C Program to Generate Multiplication Table
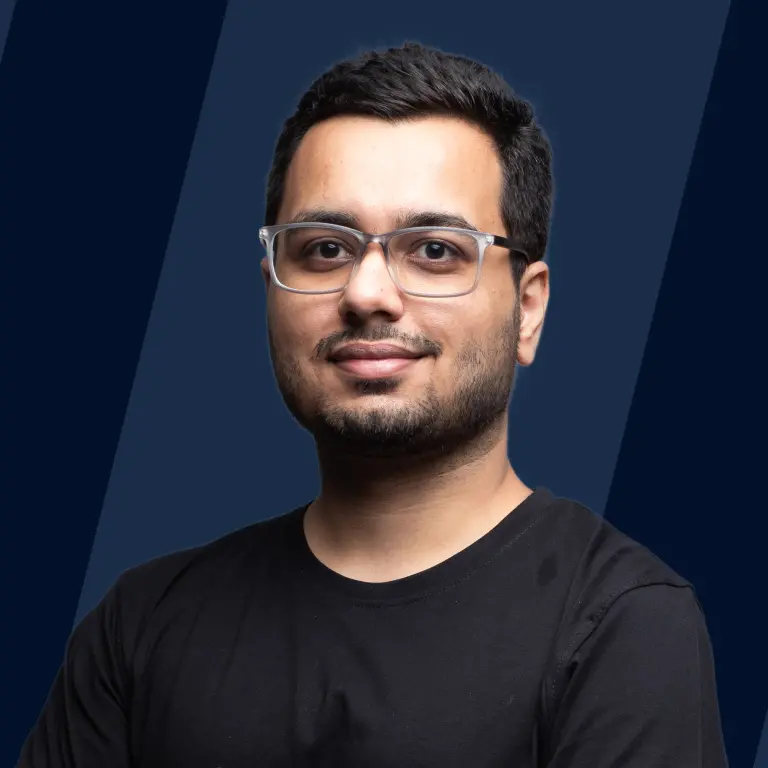
Overview
C Program to generate multiplication table is one of the common and interesting programs that every beginner comes across, One of the reasons to implement the multiplication table in C program is that it gives us a good understanding of using loops.
Logic to print multiplication table
Here, we select an integer to print the multiplication table. Then we run a loop from 1 till 10 so that for every iteration we print the multiplied time using the multiplication operator in the table format.
Algorithm:
Step 1: Declare two variables i, num (i for iterating over loop, num for defining number)
Step 2: Initialize the num variable with an Integer value
Step 3: Initialize a for loop with 1 as starting value and run the for loop till 10. Ideally for loop would look like: for(i=1;i<=10;i++)
Step 3.1: For every iteration of the loop print the value in the required format as number*i. (Remember that the i value increases for every iteration)
Output:
Explanation for the above program
In the above program, we have taken a decimal number num=13, and we have taken a for loop to print the multiplication table. For loop will terminate when i becomes 10.
In the for loop we print the multiplication table by computing number*i for every iteration.
Multiplication Table up to 10
Here, let us discuss how to print a multiplication table in C up to 10 by taking user input.
Initially, we will take the user input for the multiplication table numbers. Then we run for loop from 1 till 10 and we print the multiplied time using the multiplication operator in the required format for every iteration.
Algorithm:
Step 1: Let us initialize two variables as i, and num (num is used for user input, and i is used for iteration)
Step 2: Take the input of the user and store it in the num variable
Step 3: Now using the for loop print the multiplication table starting from 1 till 10
- Step 3.1: For every iteration in the for loop print the value using number*i
Output:
Explanation for the above program
In the above program, we have declared two variables i, and number. i variable is used for loop iteration, and the number variable is used to store the user input. After taking the user input and storing the input value in the number variable we run the for loop from 1 to 10. For every iteration of the for loop we print the value by computing number*i
Multiplication Table up to a Range
Now let us discuss another program which is a slight modification of the above two discussed methods. Here Along with the number, we will also input the range (range defines the maximum printable). range defines the maximum printable time. range will be used in the for loop as an upper limit so that loop does not execute after it. By this, we will be able to print desired size multiplication table in C.
Output:
Explanation for the above program
In the above program, we have declared three variables i, number, and range. i variable is used for loop iteration, the number variable is used for storing the user input and the range variable is used to define the range.
Initially, the program asks the user to input a number and then asks the user to input a range. Now for loop starts to execute from 1 till range. For every iteration of the for loop, we compute the value using number*i.
Conclusion
Here we come to an end of our discussion on C program to generate multiplication table in C, Let us quickly recollect what we have discussed in the article
- We made use of for loop to generate a multiplication table in C.
- We can specify range so that the multiplication table in C will be printed up to that range