Null Pointer Exception in Java
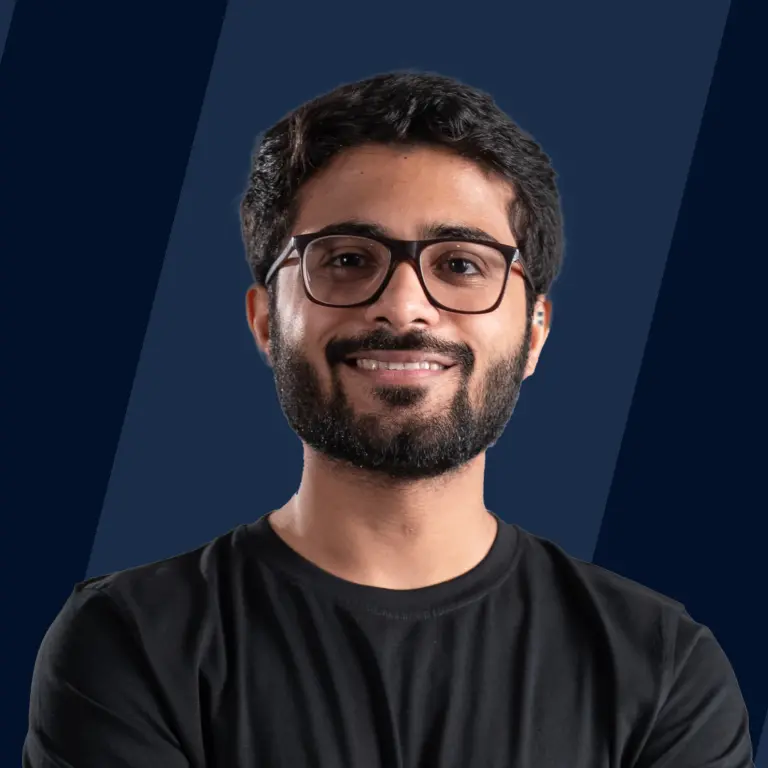
The null pointer exception in java is a runtime exception, which is thrown when the program tries to use an object reference that is set to the null value. Refer to the following coding example to understand the null pointer exception in java.
Example :
Output :
In this, we initialized the null value to an object, obj. On the obj object, we performed the toString() operation. Because the value of obj is null, it throws the null pointer exception, as shown in the output.
Let's try to understand the hierarchy of null pointer exceptions in java :
As we discussed, the null pointer exception in Java is a runtime exception, which is why it extends from the runtime exception class. The runtime exception class inherits the exception class. Throwable is a subclass of the object class, and the exception class is derived from the throwable class.
Reason for Null Pointer Exception
There are multiple scenarios where the null pointer exception occurs.
The Method is Invoked Using a Null Object
When we try to invoke the method with the null object, it gives the null pointer exception, as shown in the following code.
Output :
In the above program, there are 2 methods in the funClass; one of the methods is funMethod, which returns the null value for object obj on line 15. When we use this null object on line 18 to print the string, it gives the null pointer exception as we can't use the null object for method invocation.
Access Field of Null Object
Consider the following program where the null object is used to access the number field. It can raise the null pointer exception.
Output :
In the above program, there is a number field of type integer with variable name funNumber. When we use the null object on line 18 to access this number, it gives the null pointer exception as we can't use the null object to access the fields.
Passing Null Object as an Argument
In the following program, we passed the null value as an argument to the function. Due to the null object invoking method, a null pointer exception occurs.
Output :
The above program passes the null value to the function call at line number 9. But we can't perform the operations on the null string. That's why the null object invoking method null pointer exception occurs.
Getting Length of a Null Array
If we perform the in-built operations on the null array, it throws a null pointer exception, as in the following code.
Output :
In the above code, on line 5, we declared the array funArray and assigned it a null value. When we tried to use the length method on line 8, it gave the null pointer exception.
Access Index of a Null Array
If the array is null, and if we try to access the element by the index number of an array, then it gives the null pointer exception.
Output :
In the above code, we declare the array myArray on line 4 and assign it a null value. When we try to access the index of this null array on line 7, we get the null pointer exception.
Synchronization on a Null Object
We can't use the null value in a synchronized block; otherwise, it throws the null pointer exception, as demonstrated in the following program.
Output:
In the above program, we declared the variable mutexVar and assigned it a null value. On line 7, we used the null value in the synchronized block, which gave the null pointer exception.
By Throwing Null
If the program throws the null value directly, it can give the null pointer exception as demonstrated in the following program.
Output:
Requirement of NULL Value
The null value represents the no value assigned to the reference variable. There are multiple uses of null values while designing linked lists, trees, or singleton design patterns. Thus, null values are very useful in Java in various places. Its utility extends across various applications, including linked list and tree data structure implementation. Additionally, it finds application in design patterns like the Null Object pattern, which substitutes null references with objects that perform no action or provide default behaviour, and the Singleton pattern.
Output:
Explanation:
In the above example, the string name is made null and then we are trying to find the length of it, which causes the null pointer exception by which the code fails to run successfully. We can avoid it using the try-catch block statements.
Avoiding the NullPointerException
String Comparison with Literals
It is a common problem that the null pointer exception occurs when we invoke the equals method with the null object, as shown below.
Output:
We can avoid the null pointer exception in the above code by invoking the equals method with a literal instead of the myString variable on line 8.
Output:
Keeping a Check on the Arguments of a Method
We can avoid the null pointer exception in Java by checking whether the arguments are null. We can write a simple if condition, as shown in the following program.
Output:
Use of a Ternary Operator
It is also possible to use the ternary operator to check the condition of whether the given argument is null or not. We can check it as shown on line number 6 and line number 11 in the following program.
Output:
Conclusion
- Null pointer exception in Java is a runtime exception.
- Null pointer exception occurs when the program uses the reference of the object set to null.
- We can handle null pointer exceptions by making some changes in programs, such as invoking methods directly with literals instead of null objects.
- We can use the conditional checks to avoid the invalid object invocation.
FAQs
Q: How do you pass a NullPointerException in Java?
A: We handle it using try-catch blocks or by adding null checks.
Q: Should we catch NullPointerException in Java?
A: Yes, it's generally advisable to catch and handle NullPointerException in Java to prevent unexpected program termination.
Q: What is NullPointerException caused by?
A: NullPointerException is typically caused by attempting to access or modify an object reference that is null.