Javascript Object.defineProperties()
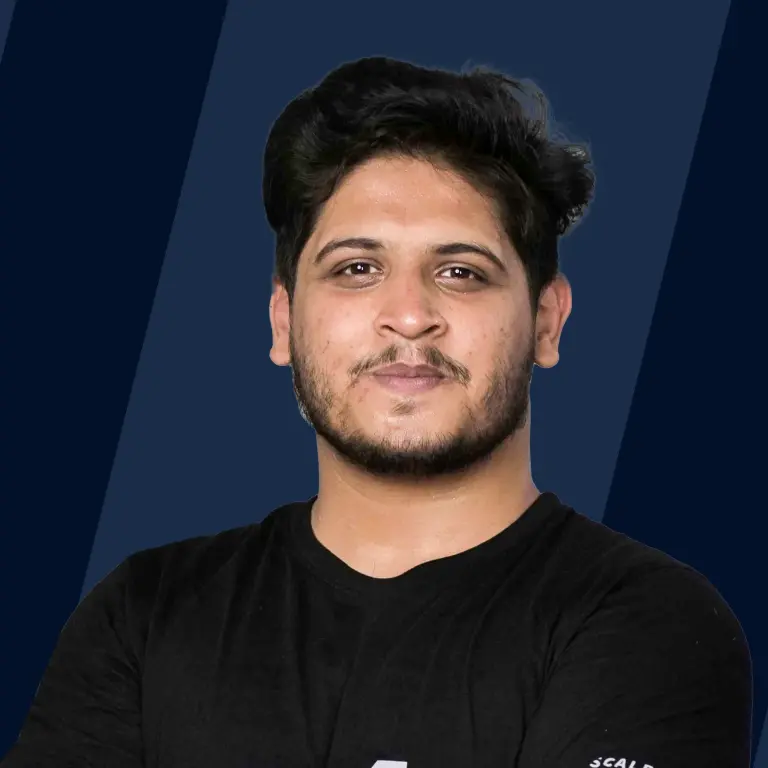
Object.defineProperties() is a method in Javascript that helps us create, add, and modify the properties of an object and returns the object passed to it as a parameter. The static method named defineProperties() gets called using the class Object.
Syntax
The following is the syntax of Object defineProperties():
Parameters
-
Obj: It refers to the object whose properties are to be defined or modified.
-
Props: It represents an object having keys designating the name of the properties to be defined and values that describe those properties. Props values are either a data descriptor or an accessor descriptor having a configurable or enumerable property. The properties are optional. The data descriptors can take two values - value and writable. The accessor descriptor takes the get and sets the value.
- Configurable: This parameter determines whether a property can be redefined or removed via the delete operator.
- Enumerable: This parameter determines if a property gets returned on being accessed using the for_in loop of Javascript. It values to true if this property shows up during the enumeration of the properties on the corresponding object. All the properties created by assignment or initializer are enumerable by default.
- Value: This parameter contains the actual value of a property. The value associated with the property can be any valid JavaScript value that is either a number, object, function, etc. It takes the undefined default value.
- writable: This parameter specifies if the value connected with a property can be changed using an assignment operator or not. Its value can be either true or false.
- get: The get function is a descriptor for the accessor property. It gets called when data is read from the property and has to return a value. The default return value of the get function is undefined. The function's return value is the property's value.
- set: The set function gets called when the accessor property has to be assigned a value. It works as a setter of the property. The new value assigned to the property serves as the only argument of the set function. It takes the undefined default value.
Return Value
Return Type: Object
This method returns an object passed as an argument to the function.
Exceptions for Object defineProperties()
A descriptor gets considered a data descriptor if it has no keys out of value, writable, set, or get. If a descriptor has keys of both data descriptor ( value or writable ) and the accessor descriptor ( set or get ), it throws an exception.
Examples
Example 1
Output:
Explanation: As we can see in the above example, the value of first property is set to 44, and it is printed in the console.
Example 2
Output:
Explanation: In the above example, multiple values are assigned to a property, but it takes only the last assigned value.
Example 3
Output:
Explanation: The above example clearly shows that multiple properties can be defined in a single object.
Example 4
Output
Explanation: The above example shows that if the value is not asigned to a property then it takes undefined as default value.
Example 5
Output:
Explanation: As we can see in the above example, we can set the writable as false.
Example 6
Output:
Explanation: The above example clearly shows that the false writable value does not allow the value to be changed in the object.
Example 7
Output
Explanation: The above example shows how Object defineProperties can be used with array like objects (with and without random key ordering).
Polyfill
In older browsers that do not support our code, polyfill refers to a piece of code that gives a modern functionality to them. Javascript Object.defineProperties can reimplement to an equivalent code in a new execution environment with all names and properties referring to their initial values.
Explanation:
The above code takes two parameters - an object and its properties in the defineProperties function. The function checks if the passed parameter is of the type object. Otherwise, it returns a type error with the message bad obj. The object keys represent the properties that get stored in the descs array. The function convertToDescriptor checks if the descriptor owns the property like configurable, enumerable, writable, and value.
Browser Compatibility
The Object.defineProperties() method in JavaScript is supported by all browsers such as Chrome, Edge, Safari, Opera, etc.
- Also supported in Safari 5, but not on DOM objects.
- In Internet Explorer 8, this was only supported on DOM objects and with some non-standard behaviors. This was later fixed in Internet Explorer 9.
- Also supported in Safari for iOS 4.2, but not on DOM objects.
Conclusion
- Javascript Object.defineProperties() is a method that creates, adds, and modifies the properties on an object and returns the object passed to it as a parameter.
- It has various parameters like obj, props, configurable and enumerable.
- It has two types of descriptors - data descriptor and accessor descriptor.
- The data descriptor can be value or writeable.
- The accessor descriptor can be set or get.
- The polyfill is a code that helps us reimplement Object.defineProperties in Javascript in browsers that don't support it.