Convert Object to String in Java
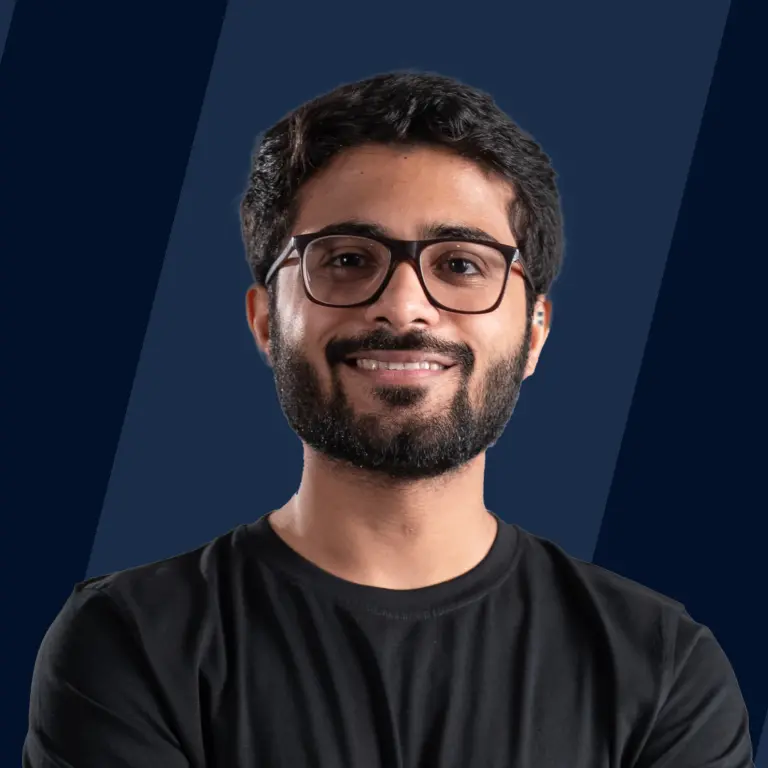
In Java, you can convert an object to a string using the toString() method, which is a method of the Object class. It returns a string that represents the object.
We can also use the String.valueOf() method to convert an object to a string in Java. This method is a static method of the String class that takes an object as a parameter and returns a string that represents the object.
Introduction
The Object class is the superclass of all other classes in Java. It implies that a reference variable of Object type can refer to an instance of any other class. Every class in Java inherits methods of the Object class implicitly.
In Java, you can convert an object to a string using the toString() method, which is a method of the Object class. Similarly, there are other methods in the Object class that can be used for converting an instance to a string as listed below.
Methods | Description | Time Complexity |
---|---|---|
String toString() | Returns the string representation that describes the object. | O(n) |
String valueOf() | This method belongs to a String class and is a static method. | O(n) |
+ operator | It is used for concatenating any type of value with a string and returns the output as a string. | O(1) |
join() | It allows you to an array of objects into a single string. This method is part of the String class and it takes two parameters: a delimiter and an array of objects. | O(n*k) |
Java Program to Convert Object of a User-Defined Class to String Using toString() Method
In this example, we will learn how to convert an object into a string using toString() method.
Output:
Explanation:
- The code above defines a class EmployeeTest that has two instance variables firstName and lastName, along with setter and getter methods to access and modify these variables.
- The class also overrides the toString() method to provide a customized string representation of the EmployeeTest object.
- The main() method creates an EmployeeTest object and calls its getString() method to print its string representation as shown.
Java Program to Convert Object of Predefined Class(StringBuilder) to String Using valueOf() Method
In this example, we will learn how to convert an object to a string using the valueOf method.
Output:
Explanation:
- In this code, the main() method creates an EmployeeTest object and calls the String.valueOf() method to print its string representation as shown.
Java Program to Convert Object to String Using the + Operator in Java
Write a program to covert the object to string using the ‘+’ concatenation operator
Output:
Explanation:
- In this code, the main() method creates an EmployeeTest object and uses the concatenation (+) operator method to print its string representation as shown.
Java Program to Convert Object to String Using the join() Method in Java
The join() method can be used to concatenate the string representations of the objects in an array as shown below.
Output:
Explanation:
- This code creates a list of strings list and adds three string elements to it.
- The join() method of the String class concatenate the elements of the list into a single string with a comma separator.
- The resulting string is then printed to the console using the System.out.println() method.
Conclusion
- Java provides several methods for converting an object to a string, including the toString() method, String.valueOf() method, + operator, and join() method.
- The toString() and String.valueOf() methods are part of the Object and String classes, respectively, and they return a string that represents the object.
- The + operator is used for concatenating any type of value with a string and returns the output as a string.
- Finally, the join() method allows you to join an array of objects into a single string.
- When converting an object to a string, it is essential to consider the time complexity of the method used. The + operator has a time complexity of O(1), while the join() method has a time complexity of O(n*k).