Occurrence of Character in String in Java
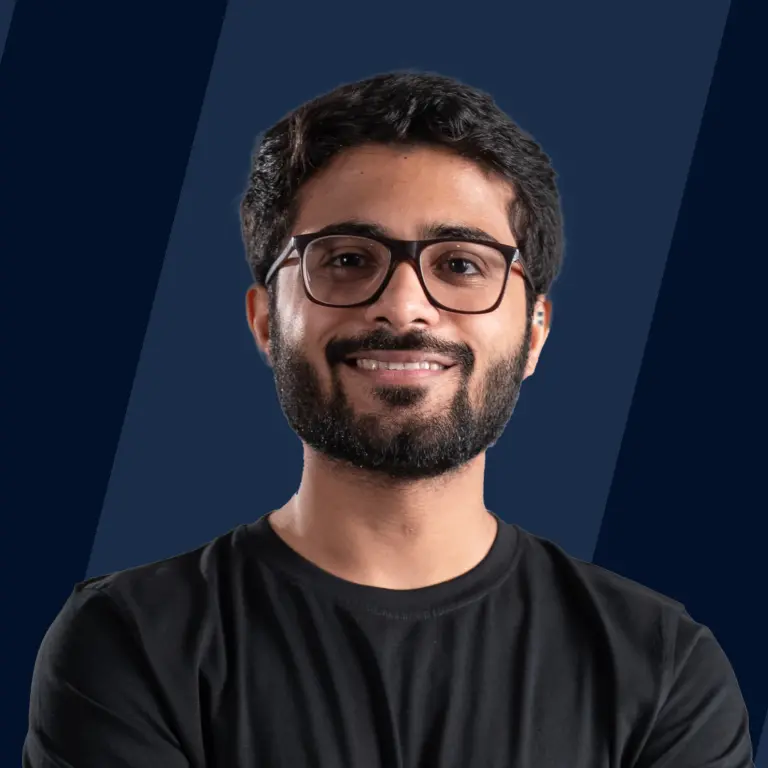
Overview
A String is a sequence of characters. We can find the number of occurrences of a given character in a String using various methods such as:
- Iterative Approach
- Recursive Approach
- Regex Patterns
- External Libraries
Introduction
A String is a sequence of characters. This sequence can have duplicate characters as well. In this blog, we will discuss various methods to count the number of occurrences of a character in a given string.
Let us go through them one by one.
Using Core Java Lib
Iterative Approach
The iterative solution is the most conventional, intuitive and easiest method for counting occurrences of a character in a string.
In this method, we are iteratively traversing each character of the string and comparing that character with the character to search. For this, we are using charAt method (offered by the String Class of java.lang package) that returns the character present at the specified index in the string.
Output
Using Recursion
Recursive solution is not very intuitive, but still a little tricky and an interesting method.
In the code below, we call our recursive method for the index 0 of the string (the first character). Then we compare this character with the character to search. If the character matches, we increment our counter to 1 and then call the function recursively for index+1 (next index). We keep on going until we reach the end of the string.
Output
Using Regular Expressions
A regular expression is a sequence of characters that forms a search pattern. When we search for data in a text, we can use this search pattern to describe what we are searching for. A regular expression can be as simple as a single character or can be a more complicated pattern.
Java doesn't have a built-in Regular Expression class, but we can import the java.util.regex package to work with regular expressions. The Matcher and Pattern classes in this package provide the facility of Java regular expressions.
Another way would be to use regular expressions:
Output
Using Java 8 Features
We can use Java 8 or above JDK Version for a cleaner and more readable solution using the core Java library. We can use the feature of lambdas and Stream for counting occurrences of a character in a string.
Using chars() method of IntStream class
First, we will use the chars() of the IntStream class which will return a stream of characters. Then, we will filter them using the Lambda expression with the search character as an argument of the filter() method. Now, we will count their occurrences using the count() method, which is used to get the count of matched lambda expressions in the filtered stream.
Code:
Output
Using codePoints() method of IntStream class
Similarly, this time we will use the IntStream class method -codePoints(), which returns the integer codepoints (Unicode value) of the character stream. Then again, we will filter them using the Lambda expression with the search character and by using the filter() method. Finally, we will count their occurrences using the count() method.
Code:
Output
Using External Libraries
Using StringUtils
StringUtils is a utility class in Java that offers many String manipulative methods (since java.lang.String class offers a minimal set of String methods). Many libraries have it, but the most common library is Apache commons-lang.
For using StringUtils, we first need to import the corresponding JAR file into our project. This can be done manually or through a dependency management tool like Maven or Gradle.
Maven Environment
Add the following snippet to the <dependencies /> section:
Gradle Environment
We need to ensure that the maven standard repositories are available like this:
Then add the Apache commons dependency in the dependencies section like this:
Import Statement
Next, the commons.lang.StringUtils class offers a countMatches() method. This method is used for counting chars or even sub-strings for a particular String.
We can use countMatches() method to count the number of occurrences of character 'o' in the "www.scaler.com/topics" String.
Output
Using Guava
Guava is an open-source set of common Java-based libraries. It is reliable, fast, efficient and mostly developed by Google. It provides many utility methods for string processing such as Joiner, Splitter, CharMatcher, Charsets, CaseFormat.
We first need to import the JAR file into our project for using Guava. This can be done manually or through a dependency management tool like Maven or Gradle.
Maven set up
Add the following snippet to the <dependencies /> section:
Gradle We need to ensure that the maven standard repositories are available like this:
Then we need to add the Guava dependency in the dependencies section like this:
Next, the Guava offers a CharMatcher class. This class can be used for counting chars for a particular String.
Let's see how we can use CharMatcher to quickly help us count the number of occurrences of character 'o' in the "www.scaler.com/topics" String.
Output
Using Spring
If we have Spring Framework in our project, we can also use org.springframework.util.StringUtils to count occurrences of a character in a string.
org.springframework.util.StringUtils offers countOccurrencesOf method. Let's use countOccurrencesOf for counting the number of occurrences of character 'o' in the "www.scaler.com/topics" String.
Output
Conclusion
- There are many ways to count number of occurrences of a character in a string, some using the core java library and some using external libraries.
- Methods using only core java library discussed in the article are: Iterative Approach, Recursive Approach, Approach with Regular expression, Approach using Java 8 features.
- We can use the chars() and codePoints() methods of IntStream to count the number of occurrences of a given character in a string.
- Methods using external libraries discussed in the article are: using StringUtils, using Guava, using Spring.
- Generally, adding the external libraries into our project just to count characters in a string doesn't make much sense. However, if we already have them in our project, then we can directly use them.