as "," where we see that we obtain the output as a list for multiple input in Python.
Using the List Comprehension via map() function
We shall be studying the use of the map() function to take multiple input in Python by the list comprehension method.
map() function is also widely implemented by the developers while taking multiple input in Python from the user.
Syntax The syntax for list comprehension to feed multiple input in Python is as below:
where we use the list function to draw a list of values for each variable by giving multiple input in Python in a single line. We make use of the map() function as shown in syntax to divide the multiple input in Python into individual variables.
Code: Below the code we are exploring how we can use the list comprehension along with implementing the map() function to feed multiple input in Python which lets us input values to variables in a single line. We have considered three scenarios where we are exploring the list comprehension methods in addition to the implementation of the map() function when we input two inputs, three input, and unlimited inputs in a single line and obtain individual values getting assigned to each variable.
Output
Explanation: As seen in the above example we have considered three scenarios where we implement the logic of list comprehension in addition to the implementation of the map() function that we just studied where we input the values to variables in a single line as a multiple input in Python. In case 1 we are talking about asking the user to input two values of two variables in a single line separated by space as well as a delimiter ",".
Similarly, we obtain the input for three variables in case 2 where we are giving white space as a delimiter in subcase 1 and giving the delimiter as "," in subcase 2 to take multiple input in Python. In both these cases, we use list comprehension and by making use of the map() function the values get assigned to individual variables by inputting values in a single line for multiple variables. Also, we can notice that the input here is restricted to int where e have the flexibility to assign any form: int, string, float, etc. For case 3, we have not given any delimiter, as a result, the whitespace is the default delimiter or separator and we see that we obtain the output as a list for multiple input in Python.
Know more about input and output in Python with scaler topics
In the above scenarios, we studied taking multiple input in Python from the user in a single line which reduces the heaviness of the program and also makes the code more efficient as it reduces the code complexity as well by not using the Python inbuilt function repetitively.
We come across the input and output in Python and understanding the concepts around it is also very important.
To dive deep into the logical concepts for the same please refer to the below article linked down to expand your knowledge for same.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.
Conclusion
-
To stop using the same inbuilt functions again and again which makes the program heavy and the code complex, we can take multiple input in Python in a single line and which will help us to write short and efficient code by the three methods we learned in the article as stated below:
- Using the split() method
- Using the List Comprehension
- Using Map () function
-
To give multiple input in Python, the split() function in Python helps to split the multiple input fed by the user into single individual values for each variable. The method takes two parameters as part of its syntax that is, the separator and the maxsplit where the separator is any delimiter or whitespace by default and the maxsplit is the maximum number of times the variables could be split which is -1 by default.
-
To take multiple input in Python from a user for assigning individual values to multiple variables separately we can also use the list comprehension method that allows us to take values and efficiently convert them into a list by using either the split() or the map() function.