What is None Keyword in Python?
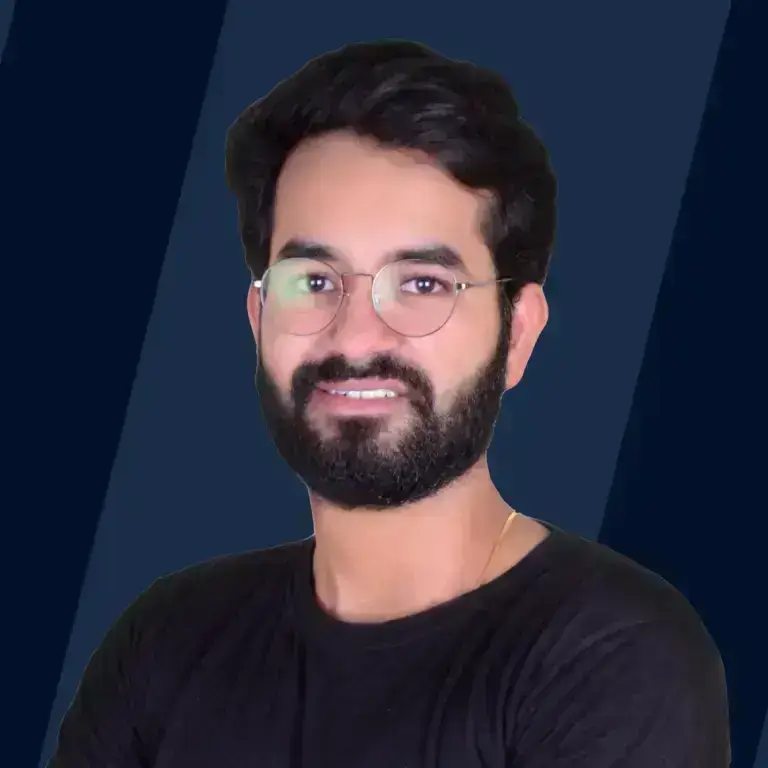
None is merely a keyword that is frequently used to denote "empty" or "no value." It is an object, and the only reason it has significance is that the Python documentation claims it does. Now, according to the Python Documentation --
The sole value of types.NoneType. None is frequently used to represent the absence of a value, as when default arguments are not passed to a function. Changed in version 2.4: Assignments to None are illegal and raise a SyntaxError."
Here, in this article, we will be discussing in-depth what is None Keyword in Python, its syntax, and various use cases which can enhance our Python code in a more pythonic way.
Introduction to None Keyword in Python
You have probably heard of the concept of null if you have worked with other programming languages like C or Java. This is a common way to designate empty variables, pointers that do not point anywhere and the default parameters that have not been explicitly specified. In such languages, null is frequently specified as 0. However, null in Python has a distinct meaning.
Python defines null objects and variables with the keyword None. The None keyword is used to define a "null value", in simple words no value at all. Please note that None is similar to, or cannot be assumed to be equal to 0, False, or an empty string. NoneType is the data type of None, and None can only be equal to None.
None is both an object and a first-class citizen in Python!
What are first-class citizens in Python?
Python functions are first-class objects, which means, the function in Python can be passed to other functions as arguments, or functions can be returned from other functions as values. The functions in Python can even be stored in variables and other data structures.
A few important properties of the None keyword in Python are:
-
The None keyword in Python is a data type and an object of the NoneType class.
-
The None keyword is used for defining a null variable or an object in Python.
-
None can be assigned to any variable, but new NoneType objects cannot be created.
-
We cannot change the reference to None by assigning values to it.
Syntax of None keyword in Python
The syntax of the None keyword in Python is very straightforward because it can be simply used by writing the keyword None. We do not need to explicitly define or import or perform any operation to use None in Python. Below given is the syntax for None in Python:
Interesting Facts about None in Python
Now that we have learned in detail about what is None keyword in Python, let us look into some of its interesting facts.
- None keyword in Python is not the same as the False.
- None is the same as 0 in Python.
- None is also not considered an empty string in Python.
- If we compare None to anything, it will always return False in Python, except while comparing it to None itself.
- A variable can be returned to its initial, empty state by being assigned the value of None.
- None keyword provides support for both is and == operators.
Examples of None keywords in Python
Let us now look into some of the most interesting examples for the None in Python to understand its uses better.
1. Check Whether a Variable is None Using the "is" Identity Operator:
We can check whether a variable is None or not by using the is identity operator in Python. Let us look into a code example for the same.
Code:
Output:
Explanation: In the above code, we checked whether a variable in Python is None or not using the "is" identity operator. For that, we directly checked the variable with the is None code snippet. Since the variable foo was None, we got the output as foo is None!.
2. Check Whether a Variable is None Using the "is not" Identity Operator:
We can check whether a variable is None or not by using the isnot identity operator in Python. Let us look into an example of the same.
Code:
Output:
Explanation: In the above code, we checked whether the variable we declared in Python is None or not by using the " is not" identity operator. To do so, we directly checked the variable using the is not None code snippet. Since the variable bar was not None, we got the output as bar is not None!.
3. Check Whether a Variable is None Using the "==" Relational Operator:
We can check whether a variable is None or not by using the == relational operator in Python. Let us look into an example of the same.
Code:
Output:
Explanation: In the above code, we checked whether a variable in Python is None or not using the "==" relational operator in Python. For that, we directly checked the variable with the == operator in the code. Since the variable bar was None, we got the output as bar is None!.
4. Check the Type of the "None" Object:
In this example, we will check the type of the None object by using the type() in Python. Let us look into the code for the same.
Code:
Output:
Explanation: In the above code, we checked the type of the None keyword in Python, which turned out to be from the class NoneType, as we discussed in the earlier sections of the article.
5. Comparing the None keyword with the None type in Python:
In this code example, we will compare the None keyword with the None type in Python. We are performing this comparison using the equality operator. Let us look into the code example for the same.
Code:
Output:
Explanation: In the above example, we saw that when we compared the None type with None, we got the result as True, because if we compare None with None using the equality operator, we get True as a result. Anything else, when compared with None using the equality operator results in False.
6. Comparing the None type with False in Python:
In this code example, we will compare the None keyword with the False in Python. We are performing this comparison using the equality operator. Let us look into the code example for the same.
Code:
Output:
Explanation: In the above example, we saw that when we compared the None type with False, we got the result as False, because if we compare None with anything apart from None itself, using the equality operator, we get False as a result. Hence, we got False as our result in the above code.
Ready to become a certified Python rockstar? Our course will guide you to success in the coding world.
7. Comparing the None with Empty string in Python:
In this code example, we will compare the None keyword with an empty string in Python. We are performing this comparison using the equality operator. Let us look into the code example for the same.
Code:
Output:
Explanation: In the above example, we saw that when we compared the None type with the empty string, we got the result as False, because if we compare None with anything apart from None itself, using the equality operator, we get False as a result. Hence, we got False as the result in the above code. We have also seen similar examples previously when we tried to compare None with False.
8. Working with None and Lists in Python:
While working with list in Python, we can check whether a list is empty or not, even assign None to a list, and perform several other operations with None and list in Python. Let us look at all of them in the below example.
Code:
Output:
Explanation: In the above example, we have included various scenarios of working with lists and None in Python. Let us just jolt down the points we conclude from the above examples:
- Lists can be initialized to None in Python
- If any variable is initialized to None explicitly, and we compare whether or not it is equal to None, then we will get the result as True.
- Empty list is not equal to None, which means, if we try to check whether an empty list is equal to None in Python, we will get the result as False.
Learn More
Now that you have got a clear and crisp idea about what is None in Python, I encourage you to go ahead and pick any of the below scaler articles to further enhance your knowledge in Python --
Conclusion
In this article, we learned about " What is None Keyword in Python ". Let us now quickly summarize what we studied till now --
- The None keyword in Python is a data type and an object of the NoneType class.
- The None keyword is used for defining a null variable or an object in Python.
- We can check whether a variable is None or not by using the is identity operator in Python.
- We can check whether a variable is None or not by using the == relational operator in Python.
- We can check the type of the None object by using the type() in Python.
- If we compare the None type with None using the equality operator, we will get the result as True. In other cases, we will get the result as False.
- We can perform various operations with the list and None keyword in Python.