What is Nested if Statement in Python?
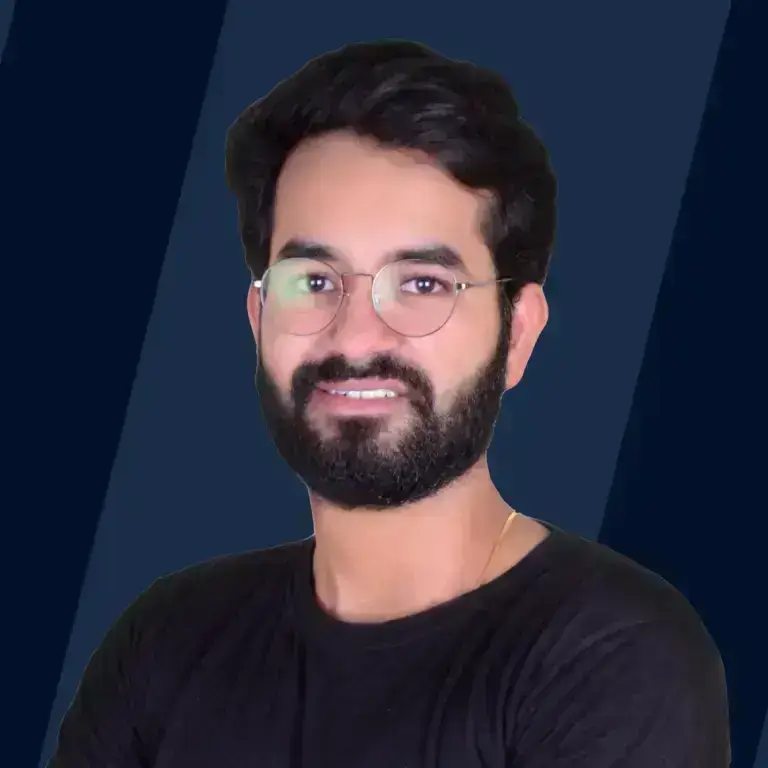
There come some situations in real life when we need to make some decisions and based on those decisions, we decide what we should do next. Just like that, these situations also arise in programming when we need to use if statements inside other if statements to control the program flow based on our decisions, called nested if statements.
The nested if statements in Python are the nesting of an if statement inside another if statement with or without an else statement. In some cases, we need nesting of if statements to make the entire program flow of code in a semantic order and make it easily readable.
Syntax of the Nested if Statements
The syntax of the nested if statements in Python is:
The syntax of the nested if statements can vary, but the main thing is an if-else block is nested inside another if-else block.
Flow Chart for Nested if Statements
We will now use a flow chart to visually understand the program flow of nested if else statements:
Python Program representation for the above flow chart:
If is true, we will check if is , if yes, the will execute, but if is false, the will execute.
If is , we will check for , if that is true, the will be executed, but if even this condition doesn't satisfy, the will be executed.
After this, the if-elif-else block will end and the rest of the program (i.e., ) will be executed.
Nested If Statement Examples in Python
Python Program to Check if a Given Year is a Leap Year or Not
A leap year occurs in each year which is an integer multiple of 4 and if the year is also a multiple of 100, it should also be a multiple of 400 to be a leap year.
In the Gregorian calendar, a leap year has 366 days instead of 365, by extending February (by 1 day) to 29 days rather than the common year's 28 days.
Algorithm:
Check if the year is divisible by 4, if not, it is not a leap year, if yes, check if the year is divisible by 100, if not, it is a leap year, if yes, check if the year is divisible by 400, if not, it is not a leap year, if yes, it is a leap year.
The condition to check if the year is divisible by 100 is nested inside the condition to check if the year is divisible by 4. and the condition to check if the year is divisible by 400 is nested inside the condition to check if the year is divisible by 100. Therefore, this example uses a nested if statement inside another nested if statement.
Code:
Output 1:
Output 2:
Explanation:
In the first output, the year 1900 is divisible by 4 but it is not divisible by 400 while being divisible by 100, hence it is not a leap year.
In the second output, the year 2000 is divisible by 4, 100, and 400, hence it is a leap year.
Python Program to Display the Greatest Among Three Numbers Using Nested if Statement
In this program, we will find out the greatest number among the three given numbers (a, b, and c).
Algorithm:
Check if the number a is greater than b, if yes, check if the number a is greater than c, if yes, a is the greatest number among the three.
If the number a is not greater than b, check if the number b is greater than c, if yes, check if the number b is greater than a, if yes, b is the greater number among the three.
If the number b is not greater than c, check if the number c is greater than a, if yes, check if the number c is greater than b, if yes, c is the greater number among the three.
Code:
Output 1:
Output 2:
Explanation:
In the first case, a=3, b=2, c=1. The number a is greater than and c, therefore, a=3 is the greatest number among the numbers 1, 2, 3.
In the second case, a=100, b=102, c=141. The number c is greater than a and b, therefore, c=141 is the greatest number among the numbers 100,102,141.
Python Program to Check if the Given Number is Positive or Negative
In this program, we will check if a number is positive, negative, or zero using nested if statements.
Algorithm:
Check if the number is greater than or equal to 0, if not, it is a negative number, if yes, check if the number is greater than 0, if not, it is 0, if yes, it is a positive number.
Code:
Output 1:
Output 2:
Output 3:
Explanation:
In the first case, the number 100 is greater than or equal to 0 and not equal to 0, so it is a positive number.
In the second case, the number -11 is less than 0, so it is a negative number.
In the third case, the number 0 is greater than or equal to 0 and equal to 0, so it is 0, neither positive nor negative.
Learn About if-else Statements in Python
The if-else statements in Python are used for decision making. They are used to run a block of code upon the satisfaction of the if condition, if that fails, we can also use several elif conditions to add different code for other conditions, and at, last we can use an else condition which runs a block of code if none of the above conditions are satisfied.
To learn more about if else statements in Python, click here
Conclusion
- The nested if statements are used to make decisions based on other decisions.
- Nesting of if statements mean to write an if condition inside another if condition.
- We can solve various problems like finding leap year, finding the greatest among three numbers, finding if the number is negative or positive, etc., easily.
- Using nested if statements are good practice for smooth and readable program flow.