Object Keys JavaScript
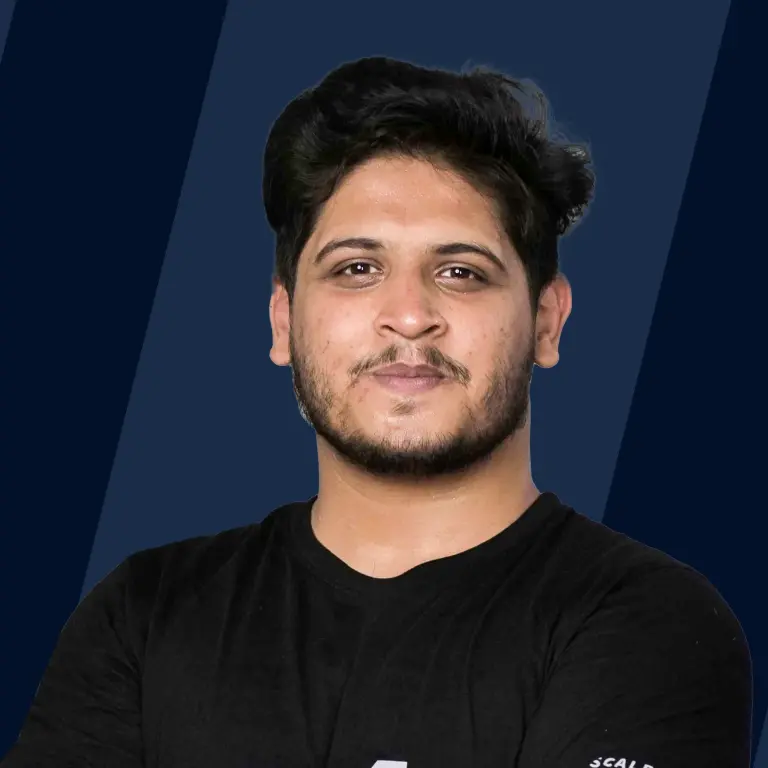
Overview
Javascript is a scripting language used excessively in web user interfaces to be run in a client web browser, on the server-side, and for making even web applications and desktop applications, etc. Objects are an important and useful data structure in Javascript. They store key-value pairs. In this article, we shall discuss the Object keys javascript method.
Syntax of JavaScript Object.keys( ) Function
The following is the syntax for using the Object keys javascript method in Javascript:
Parameters of JavaScript Object.keys( ) Function
The parameter passed to an Object keys javascript method call could be one of:
- array
- object
- non-object argument : it is from ES2015 onwards. It will be coerced to an object.
Return Value of JavaScript Object.keys( ) Function
Return Type: array of strings
A call to the Object.keys() js method returns an array of strings that represent all the enumerable properties of the argument object.
Exceptions of JavaScript Object.keys( ) Function
A call to Object.keys() method in js throws exception
If no argument or null (or null valued variable) is passed as an argument. If using versions prior to ES2015, a typeError exception is thrown if the argument is not of data type Object.
Example
The following is an example of JavaScript Object.keys() Function:
Output:
As you can see, an array of a string is returned that is the array of keys of the above object named obj.
What is JavaScript Object.keys( ) Function
The object data structure in Javascript stores key-value pairs. Unique keys are stored in a js object. Corresponding to each key there is a value. The value could be a number, string, another object, etc. The Object keys javascript function is used for returning an array, the elements of which are strings corresponding to the enumerable properties found directly upon an object. The ordering of these properties is the same as the order obtained from the object manually when a loop is run across the properties. The Object keys javascript method takes as argument an object of which the properties should be returned. The method then returns an array of strings that denote all the properties of the argument object.
Suppose we have an object ‘obj’. We want to obtain a list of all the keys in the object obj. This is where we use the ‘keys’ method of class ‘Object’. Since arrays or all non-primitive data types are also of type Object, hence we make a call to the Object.keys() method passing a parameter of any of these types. As mentioned earlier, from ES2015 onwards, an argument of non-object data type is also considered to be valid. The result (array returned) will be slightly dependent upon the data type of the argument passed, we shall understand this difference in brief later in examples.
Applications of JavaScript Object.keys( ) Function
Object.keys() method serves solely one purpose - returning enumerable properties of an argument. There can arise many scenarios in which one may use Object.keys() js method. We will have a thorough discussion on some of the prominent use cases of the Object.keys() method in js in the Examples section.
More Examples
1. With an argument of object data type
Output:
Explanation:
As shown in the above example, how we can use Object.keys() in javascript with its argument as object data type.
2. With an argument of non-object data type
Output:
Explanation:
As shown in the above example, how we can use Object.keys() in javascript with its argument as non object data type. In this case, the argument passed is of string, number, and boolean data type.
Polyfill
For adding compatible Object.keys support in older environments that do not support it natively, use the following snippet:
In the above code, we try to create a Object.keys() method if it doesn't already exist. Please note that the above code includes non-enumerable keys in IE7 (and maybe IE8) when passing in an object from a different window. For a simple Browser Polyfill, check out the Browser Compatibility section ahead.
Browser Compatability
The Object.keys() function in javascript is supported by all the browsers such as Google Chrome, Microsoft Edge, Internet Explorer, Opera etc.
Conclusion
- Objects are an important and useful data structure in Javascript. They store key-value pairs.
- The Object.keys() function in js is used for returning an array, the elements of which are strings corresponding to the enumerable properties found directly upon an object.
- The argument to Object.keys() method can be of type non-object from ES2015 onwards.
- Object.keys() method serves solely one purpose - returning enumerable properties of an argument. There can arise many scenarios in which one may use the Object.keys() javascript method.