Neon Number in Java
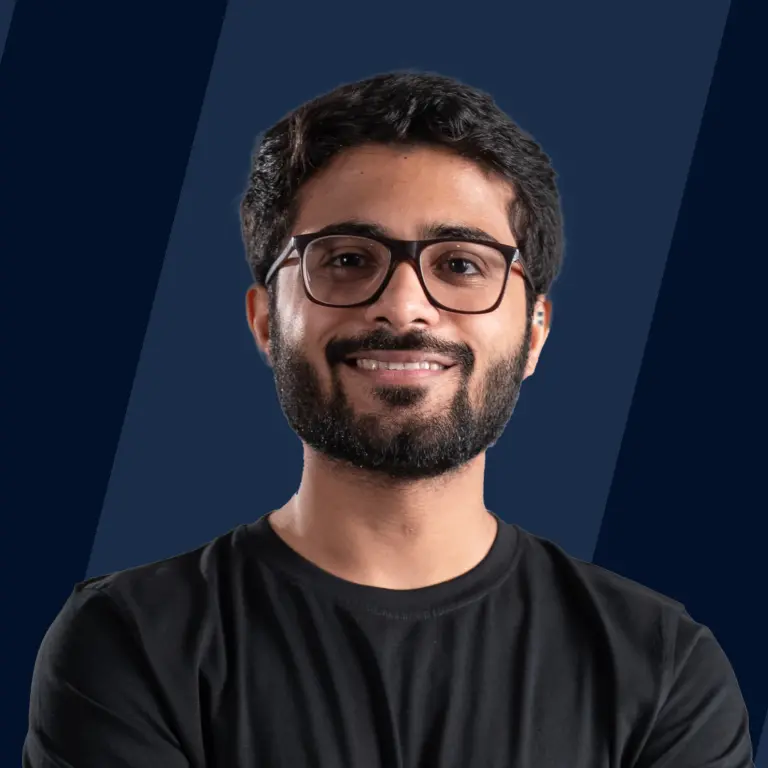
Neon numbers are the numbers whose square is equal to the sum of its digits. These are used in number theory, recreational mathematics, or mathematical puzzles. There are only 3 neon numbers (0, 1, 9) in the first 1 lakh numbers tested, this is the beauty and elegance that is only found in mathematical structures.
Example of Neon Number
Input:
Output:
Input:
Output:
Steps to Find Neon Number
To find the Neon Number following steps are to be followed:
- Take an input number from the user.
- Calculate the square of the number.
- Calculate the sum of digits of the computed square.
- Check if this sum is equal to the initial input number.
- Print the output accordingly.
Simple Program to Find Neon Number in Java
Let us write a Java Program to detect whether the number given is a Neon Number.
Step - 1:
Take an input number from the user.
- This can be done using the Scanner class in Java. We will ask the user to enter a number.
The user's input is stored in num.
Step - 2:
Calculate the square of the number
-
This can be done in two ways:
-
We can either multiply the number by itself
-
Or we can use the sqrt method of Math class
Step - 3:
Compute the sum of digits of the computed square.
- To calculate the sum of each digit of the number we we run a while loop until our number is greater than 0.
- For each iteration, we take the modulus of the number with 10 to get the digit at the unit's place and divide it by 10 to get the next digit.
Steps - 4 & 5:
Check if this sum is equal to the initial input number. Print the Output Accordingly.
- Now, we simply compare this sum with the input number.
The complete code is as follows:
Output
Complexity Analysis:
- Time Complexity:
The relationship between the number of digits in the square of a number is proportional to log(num). - Space Complexity:
No additional space is used.
Recursive Approach to Find Neon Number in Java
Let us understand it step by step. The approach is the same, we still calculate the square and get each digit of the square. But instead of calculating the sum we simply subtract it from the number. So, the while loop is eliminated and recursion is used.
Let's break it down into steps.
-
The function takes the input number and its square as input.
-
Now in each recursive call:
-
First, we check if the square is 0 (same condition as in the while loop).
- If it is true, then we check if the num is 0.
- If the condition satisfies we return true else we return false.
-
Else:
- Now, we get the unit's digit.
- Divide the square by 10
- And subtract this digit from the input number.
- Again the function is called recursively, with the new values of square and num.
-
The code for the same is as follows:
Output
Complexity Analysis:
- Time Complexity:
- Space Complexity:
As we are using recursion, the recursive calls are stored in the recursion stack and thus, it adds up to the space complexity.
Conclusion
- Neon number is a magical number where the sum of digits of the square of the number equals the number itself.
- We are using two approaches to find the Neon Numbers.
- In the first method, the square of the number is calculated, and the sum of the digits of the square is checked with the number. The second method uses recursion for the same approach.
- The first method is better as the time complexity is the same for both approaches but the space complexity of the first method is less.