Scanner nextInt() Java
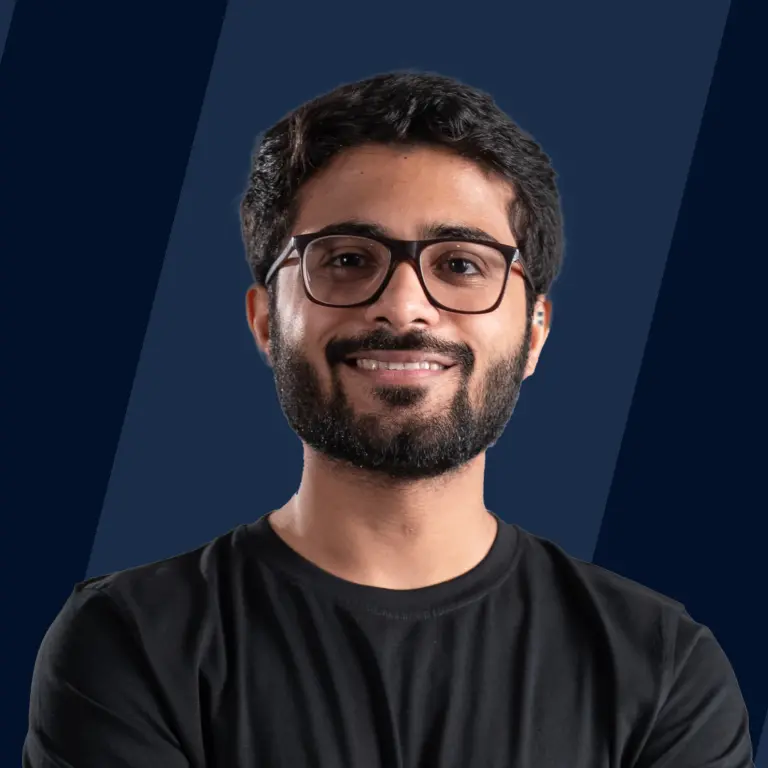
Overview
The nextInt() method of the java.util.Scanner class is used to read, scan, or parse the next token of an input as int. It can scan input through Scanner class from String, InputStream, File, etc.
Syntax of nextInt() Java
The syntax of the nextInt() method of the Scanner class is:
Without Radix
With Radix
Parameters of nextInt() Java
- The nextInt() method converts the input token to base 10 integer value. If no radix is passed, the default value is 10.
- The nextInt(radix) method converts the input token to the integer value using the specified radix. For example, scanning the value 5 with nextInt(3) throws an exception because 5 can't be represented in base 3.
Return Value of nextInt() Java
The nextInt() method parses the token from the input and returns the integer value.
Exceptions of nextInt() Java
The nextInt() method throws the below exceptions when it encounters an error parsing the token:
InputMismatchException
The InputMismatchException is thrown when the token parsed doesn't match integers regular expression or doesn't fit in the given radix.
NoSuchElementException
The NoSuchElementException is thrown when all the input tokens are parsed (i.e.) the input is exhausted.
IllegalStateException
The IllegalStateException is thrown when the nextInt() method is called on a Scanner after it is closed.
Example
Example 1: nextInt() Without Radix
In this example, we call the nextInt() method without any radix. By default, it uses 10 for radix.
Output
Explanation In each iteration, the token of the given input is parsed, and the nextInt() method converts the parsed token to int. The hasNext() method is used to check whether a token is available (i.e.) the input is not exhausted.
Example 2: nextInt() with Radix
In this example, we call the nextInt() method with the radix 3.
Output
Explanation We passed the radix 3 to the nextInt() method. So while scanning the tokens as int, they are converted to base 3 representation. The conversion of the number from base 10 to 3 is given below:
Number in Base 10 | Number in Base 3 |
---|---|
0 | 0 |
1 | 1 |
2 | 2 |
10 | 101 |
11 | 102 |
12 | 110 |
20 | 202 |
21 | 210 |
What is nextInt() Java?
Consider we have a string that contains numbers separated by spaces. We want to read the input string as an int and store it in an integer array. We can use Scanner that scans the input string and convert the integer strings to integers through its nextInt() method.
The nextInt() method reads the token as int. It default converts the token to a base 10 integer. We can also pass a radix to convert it to other base integers.
How does the nextInt() Method Work?
The nextInt() method reads the next token from the scanner input and checks if it matches the Integer regular expression, and then the token is converted to an int value. The nextInt() method also works for negative values.
The nextInt() method works for different Scanner input sources such as Readable, String, InputStream, File, etc.
More Examples
Example 1: nextInt() for InputStream
Output
Explanation
In this example, we parsed the input from InputStream that will be entered by the user and converted the token to int.
Example 2: InputMismatchException
Output
Explanation
When parsing the token 3, the compiler throws InputMismatchException because the number 3 is not part of base 3 numbers. Base 3 number system can contain only 0, 1, and 2.
Example 3: NoSuchElementException
Output
Explanation In this example, we are parsing the token without validating with hasNext(), so when the input is exhausted, it throws NoSuchElementException.
Example 4: IllegalStateException
Output
Explanation In this example, we closed the scanner using scanner.close() before calling its hasNext method and ended up with IllegalStateException.
Conclusion
- The nextInt() method is used to read, scan, or parse the next token of an input as int
- The nextInt() method belongs to the java.util.Scanner class.
- The nextInt() method can throw InputMismatchException, NoSuchElementException, or IllegalStateException.
- It works for both positive and negative values.