How to Get the Length of an Object in JavaScript?
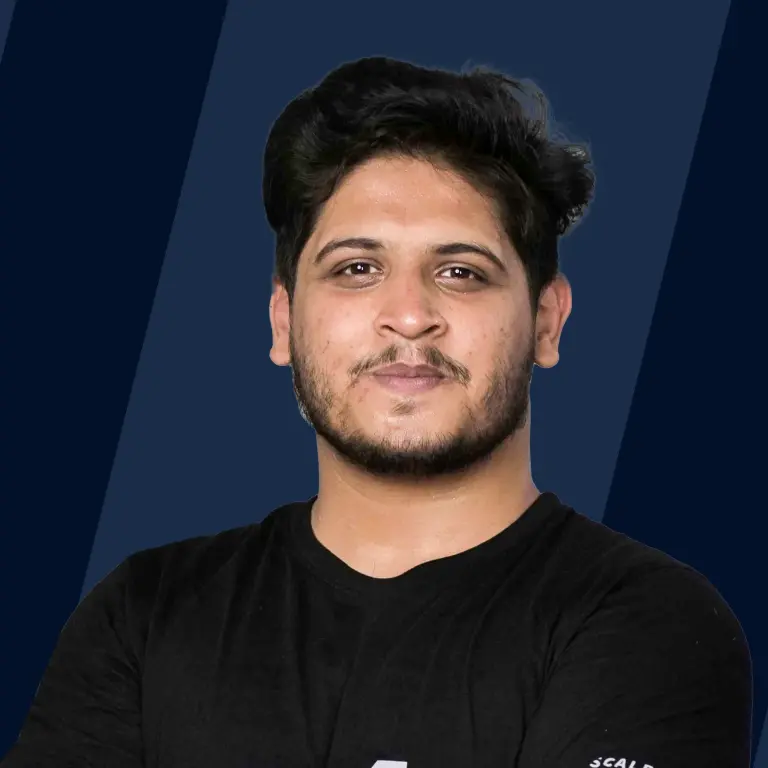
Overview
An object is a collection of key values linked as links between names and values. It is important to understand how to check the length of an object in order to avoid unnecessary bugs. Checking the object length in javascript isn't a common and fundamental operation; however, it is crucial to understand how to do so. There is no default length property on the object. It is only available to arrays and strings that have the length property.
Introduction
Objects are collections of key-value pairs that have properties that are linked by their names. Understanding how to find the object's length is not a common and basic operation, but in order to avoid unnecessary bugs, it is essential to know how it can be done. There is no length property by default on the object. It is only available to arrays and strings that have the length property. A JavaScript object's length can be determined by either using one of its static methods or by using the for...in loop method. Let us explore them one by one
Method 1: Using the Object.keys() Method
An object's length can be obtained by calling the Object.keys() method and accessing the length property, such as Object.keys(obj).length. A given object's key name is returned by Object.keys(); its length is returned by its length property.
Syntax:
Example:
This example shows how we can find the length of an object with three fields using Object.keys().
Output:
Method 2: How to Loop through All the Fields of the Object and Check Their Property
A boolean value is returned by the hasOwnProperty() method indicating whether the specified property belongs to the object. It is possible to check whether each key is present within the object by using this method. Each key in the object is looped through, and if it is present, the total number of keys is increased. In this way, you can find out the object's length from this.
Example:
This example shows how we can loop through the object with three fields using the hasOwnProperty() method. We loop through all the 3 fields inside the user and display it as output using hasOwnProperty() method.
Output:
Get Length of Object with Object.values()
The Object.values() method returns a list of the enumerable property values associated with the object. As a next step, we will use the object's length property to determine how many elements there are in the array (length of the object).
Syntax:
Example:
This example shows how we can find the length of an object with three fields using Object.values().
Output:
Get Length of Object with Object.entries()
The Object.entries() method returns a collection of string-keyed properties [key, value] on your object. We will then use the length property to determine the number of elements.
Syntax:
Example:
This example shows how we can find the length of an object with three fields using Object.entries().
Output:
Get Length of Object Using for…in Loop
In the for...in statement, all enumerable properties of an object, including inherited enumerable properties, are iterated over (ignoring ones keyed by symbols).
Example:
This example shows how we can find the length of an object with three fields using for..in the loop.
Output:
Object.keys vs Object.getOwnPropertyNames
The Object.getOwnPropertyNames() method returns the names of all the properties owned by an object. The Object.keys() method returns all the object's enumerable properties. If you don't set enumerable: false to any property, both return the same result.
The student object has two properties, including its name and age.
Let's define another property named Class but set it to enumerable: false:
In JavaScript, a property is enumerable if it can be read while being iterated using the for...in the loop or the Object. keys() function.
Object.keys do not include the resolution property:
Object Length with Symbols
The symbol primitive data type was introduced in ECMAScript 6. It returns an array of all symbol properties found directly on an object using the getOwnPropertySymbol() method.
Example:
Example:
This example shows how we can find the length of an object with three fields using getOwnPropertySymbol() method.
Output:
Level up your coding game with our JavaScript course. Enroll today and get certified!
Conclusion
- We explored about the various ways we could use to find the length of objects using Object.values(), Object.entries() and by using for..in loop
- The length property of the Object.keys() method is perhaps the simplest and fastest way to obtain the length of an object in JavaScript.
- Both static and for..in loop would do the job for you but most programmers prefer the object static method only
- Finding the length of javascript objects is not as easy as finding the length for arrays it is quite complicated but can be solved by using the methods listed in this article