Multiplication of Two Matrix in Java
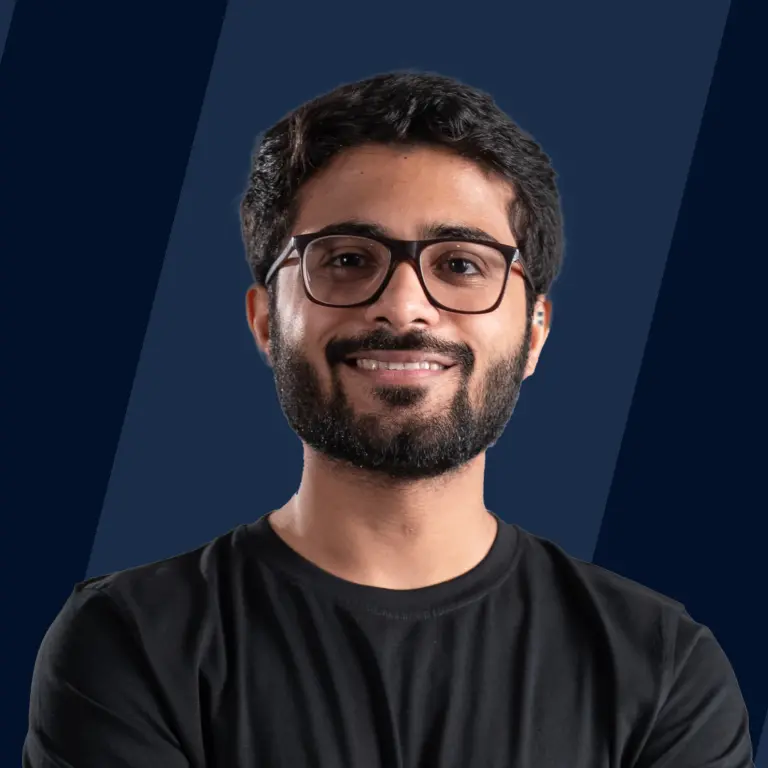
Overview
Matrix multiplication has many applications in various studies. Matrix multiplication involves taking a matrix as input from the user, checking if multiplication is possible for the given two matrices, knowing the dimensions of the resultant matrix, and finally finding the resultant matrix and printing the matrix.
Matrix Multiplication
Matrix multiplication has facilitated and clarified computations in linear algebra. Matrix multiplication and linear algebra are fundamental in all mathematics, as well as in physics, chemistry, engineering, and computer science. Thus, it is very important to derive a product matrix from two matrices. The matrix in programming can be represented by a 2D array. The 2D array is nothing but an array of arrays.
Taking Matrix as Input
For taking the matrix as input from the user, first, ask for the number of rows and columns of the respective matrix. Secondly, for inserting values in an array/matrix, ask for input values in row-major format i.e. a matrix (2D array) write all the column values of the first row, then the second row, and so on. Run 2 for loops, outer for the rows, and inner for the columns. The outer one is static until all the inner values (in the columns of that row) are filled. At last, we return the matrix filled with values. This method doesn't take any parameters.
Checking if Multiplication is Possible
Two matrices can only be multiplied if the number of columns of the first matrix is equal to the number of rows of the second matrix. Therefore, it is necessary to check if the two matrices are compatible with multiplication.
This method returns a boolean value. If the number of columns of the first matrix is equal to the number of rows of the second matrix it will return true else it will return false.
Dimensions of the Resultant Matrix
If two matrices can be multiplied the resultant matrix will have dimensions as follows:
Multiplication of Matrix
Multiplication of two matrices is done in the following way mathematically:
Here, A and B are two matrices to be multiplied, and C is the resultant matrix or matrix product of A and B.
Let us see an example to understand matrix multiplication in mathematics. Here, we multiply 2 matrices of 3X3 dimensions each. Thus, the resultant matrix should also be of 3X3 dimensions.
Matrix multiplication in java is the same as that in Mathematics. Let us see the multiplication of matrices in Java.
Dimensions of the new matrix are equal to the number of rows of matrix1 X the number of columns of matrix2. Thus we take rows1 and cols2, and new matrix ans with the same dimensions. We fill the 2D array with 0 to avoid NullPointerException which is thrown if the element of the array is not initialized. i in the outer for-loop visits each row of the first matrix, j in the first inner for-loop visits each column of the second matrix. Combination i and j together fills the resultant array ans and k starts from 0 to one less than the number of rows of matrix2 or the number of columns of matrix1. k is column number for matrix1 and row number for matrix2. In the end, we return the matrix which is the multiplication answer of the two matrices passed as parameters.
Printing Matrix
To print a matrix i.e. 2D array, simply run nested for loop. Outer one for rows and the inner one for columns, visit each position and print the value at the respective position. Go to the next line for a new row.
Program to Multiply Two Matrices
Let us take an example of two matrices for multiplication. We begin by taking two matrices as input from the user. Then check for the possibility of multiplication and finally print the resultant matrix that we obtain after multiplying the two matrices.
In the example above, the first matrix is of the dimensions 2X3 and the second matrix has dimensions 3X2. Matrix multiplication is possible as the number of columns of matrix1 i.e. 3 is equal to the number of rows of matrix2 i.e. 3. So, the resultant matrix has dimensions 2X2 and the resultant matrix is as follows.
Output:
Example: Multiplying More than 2 Matrices
It is possible to multiply more than 2 matrices. Let us take an example to multiply 3 matrices.
Output:
Here we first check if it's possible to multiply the first two matrices, if possible we multiply them. The result of the multiplication of the first two matrices is stored in the temp array. temp is later multiplied by the 3rd matrix if the is_possible method returns true and stores in ans. Thus, ans is the required matrix and we print it using the print_matrix method.
Conclusion
- Matric multiplication and linear algebra are very important in computer science, engineering, physics, and chemistry.
- Before multiplying two matrices, it is necessary to check if multiplication is possible i.e the number of columns of the first matrix equals the number of rows of the second matrix.
- Dimensions of the matrix product are number of rows of matrix1 X number of columns of matrix2
- Multiplication of matrix in Java is the same as mathematical multiplication.
- Also, it is possible to multiply more than 2 matrices if they are compatible with multiplication.