[object, Object] in JavaScript - How to Fix It?
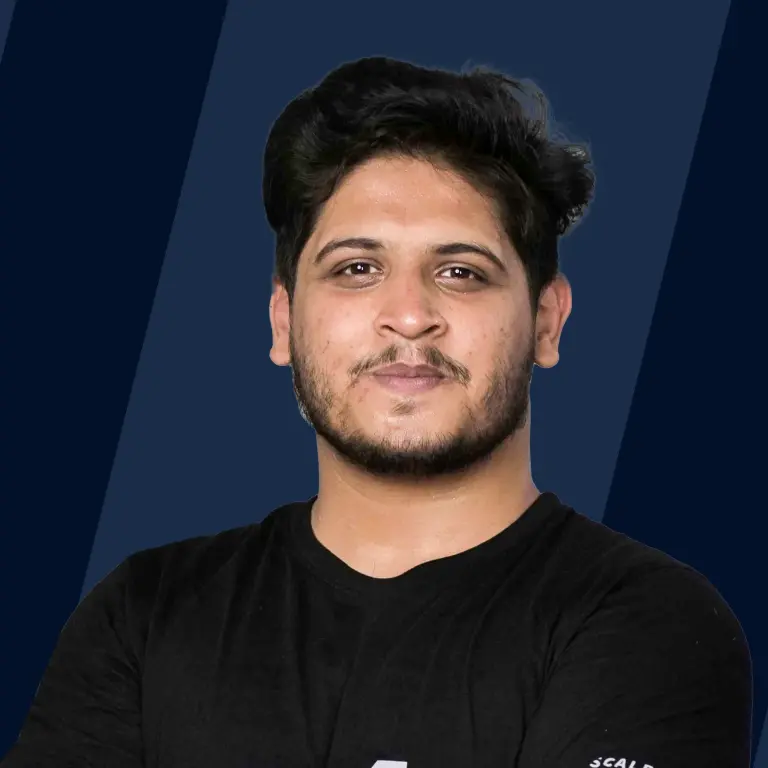
While working in JavaScript, you must have come through some output [object, Object] (generally while using the alert() function on some objects). [object, object] is the string representation of a JavaScript object data type. When we use the alert() function for the object, the default implementation returns [object Object] as output which is nothing but object object javascript.
What Happens When the alert() Method is Used to Display an Object in JavaScript?
Code:
In the code above, we created an object called student. After using the alert() method to display the object in the browser, we got the output below:
From the image above, instead of having the object and its properties displayed, [object, object] was displayed.
This happens because when you use the alert() method to display an object in JavaScript, you get the string format displayed.
What Happens When the toString() Method is Used on an Object in JavaScript?
The toString() method in JavaScript returns the string format of an object. This section will help you understand what happened under the hood in the last section.
When you use the toString() method on an object in JavaScript, you get the string representation – [object, object] – returned.
Code:
As you can see in the code above, we used the toString() method on an object called student: student.toString().
When we logged this to the console, we got [object, object].
Fixing [object, Object] in JavaScript
Method 1: Using JSON.stringify() Method
To fix the [object Object] error in JavaScript using the JSON.stringify() method, you can simply pass the object to the JSON.stringify() function. This will convert the object to a JSON string, which can then be displayed using the alert() method or any other method that can display strings.
Code:
Output:
The JSON.stringify() function takes an object and returns a JSON string that represents the object. The JSON string is a string that contains the properties of the object, as well as their values.
Method 2: Using Custom toString() Method
To fix the [object, Object] error in JavaScript using a custom toString() method, you can override the default toString() method of the object. This will allow you to provide your own implementation for converting the object to a string.
Code:
Output:
When you override the toString() method of an object, it is important to note that the new toString() method will be used whenever the object is converted to a string. This includes when the object is displayed using the alert() method.
Method 3: Using Template Literals
To fix the [object, Object] error in JavaScript using template literals, you can use the ${} expression to interpolate the object's properties into the string.
Code:
Output:
Template literals allow you to create multi-line strings and embed expressions within the string. The ${} expression evaluates the expression and inserts the result into the string.
Conclusion
- The string "[object Object]" in JavaScript is the default string representation of an object. It is returned when you try to print an object to the console without first formatting the object as a string.
- There are two main contexts where you might see the "[object Object]" string:
- When you use the alert() method to display an object.
- When you use the toString() method on an object.
- To fix the "[object Object]" error, you can use one of the following methods:
- Use the JSON.stringify() method to convert the object to a JSON string.
- Override the toString() method of the object to provide your own implementation for converting the object to a string.
- Use template literals to interpolate the object's properties into a string.