Number Guessing Game Python Project
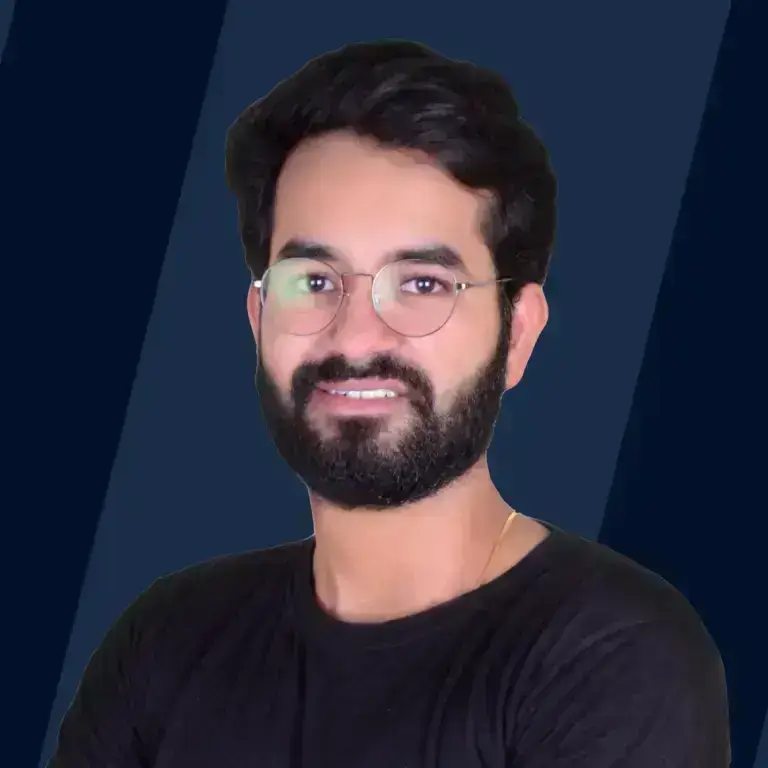
Overview
The number Guessing game in Python is a common project for programmers who have recently learned a new language and mastered topics like number generation and conditional statements with iteration.
What We are Building?
The foundation of the number guessing game in Python is the player's assumption that they can guess a number within the given range. The player wins the game if they correctly guess the target number; else, they lose. The player must specify how many of the game's restricted attempts they have since it has limited efforts. The player will lose the game if this happens.
Pre-requisites
The Pre-requites for Number Guessing Game in Python are listed below with their study links, which can be taken into reference before starting this topic.
How are We Going to Build Number Guessing Game Using Python?
There are a few tasks that we need to take care of while building a number-guessing game using Python. First of all, we need the user to select a range of numbers in which the number-guessing game will be played. Once the user has selected the range from X to Y, where X and Y are integers. Some random number will be selected by the integer and the user has to guess that integer in the minimum number of guesses.
So, How do we find this minimum number of guesses? Well, that minimum number of guesses depends upon range and we already have the formula for it.
Now, we have every possible information required for creating our Number Guessing game in Python. So, let’s understand its Algorithmic flow.
Algorithm
- Step1: The range’s lower and upper bounds are taken as input by the user.
- Step 2: A random integer between the range is generated by the Python compiler and stored in a variable for later use.
- Step 3: An initialized while loop will be used for repeated guessing.
- Step 4: The user receives the message “Try Again! You guessed too high” if the guesses are higher than the randomly chosen number.
- Step 5: Else The user receives the output “Try Again! You guessed too small” if the user’s guess is less than the randomly chosen number.
- Step 6: And if the user correctly predicted the answer in the required number of attempts, they are congratulated.
- Step 7: At the end, the user will receive the output “Better Luck next time” if they failed to correctly guess the integer in the required number of attempts.
Now, since we have familiarized ourselves with a required algorithm. Let’s try to implement our Number Guessing game using Python
Python Code
Output 1:
Output 2:
What’s Next?
Now, since we have successfully implemented our project. What we can do forward is try to add extra features like adding a maximum number of chances a user can get to guess the number. If the user is unable to guess the number within the number of chances, then the user loses.
How, to implement this feature?
We can define a variable for the maximum number of chances that the user gets to guess the missing number. Then, we can check whether the user has chances or not before asking for input and terminate the game if the number of chances is exhausted.
So, try to implement this feature and get ahold of Python.
Conclusion
Although we created a simple number guessing game in Python we got to learn a lot of things while building this project.
- Firstly, we learned about Binary Search Upper and Lower Bound, followed by the use of while loop in Python.
- Finally, we got to brainstorm and as a result, we understood the thought process while building a project from scratch.