Nested Loop in JavaScript with Examples
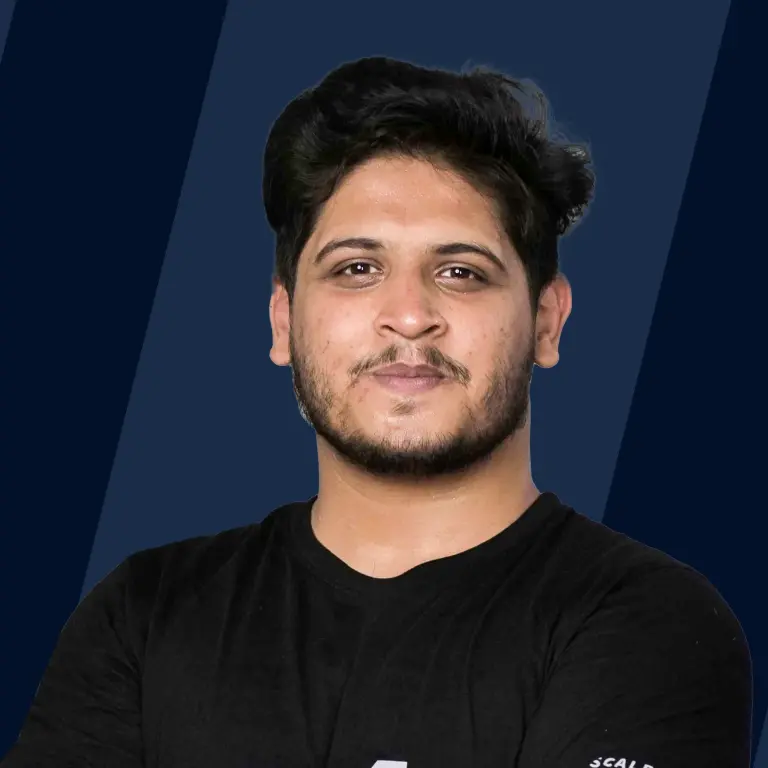
As the name implies, a nested loop consists of multiple loops nested inside one another. As a result, we can loop across multidimensional data structures like matrices. A nested loop is a construction where the body of one loop (the inner one) stays inside the body of another (the outer one). In each iteration of the outer loop, the whole inner loop is executed.
Syntax
Consider the snippet below. The expression inside the for loop body is again a for loop in a nested loop. This results in the inner for loop running completely for each iteration of the outer for loop.
In this example, the inner loop executes a specified number of times for each outer loop iteration. Thus, the loop executes outerLimit * innerLimit times altogether.
The execution process for a nested for loop is shown in the image above. As shown in the illustration, it is easy to see that the outer loop determines whether a condition is true first, then evaluates the nested loop and executes its body. It returns to the outer loop when the inner body has been completed, evaluates it, and then evaluates the inner body. This process is repeated until both evaluations are true.
Let us consider another example where a while loop and a for loop are nested inside a for loop. Here's the syntax for the same:
Note: The two inner loops are not nested within one another. The above example is a two-level nested loop having three loops.
Here's the syntax of n-level nested loops:
All loops have independent initialization values, terminating conditions, and loop variable updates.
Examples
Example 1
The following is an example that shows a for loop nested inside a while loop:
After clicking on the button, the output will be as given below:
Explanation:
This HTML and JavaScript code defines a webpage with a button. When the button is clicked, a function (myFunction()) is triggered, which uses nested loops to generate and display a message showing the values of variables i and j for each iteration. The outer loop runs 5 times, and the inner loop runs 2 times for each iteration of the outer loop. The resulting messages are appended to an HTML element with the id "did."
Example 2
The following is an example of three-level nested loop in JavaScript, using a mix of for and while loops:
Output:
Explanation:
The provided JavaScript code illustrates a three-level nested loop structure. This example consists of an outer for loop, a middle while loop, and an inner do-while loop. It consists of an outer loop that iterates three times, a middle loop inside the outer loop that iterates twice for each iteration of the outer loop, and an inner loop within the middle loop that iterates three times for each iteration of the middle loop. The loops generate output messages indicating the iteration levels.
How to Break Nested Loops?
To end a loop early, use the break statement. A label might be used with a break to manage the flow more accurately. A statement or a block of code may be given a label by applying an identifier to it followed by a colon (:).
The label name and corresponding loop should be followed by something other than another statement.
Example-1: Break from nested loop
Output:
Explanation: This JavaScript code demonstrates the use of the while loop to create a nested loop structure. The outer loop (while (i < 5)) iterates five times, and the inner loop (while (j < 5)) iterates within each iteration of the outer loop.
The code prints messages to the webpage during each iteration of both loops using document.write(). However, there's a break statement in the inner loop (if (j == 3) break;), causing it to terminate when j reaches 3. This break exits the inner loop but allows the outer loop to continue.
Additionally, there's another break statement in the outer loop (if (i == 3) break;), terminating the entire loop structure when i reaches 3. Consequently, the program writes "Hello World!" to the webpage only after a specific condition is met in both the outer and inner loops.
Example-2: Break from nested loop using Labels.
Output:
FAQs
Q. What is a nested loop in JavaScript?
A. A nested loop in JavaScript is a loop placed inside another loop. It allows you to iterate over elements or perform repetitive tasks within a loop, which is part of another loop. This is often used for complex iterations or working with multi-dimensional data structures.
Q. Why would I use nested loops?
A. Nested loops are used when you need to perform a repetitive task within a repetitive task. For example, you might use nested loops to iterate through rows and columns when working with multi-dimensional arrays. They are also useful for generating combinations, permutations, or comparing elements within multiple collections.
Q. How do I control the number of iterations in nested loops?
A. You can control the number of iterations in nested loops by specifying the loop conditions for both the outer and inner loops. You can use loop variables (e.g., i and j in the example above) and set the limits based on your requirements.
Conclusion
- JavaScript supports the concept of nested loops, where one loop is contained within another loop.
- A loop may define one, several, or n levels of nested loops inside another.
- The inner loop runs for each iteration of the outer loop.
- When the break or continue statement is used inside the inner loop, just the inner loop and not the outer loop is broken or continued.