Nested For Loop in C Programming
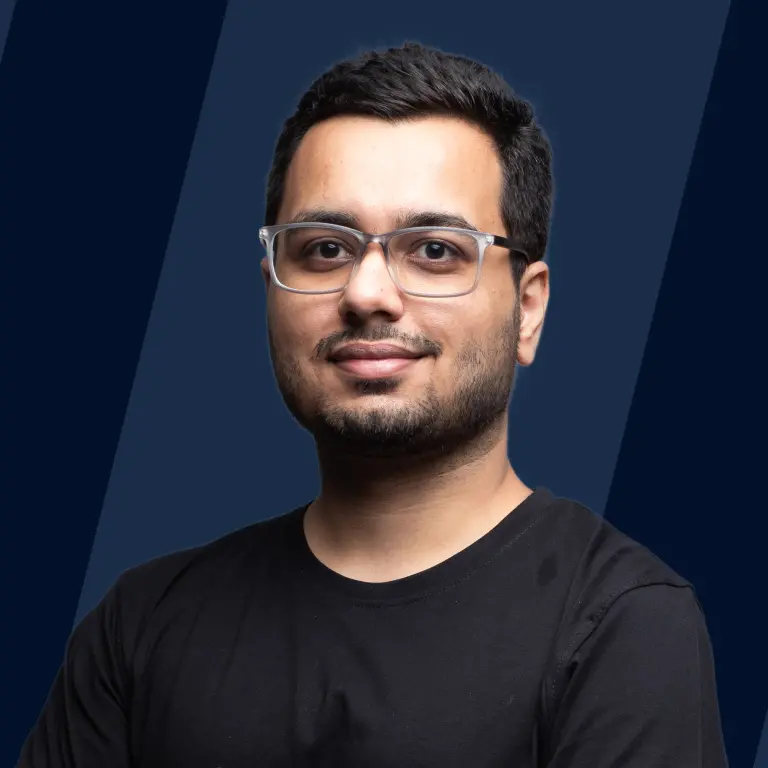
Overview
A Nested for loop is a strong technique in C programming that allows us to tackle difficult jobs easily. Consider it as loops inside loops, similar to Russian dolls. Nested loops run many iterations inside one another, much like opening one doll to discover another inside. We iterate over a list using a simple for loop. We resort to nested loops when faced with several dimensions or sophisticated patterns. Each repetition of the outer loop causes the inner loop to finish its path. With stacked loops, you can handle complex processes with ease and finesse.
Syntax
The for loop is an essential construct for iterating through code sequences in C programming. Its syntax is elegantly simple yet powerful, enabling developers to have precise control over iteration processes.
The syntax follows this structure:
nitially, the initialization step sets up the loop, initializing the loop control variable. The condition serves as the gatekeeper, determining whether the loop should continue based on its truth value. If the condition holds, the loop's code is executed. The update step alters the loop control variable after each iteration.
For instance, consider calculating the sum of integers from 1 to 10 using a for loop:
When dealing with nested for loops, which are loops within loops, adhere to best practices to enhance modularity, readability, and code maintainability:
- Use Descriptive Variable Names: Employ meaningful variable names like row and column instead of generic i and j. This enhances code readability and clarifies the purpose of each loop.
- Indentation and Formatting: Maintain consistent indentation and code formatting. Properly indent the inner loops to visually represent the nesting. Use whitespace to make the code more readable.
- Modularize Inner Loops: If the inner loop's purpose is complex, consider encapsulating it in a separate function with a descriptive name. This promotes code modularity and reduces complexity.
- Comments and Documentation: Add comments to clarify the intent of nested loops, especially if the logic is intricate. Good documentation helps other developers understand your code.
- Optimize When Possible: Evaluate whether you can optimize nested loops by reducing unnecessary iterations or using more efficient algorithms. This can lead to performance improvements.
Structure of Nested for Loop
A nested for loop is technically comprised of an outer loop and one or more inner loops. The outer loop initiates the process, which encases the inner loops. The inner loops complete their journey with each repetition of the outer loop. This configuration enables you to complete complex activities by breaking them into smaller, more manageable chunks.
As an example, suppose you're constructing a chessboard. The outer loop might represent rows, while the inner loop is responsible for each cell inside a row. You can effectively move around each square on the board by merging the two loops.
Parallelization is the technique of dividing a task into smaller, independent parts and processing them simultaneously using multiple CPU cores. In nested loops, it can be applied by distributing the inner loop iterations across cores, harnessing the power of multi-core processors to achieve faster and more efficient computation.
Nested for Loop Flow Chart
Nested loops are a useful tool for completing complicated operations in programming. Visualizing their business with a flowchart can bring useful insights into their complex operation.
A nested for loop is formed by enclosing one for loop within another. This structure allows for the simultaneous iteration of several variables, providing a strong approach for dealing with multidimensional arrays or matrices. Typically, the flowchart depiction of such a design begins with the outer loop, outlining the setup, condition, and increment processes. The inner loop is conducted after each iteration of the outer loop in a similar manner. This enables complete coverage of data items, as well as quick handling of operations that involve traversing grids of values.
Examples
For example, consider a scenario where you need to print a pattern of asterisks in descending order. The following code snippet achieves this using nested for loops:
Output:
Flowchart Explanation:
- To iterate across rows, start the outer loop with i.
- Begin the inner loop with j within the outer loop to regulate the number of asterisks per row.
- Print an asterisk and a space for each iteration of the inner loop.
- After finishing each row, proceed to the next line.
Conclusion
- The nested for loop form promotes hierarchical iteration by embedding one loop inside another. This technique is helpful when working with multidimensional arrays and complicated data structures.
- A flow chart clearly represents the execution flow of the nested for loop. Each level of nesting correlates to a branch in the flow chart, making complicated algorithms easier to understand.
- Each loop in the nest may be fine-tuned using different initialization, condition, and iteration expressions. This control over individual loop behavior aids in developing customized and optimized algorithms.
- Understanding the flow chart helps optimize the algorithm by identifying duplicate iterations and potential bottlenecks. This results in faster execution and better memory utilization.
- While nested loops give enormous power, excessive layering can result in difficult-to-read and maintain code. It is critical to strike a balance between optimization and readability.