Building a Number Guessing Game in C++
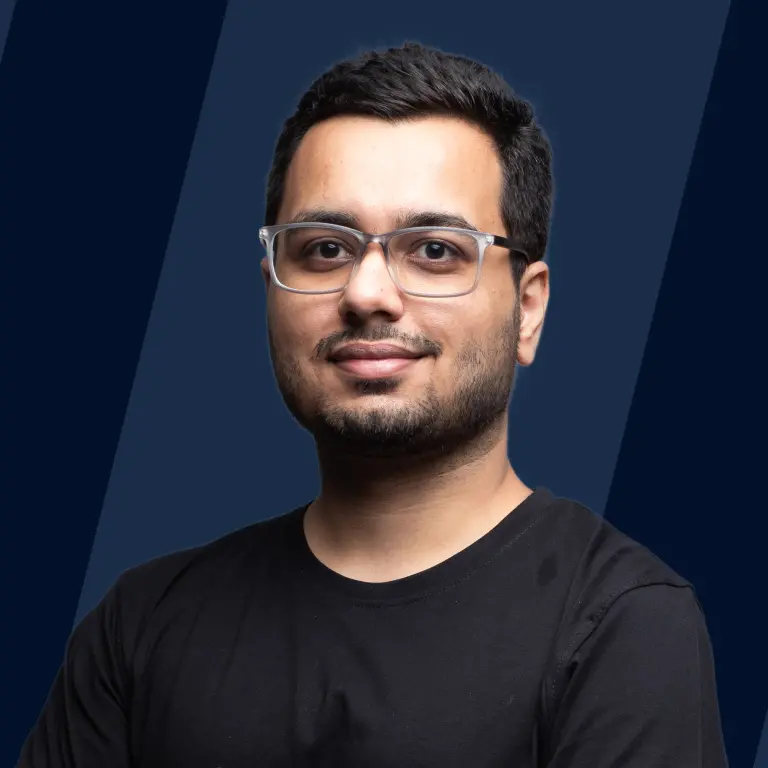
Overview
This article is all about a number-guessing game where we will be asking the player to guess the number between a certain range. If the player guesses it right then Voila! you win otherwise the player gets few more chances to guess if still the player can't guess the right number the player loses the game.
What are we Building?
We will be building a simple project of guessing the number using C++.
- A random number between 1-100 is generated by the computer which is to be guessed by the player.
- We can set difficulty levels for the player where the player can select the level to play like easy, medium, or difficult with a different number of guesses for each level.
- While the player is playing the game the computer tells if the guess is wrong or right, or if the guessed number is less than or greater than the actual number.
- During the game, the player can end the game.
Pre-requisites
To build this program you must have basic knowledge of the following: -
- Loops
- Operators in C++
- Rand() function in C++
- ctime Standard Library
- cstdlib Library
How are we Going to Build the Guessing Game?
The approach used to build the guessing game
STEP 1: - Use rand() function to generate a certain number between 1-100
To generate random numbers we use rand() function which is a part of the cstdlib library.
The syntax to use rand() function: -
In this program, we want to generate a random number between 1-100 so the upperValue is 100 and the lowerValue is 1.
We will also be using the ctime library to use the srand(time(0)) function to generate different random numbers every time the game begins.
Step 2: - Selection of difficulty level by the player
We will set 3 different levels and ask the player to select one level in which the player wants to play.
Step 3: - Set the number of guesses for each difficulty level
We will set 7 chances with easy level, 5 guess chances with medium level and 3 guess chances with difficulty level.
When the player chooses the difficulty level the code remains the same for all the levels but there is a change in the number of leftChance.
Step 4: - Check whether the guessed number is equal to the random number generated
-
Each level has a different number of chances given. Suppose the player chooses a difficult level then 3 chances are granted to the player, where we will iterate from 1 to 3 till the player guesses the actual secret number.
-
If the value guessed by the player is less than the actual number then we will let the player know by printing "Try number greater than the entered number."
-
If the value guessed by the player is greater than the actual number then we will let the player know by printing "Try number less than the guessed number."
-
The player will get chances according to the level the player chooses.
-
The chances get reduced every time the player guesses the wrong number.
-
The game ends when the number of chances is over or the user guesses the right answer.
Final Output
Requirements
These are the following requirements: -
- User should have a code editor for example - VS Code or Sublime.
- The ctime and cstdlib libraries are used.
- Loops and iteration knowledge.
- The functions - srand() and rand() knowledge.
- The user must be aware of the exit function.
Building the Number Guessing Game in C++
STEP 1: - Adding the instructions for the game
First, we will set the instructions for the game for the player.
Output for the above code
STEP 2: - Setting the difficulty levels using while loop
We will set an infinite loop that executes before starting the game, where we will set the difficulty levels for the game.
Output for the above code
STEP 3: - Select the level of difficulty
Output for the above code
Suppose the user enters a string instead of an integer then the program will exit without throwing an error.
STEP 4: - Getting the random number input from the player
To generate different random numbers each time the game is played we use the srand(time(0)) function from the library ctime. We use the rand() function to generate a random number.
STEP 5: - When the player chooses level 1 that is the easy level
STEP 6: - When the player chooses level 2 as medium level
STEP 7: - When the player selects level 3 that is difficult
STEP 8: - When the player chooses to exit the game
What’s Next
There are many approaches to solving this problem. One way is already discussed above.
The other approach can be this way where we take n number of inputs until the player guesses the right number. To do that we will just write a simple program with do while loop.
Output
Try to solve this problem with another approach if you find any!!. For example - Set some amount of inputs from the user removing the difficulty levels.
Conclusion
- Guess the number is a small project game that can be made using any programming language like C++, Python, or Java.
- We used C++ to make the game.
- The rand() and srand() functions from the ctime and cstdlib libraries were used to generate random number and srand(time()) function to generate different random numbers at each execution time.
- To get n number of chances we don't use for loop instead we use do while loop.