nextline() in Java
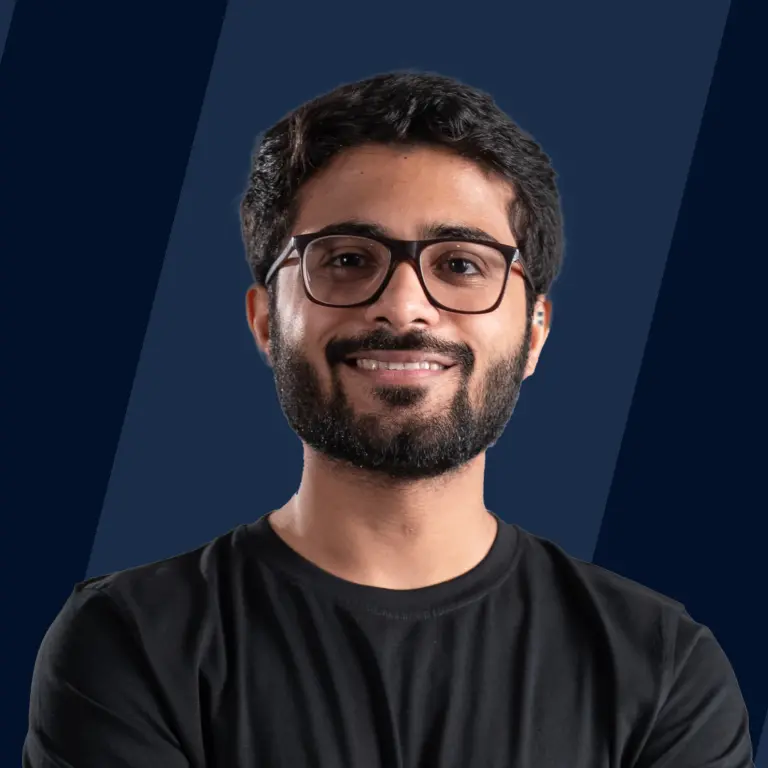
Overview
In this article, we are going to learn about the nextline() in java. Before getting started with the topic let us get a short overview of what is a nextline() in java.
nextline() in java : The nextLine() in Java is a method of the java.util.Scanner class. It will read the input string unless the line changes or a new line and then ends the input with \n or pressing enter. Usually, if we are taking any other type of input like int or String word input with the next() method before the nextLine() method we usually add an extra nextLine() method just before the nextLine() method, otherwise the nextLine() method will return a blank String. Another way would be to always use nextLine() wrapped into an Integer.parseInt.
We have got a brief overview of what is the nextLine() in Java. Now let us see the syntax of the nextLine() in Java.
Syntax of nextLine() in Java
Let us now discuss the syntax of the nextLine() in Java.
Declaration :
Explanation :
In the above syntax, we can see the nextLine() reads input only of String type.
Implementation :
Before implementing the nextLine() in Java, we must import the Scanner class in Java from java.util package. Let us look at how to import the same.
Importing Scanner class :
After importing the Scanner class, we create an object of the Scanner class and call the nextLine() method of the Scanner class with the object name followed by dot notation (.). the nextLine() method reads the input. Let us look at how we can call the nextLine() method.
Calling nextLine() method :
Let us also learn about the parameters taken by the nextLine() method in Java.
Parameter of nextLine() in Java
As we can see from the above syntax, the nextLine() method does not accept any parameter.
Return Values of nextLine() in Java
The nextLine() in Java returns the value in the string it returns the current line that was skipped.
Exceptions of nextLine() in Java
The nextLine() in Java throws two types of exceptions, let us discuss each of them.
- NoSuchElementException : The nextLine() in Java throws this type of exception when there is no line found in the input.
- IllegalStateException : The nextLine() in Java throws this type of exception if this scanner class is closed. When a Scanner is closed, it will close its input source if the source implements the Closeable interface. And the interface will no longer be able to take input from the user.
Example of nextLine() in Java
Let us now look at a small example of nextLine() in Java code, to make it more clearly understood.
Abstract :
In this code we will see how the nextLine() method reads the input string unless the line changes or a new line and then ends the input with \n or pressing enter and keep the next line input as a buffer for the next method.
Code :
Output :
Explanation :
In the above code, firstly, we are importing the Scanner class from the java.util package. After that, inside the public class MyClass, we are creating the object of the Scanner class (sc). While creating an object we can observe we are adding a String s variable to read the input from that variable. After that we are calling the nextLine() method of the Scanner class with the object name followed by dot notation (.) sc.nextLine(), and we are printing the String value. We can also observe, how the nextLine() method reads the input string unless the line changes or a new line and then ends the input with \n or pressing enter and keep the next line input as a buffer for the next method.
Internal Working of nextLine() Method in Java?
The nextLine() in Java is a method of the java.util.Scanner class, and it is used to store the user's input value of the String type. Before implementing this method to obtain the user's input, it is necessary to construct a Scanner object. The main purpose of this method is that it reads the input up till the end of the line. It will read the input string unless the line changes or a new line and then ends the input with \n or pressing enter.
Since nextLine() in Java, while looking for a line separator continues to read through the input, it may buffer all of the input reading for the line to ignore if there are no line separators present. nextLine() reads input including space between the words (that is, it reads till the end of line \n). Once the input is read, nextLine() positions the cursor in the next line.
Difference between next() and nextLine() Methods in Java
Before starting with the difference between the next() and nextLine() methods, let us get a short definition of what is next() method in Java.
next() method : The next() method in Java belongs to the Scanner class from the `Scanner.util package. It is mostly used to obtain input from users. To use this method we must construct the Scanner object at first. This method usually reads the single word in a String or up till it reaches the character space. For ex: if the String is "Hello World", this method will only read the word "Hello" and will buffer the next word.
Now, let us see the difference between next() and nextLine() methods in Java.
next() Method | nextLine() Method |
---|---|
This method usually reads the single word input from the user in a String or up to the input reaches the character space. | This method usually reads the input from the user until the next line. |
This method can’t read the words with blank spaces. | This method can read the words with blank spaces. |
After getting a blank space, this method stops reading input. | Once there is a line separator \n or pressing enter, this method stops reading the input, but it will read the blank spaces. |
After receiving input, the cursor is placed in the same place or the same line. | After receiving input, the cursor is placed on the next line. |
The escapes sequence that the next() refers to space. | The escapes sequence that the nextLine() refers to is line separator /n. |
The syntax of next() method is : sc.next() where sc is the object of the Scanner class. | The syntax of nextLine() method is : sc.nextLine() where sc is the object of the Scanner class. |
example : For String "Hello World" the next() method will read only the "Hello" word. | example : For String "Hello World" the nextLine() method will read the "Hello World" word. |
More Examples of nextLine() Method
Now, let us see some more examples of the nextLine() method in Java, for better understanding.
Example 1 :
Abstract :
In this example we will see the usage of the java.util.Scanner.nextLine() method. And we will see how the nextLine() method of the Scanner class returns the input taken from the user, and how the nextLine() method reads the input String including the blank spaces unless the line changes or a new line and then ends the input with \n or pressing enter and keep the next line input as a buffer for the next method.
Code :
Input :
Output :
In the above code, firstly, we are importing the Scanner class from the java.util package. After that, inside the public class MyClass, we are creating the object of the Scanner class (sc). While creating an object we can observe we are calling the parameterize Scanner constructor, in which we are giving System.in as a parameter, we usually provide this in the parameter of the Scanner constructor, when we want the input from the user. After that we are calling the nextLine() method of the Scanner class with the object name followed by dot notation (.) sc.nextLine(), and we are storing its value in a String variable, and then we are printing the String value. We can also observe, how the nextLine() method reads the input String unless the line changes or a new line and then ends the input with \n or pressing enter and keep the next line input as a buffer for the next method. Then we are closing the Scanner interface with the close() method, we usually add this sc.close() line at the end of our code, so that you won't be able to use System.in for the rest of your program. The fact that close() is passed through is nice because it means you don't have to maintain a separate reference to the input stream so that you can close it later. However, it is not mandatory to add this sc.close() line.
Example 2 :
Abstract :
Let us see another example of the nextLine() method in Java where we will include the blank spaces in the input.
Code :
Input :
Output :
Example 3 :
Abstract :
In this example we will see how the NoSuchElementException error occurs in the code when there is no line found in the input.
Code :
Input :
Output :
Explanation :
In the above code, we are taking the input three times for the nextLine() method in String, but while taking input, we have just given two line inputs, so it throws a NoSuchElementException error stating No line found. Hence, the input must consist of as many new lines as the nextLine() method is.
Example 4 :
Abstract :
In this example, we will see how the IllegalStateException error occurs in the code when the Scanner is closed.
Code :
Input :
Output :
Explanation :
In the above code, after calling the nextLine() method once, we are closing the Scanner by Scanner.close(). But after closing the Scanner class we are still calling the nextLine() method, so it throws an IllegalStateException error stating Scanner closed. Hence, we should call the nextLine() method before Scanner.close().
Example 5 :
We also encounter a very common mistake when we are using the nextLine() method. If we are taking any other type of input like int, double or any String word input with the next() method before the nextLine() method, we will observe that the nextLine() method will return a blank String. Let us see its example and understand why this happens.
Code :
Input :
Output :
Explanation :
In the above code, firstly, we are importing the Scanner class from java.util package. After that, inside the public class MyClass, we are creating the object of the Scanner class (sc). While creating an object we can observe we are calling the parameterize Scanner constructor, in which we are giving System.in as a parameter, we usually provide this in the parameter of the Scanner constructor, when we want the input from the user. After that we are calling the nextInt() method of the Scanner class with the object name followed by dot notation (.) sc.nextInt(), and we are storing its value in an integer variable, then we call the next() method of the Scanner class, and we are storing the String word in a String variable, then we are calling the nextLine() method of the Scanner class, and we string them next line input value in a String variable.
However, after printing the values we can observe the integer and the word is printed while the next line value is blank. This is because, after we stored the value in the word and we pressed enter, the extra change in line was buffered as input and when the nextLine() method started searching for its input, it gets the extra change in line in buffer, and just because there is no character before the extra change in line, the nextLine() method stores the blank value in the variable and prints a blank space.
Fixing the above issue:
To fix the above example's issue, we usually add an extra nextLine() method just before the nextLine() method whose value we are storing, by this the extra nextLine() will absorb the extra change in line, and then the nextLine() method after it will store the value perfectly in the String variable.
Code :
Input :
Output :
Conclusion
In this article, we learned about the nextLine() method in java. Let us recap the points we discussed throughout the article:
- The nextLine() in Java is a method of the java.util.Scanner class, and it is used to store the user's input value of the String type.
- The nextLine() method in Java will read the input string unless the line changes or a new line and then ends the input with \n or pressing enter. Since this method looks for a line separator to continue to read through the input, it may buffer all of the input reading for the line to ignore if there are no line separators present.
- Syntax of nextLine() : public String nextLine()
- The nextLine() method does not accepts any parameter.
- This method returns the value in the string, basically it returns the current line that was skipped.
- nextLine() in Java throws two type of exceptions : NoSuchElementException, IllegalStateException.
- The nextLine() in Java throws NoSuchElementException, when there is no line found in the input.
- The nextLine() in Java throws IllegalStateException, if this scanner class is closed.
- next() method usually reads the single word in a String or up till it reaches the character space.
- We have seen the difference between the next() and nextLine() method in Java. The* next() method can’t read the words with blank space, whereas nextLine() can read the words with blank space.
- For String "Hello World" the next() method will read only the "Hello" word, whereas the nextLine() method will read the "Hello World" word.
- We have seen different examples of nextLine() method in Java.