Not Equal Operator in Python
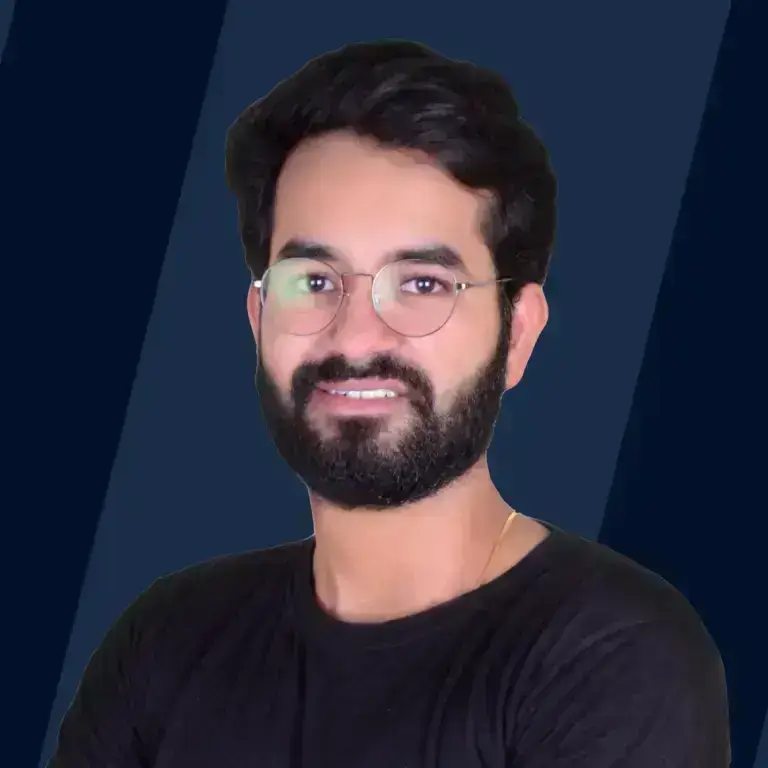
The Python NOT EQUAL (!=) operator compares two values. It returns True if the values are not equal and False if they are equal. In this article, we will learn about NOT EQUAL in Python.
Python NOT EQUAL Operator Syntax
- The syntax for the not equal to in Python is represented by the characters "!=".
- It is used to check whether two operands are not equal to each other. Here's the syntax:
- Let's see an example where we are comparing a string and a numeric type.
- Here, the string representation of the numeric value matches the string exactly, but the expression will evaluate to True because they are of different types, and Python treats it as different.
NOT EQUAL Operator in Python: Basic Use
The not equal to in python is represented by !=. It plays an important role in Python as it is used to do comparison between values.
We are able to compare values using not equal to operator in python.
How to compare numeric values using the != operator in Python
Here's an basic example demonstrating how to use the not equal in python operator to compare numeric values:
- In this example, number_1 and number_2 are two numeric values: 250 and 300.
- We will check whether these numbers are equal or not equal using the not equal != operator.
NOT EQUAL Operator in Python: Advanced Use
The not equal to in Python can be used in advanced scenarios like in loops and conditional statements where decision-making is required based on inequality.
Python Not Equal in Loops
Using the not equal to in python loops is a common practice in Python, allowing for dynamic iteration and filtering of elements based on inequality. Let's explore how it can be used in loop constructs:
- We started by defining a list named callednumbers containing integer values ranging from 11 to 20.
- We initiate a loop to iterate through each element (num) in the numbers list.
- Within the loop, we employ the not equal in python to check if the current element (num) is not equal to 20.
- Before the loop, we print a message to signify that we are about to print numbers not equal to 20.
- If the condition (num != 20) evaluates to True for a particular number in the list, that number (num) is printed.
Python Not Equal in Conditional Statements
Conditional statements in Python often based on the "not equal" operator (!=) to make decisions. Let's explore how it can be used in conditional statements:
Code
Output:
- Here, we define a variable named age and assign it the value 25.
- We use the not equal to in python to check if the value of the age variable is not equal to 30.
- If the condition age != 30 evaluates to True, indicating that the age is not equal to 30, this message is printed.
- If the condition age != 30 evaluates to False, meaning the age is indeed 30, this message is printed instead.
Python NOT EQUAL Operator with Custom Object
- The ne() gets called whenever the not equal operator is used.
- We can also override this function to chnage the nature of the not equal operator.
- Whenever the not equal operator is used, the ne() gets called.
- This function can be overriden to change the nature of Not Equal to (!=) operator.
Output:
Alternatives to NOT EQUAL Operator in Python
In Python, besides the "not equal" operator (!=), there are several alternative methods exist to express inequality or perform comparisons. Here are some common alternatives:
- Equal to Comparison with Negation
- Greater Than or Less Than Comparisons
- Inequality in Range Comparison
- Boolean Negation
- Using XOR Operator
1. Equal to comparison with negation:
Output:
Explanation:
In this method, we're comparing number_1 and number_2 using the equality operator (==). The not keyword negates the result of this comparison, effectively checking if number_1 is not equal to number_2. Since number_1 (50) is indeed not equal to number_2 (10), the condition evaluates to True, and the corresponding message "number_1 is not equal to number_2" is printed.
2. Greater than or less than comparisons:
Output:
Explanation: Here, we're comparing x and y using the greater than (>) and less than (<) operators. Since x (50) is greater than y (10), the condition x > y evaluates to True, and the message "x is greater than y" is printed.
3. Inequality in range comparison:
Output:
Explanation: In this method, we're using the not in operator to check if x is not in the range from 1 to 50. Since x (3) falls outside this range, the condition evaluates to True, and the message "x is not in the range from 1 to 50" is printed.
4. Boolean negation:
Output:
Explanation: Here, we're using the not keyword to negate the result of the equality comparison (x == y). Since x (50) is not equal to y (10), the condition evaluates to True, and the message "x is not equal to y" is printed.
5. Using XOR operator:
Output:
Explanation: In this code, we're using the XOR operator (^) to check for inequality between x and y. The XOR operator evaluates to True if the operands are different. Since x (50) and y (10) are indeed different, the condition evaluates to True, and the message "x is not equal to y" is printed.
Troubleshooting the NOT EQUAL Operator in Python
When working with the 'Not Equal' operator ('!='), encountering errors is not uncommon. These errors are commonly seen as either type errors or logical errors. Here's a detailed examination of how to troubleshoot and address these issues effectively:
Type Errors with 'Not Equal'
Type errors occur when there's a mismatch between the types of operands being compared. Below are strategies to identify and manage type errors:
- Check Operand Types: It's crucial to ensure that operands being compared are of compatible types. For instance, comparing an integer to a string can result in a type error.
Output:
- Convert Operand Types: If feasible, convert operands to compatible types before comparison.
Output:
Logical Errors with 'Not Equal'
Logical errors with the 'Not Equal' operator ('!=') may stem from misinterpretation of the logic. Here's how to address common logical errors:
- Negation Error: Ensure correct application of negation when using the 'Not Equal' operator in combination with other logical operators.
Output:
'Not Equal' operator used in conjunction with other logical operators
The 'Not Equal' (!=) operator is often used in conjunction with other logical operators in Python to create compound conditions for decision-making. Let's explore how the 'Not Equal' operator can be combined with other logical operators:
In this code:
- We defined two variables, number_1 and number_2, and assign them values of 50 and 10, respectively.
- We combine the 'Not Equal' (!=) operator with the 'and' logical operator (and). The condition checks whether both conditions are true:
- number_1 != number_2: Checks if number_1 is not equal to number_2.
- number_1 != 0: Checks if number_1 is not equal to 0.
- If both conditions (x != y and x != 0) are true, meaning x is not equal to both y and 0, the message "x is not equal to y and not equal to 0" is printed.
Explanation:
- This code demonstrates the use of the 'Not Equal' operator (!=) combine with other logical operators.
- By combining these operators, we can create a compound condition that checks if x is not equal to both y and 0 simultaneously.
- If the condition evaluates to true, the corresponding message is printed.
- This defines how the 'Not Equal' operator can be effectively used alongside other logical operators to use complex conditions for decision-making in Python programs.
Conclusion
- The (not equal to)!= operator in python is a relational operator to compare two values.
- The not equal in python operator returns True when the values for comparison are not equal.
- The not equal to operator False when the values for comparison are equal.
- The not equal to operator True even if the values to be compared are same but of different data types.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.