Building a Number Guessing Game in Java - Java Projects
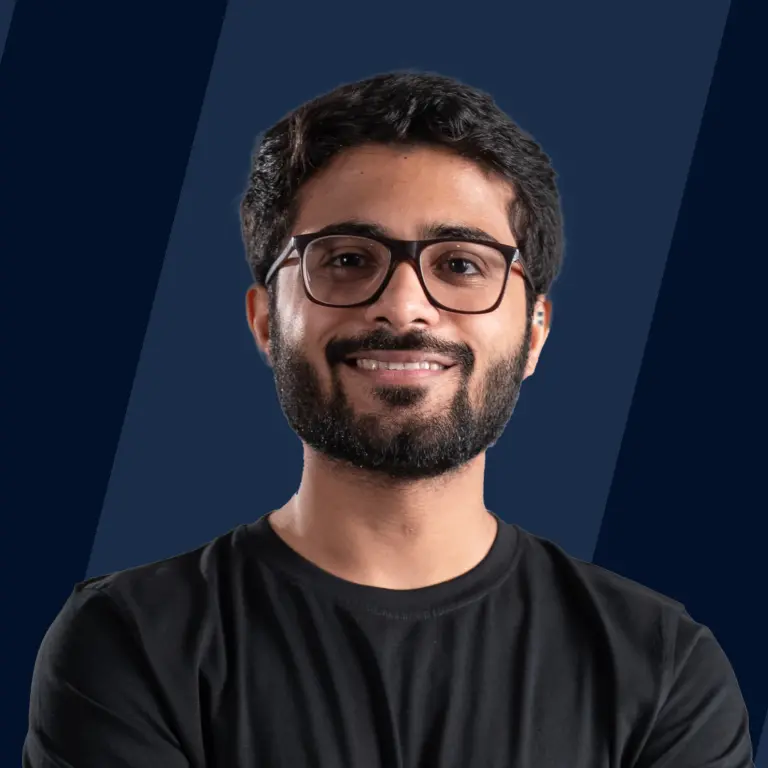
Overview
In this article, we are going to construct Project program named Number Guessing Game in which the user will be provided a range and the user have to guess a number in a limited number of attempts.
What are we Building?
The aim is to create a Java Programm in which a user is given K chances to estimate a randomly generated number. The game's rules are as follows:
-
If the guessed number is more than the real number, the program will display the message "guessed number greater than the actual number."
-
If the guessed number is less than the real number, the program will display a message indicating that the guessed number is less than the actual number.
-
If the guessed number is the same as the real number, or if all K trials have been completed, the program will terminate with an appropriate message.
Pre-Requisites
How Are We Going to Build a Guessing Number Game?
- First we are going to generate a random number using Math. random()
- Then we are going to run a loop K times and each time ask the user to enter an answer.
- If the user entered right then break and print "You won"
- else print "you lost"
Output
Requirements
- Scanner Class: Scanner class in the `java.util package is used to get input of primitive types like int, double, etc., as well as strings. The simplest method to read input in a Java program is this one.
- Math.Random(): java.lang.Math.Random() method is used to generate a random double type number greater than or equal to 0.0 and less than 1.0.
Building the Number Guessing Game
Create a class GuessingGame
Create a class GuessingGame and add the main method in it and import the Scanner and Math class.
Code Explanation In the above code, we have created a Java class in which we are going to write our Game.
Generate a Random Number Between 1 and 100 Using random()
Generate a random number between 1 and 100 using the Math. random() method of the java class.
Code Explanation Math. random() will generate a number between 0 and 1 so we multiply the generated number by 100. This will result in a number between 0 to 99. By adding 1 answer will be in the range of 1-100. A number generated by the random() method is double so the type cast(converted from one data type to another) is into an int. To learn about type casting learn here
Take User Answer For k Times
Now we are going to take input from user k(5) times
Code Explanation In the above code we have created an Object of Scanner class and run a while loop k times and for each time asked to answer the user.
Take Decision based on User Answer
Now we have to decide based on the user's answer whether the user's answer is correct or not.
Code Explanation
If the user guessed the correct then print the correct message and exit. If the user's guess is higher than the answer then the print message guess is high If the user's guess is lower than the answer then the print message guess is low. After each iteration decrease the number of trials.
What's Next
We have created a Number Guessing Game in java but that doesn't look too good. Users can interact better with this. To make it better try to create a GUI interface using Swing in java A Graphical User Interface (GUI) toolkit in Java called Swing contains the GUI components. With the help of Swing, Java applications may create sophisticated GUI components using a wide variety of widgets and packages. The Java Foundation Classes (JFC), an API for Java GUI programming that provides GUI, includes Swing.
The Java Abstract Widget Toolkit (AWT), a more dated, platform-dependent GUI toolkit, is built on top of the Java Swing library. Instead of creating the components from scratch, you can utilise the library's simple GUI programming components like buttons, textboxes, etc.
We can create a GUI Version of the Number Guessing Game in Swing by using various TextField to input numbers, buttons, and labels that allow us to create an interactive GUI.
Creating a GUI version allows users to interact with the Game easily.
Conclusion
- In this article we have created a guessing game in java.
- First we generated a number and then ask the user for answer k times(trials)
- If the guessed number is more than the real number, the program will display the message "guessed number greater than the actual number."
- If the guessed number is less than the real number, the program will display a message indicating that the guessed number is less than the actual number.
- If the guessed number is the same as the real number, or if all K trials have been completed, the program will terminate with an appropriate message.
- To Guess the number we can use a binary search algorithm For example if the number generated is 25 then we can first check if the answer is (0+100/2)=50 as it is higher than then answer then we will move our low to 0 and high to 50 then mid will be 25 which is the answer.