C++ Program for Octal to Decimal Conversion
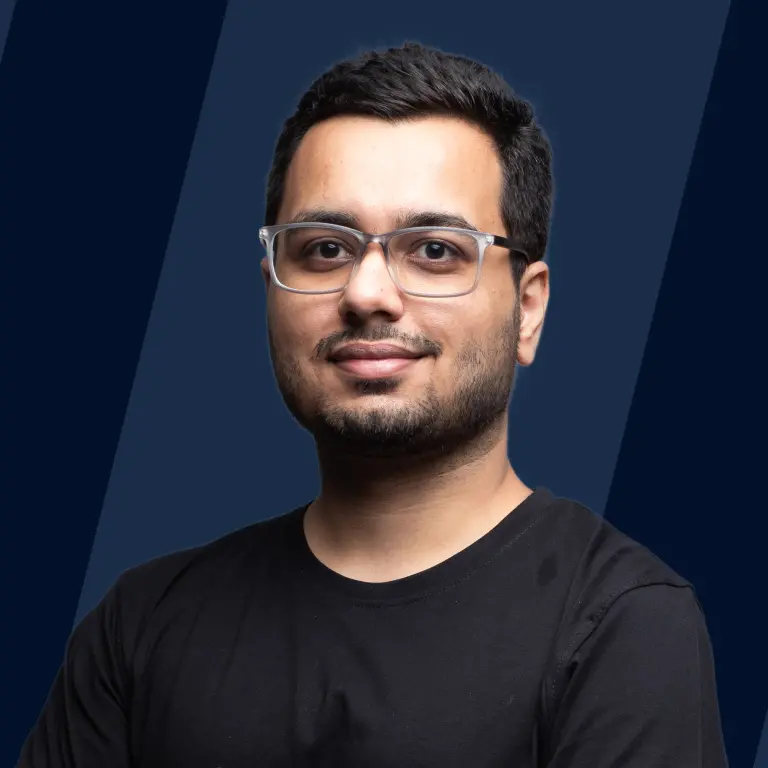
Overview
We need different number systems to meet different-different needs as per the situation, so as it happens, we must also know the various conversion methods to convert a number from one number system to another.
Introduction
The term octal to decimal refers to the process of converting an octal number with the base as 8 to a decimal number with the base as 10. The first question you might ask that why we even need different number systems in the first place.
See, we all know that computer only understands the language of 0s and 1s ie, switch on or switch off, and for us as humans, it can become quite difficult to communicate with the computer using the the binary form of data.
If we talk specifically about numbers, then various number systems were introduced like ternary, octal, decimal, and hexadecimal to ease our task of writing numerical data.
For Example:
You can see, that the same number, when written in binary, is taking 8 digits, while in octal it is taking only 3 digits and in decimal and hexadecimal systems it only takes 2 digits.
This is why the need for different number systems came into existence.
So as different number systems exist, we also must be aware of the conversion methods to convert a number from one number system to another, in this article we will be mainly focusing on octal to decimal conversion.
Working of Octal to Decimal
Let's now understand the working process behind this conversion.
Like any other number conversion set, here also, the base number is used to convert a number from one number system to another, here we multiply each digit of the given number with the power of 8 starting from the right-most digit. Firstly we multiply the rightmost digit of the number with 8 raised to the power 0, then we move a digit left and multiply the second right-most digit with 8 raised to power 1 and we continue this process till we reach to the left-most digit of the number, after that, we add all the individual results and that gives the equivalent decimal number to the given octal number.
Let's have a look at the below picture to have a visual understanding of the working process.
Program for Octal to Decimal Conversion Manually by Creating a User-defined Function
Algorithm
- Step 1 - defining the function
- Step 2 -
Example
Input
Output
Explanation
-
Starting with the main() function, we are declaring a variable num to receive the octal number which we have to convert, and we are taking the user input
-
After that we are calling the octalToDecimal() function by passing the num variable into it and storing the calculated result into the decimalValue variable
-
In the octalToDecimal() function we are declaring a variable temp to temporarily store the value of the passed num variable, so that when we do the computations on the given input, then the actual value should also be kept intact somewhere if we anyhow need it ahead.
-
Then we are initializing the decimalValue variable with 0 in the start, after that, we are initializing one more variable to hold the multiplying base value, which we will multiply to the digits by raising this base to the powers of 8 starting from 0 and hence it is 1 ie. 8^0 = 1.
-
Inside the loop we are capturing the last digit of the given input so that we can multiply it with the base 8 and can get the required value, and then we are truncating the number by one place by dividing it by 10.
-
After that, we are updating the value of the decimalValue variable and the base variable to continue with the next iteration, and after the successful execution of the loop, we are returning the calculated decimal value.
-
And finally, we are printing it.
Program for Octal to Decimal Conversion without Using pow() Function
Example
Input
Output
Explanation
-
Here also, starting with the main() function, we are declaring a variable num to receive the octal number which we have to convert, and we are taking the user input
-
After that we are calling the octalToDecimal() function by passing the num variable into it and storing the calculated result into the decimalValue variable
-
In the octalToDecimal() function we are declaring a variable temp to temporarily store the value of the passed num variable, so that when we do the computations on the given input, then the actual value should also be kept intact somewhere if we anyhow need it ahead.
-
Then we are initializing the decimalValue variable with 0 in the start, after that, we are initializing the exponent variable with the value 0 to raise it to the powers of the base 8.
-
Inside the loop we are capturing the last digit of the given input so that we can multiply it with the base 8 and can get the required value, and then we are truncating the number by one place by dividing it by 10.
-
After that, we are updating the value of the decimalValue variable using the pow() function by raising the base 8 to the power of the variable i and then incrementing the value of the variable i by 1, and after the successful execution of the loop, returning the calculated value.
-
And finally, we are printing it.
Program for Octal to Decimal Conversion Using Algorithmic Way
Algorithm
- Step 1 - defining the function
- Step 2 -
Example
Input
Output
Explanation
-
Here as well, starting with the main() function, we are declaring a variable num to receive the octal number which we have to convert, and we are taking the user input
-
After that we are calling the octalToDecimal() function by passing the num variable into it and storing the calculated result into the decimalValue variable
-
In the octalToDecimal() function we are initializing the decimalValue variable with 0 at the start, after that, we are initializing the exponent variable with the value 0 to raise it to the powers of the base and then we are initializing a base variable with the value as 8.
-
Inside the loop we are capturing the last digit of the given input so that we can multiply it with the base 8 and can get the required value, and then we are truncating the number by one place by dividing it by 10.
-
After that, we are updating the value of the decimalValue variable using the pow() function by raising the base 8 to the power of the variable i and then incrementing the value of the variable i by 1, and after the successful execution of the loop, returning the calculated value.
-
And finally, we are printing it.
Program for Octal to Decimal Conversion Using Inbuilt Function stoi()
Let's firstly understand what the function stoi() is and what is its working, The function stoi() is a standard library function in c++ language which is used to convert a string into an integer value. The function takes three parameters, first being the string which we have to convert, second being the position ie. the index in the string from where we want the function to start parsing it, and third being the base value in which we want the final result to be ie. 2 for binary, 8 for octal, 10 for decimal and 16 for hexadecimal.
The function stoi() is relatively new and was added into the language in its C++11 revision (2011).
Example
Input
Output
Explanation
-
Firstly we are declaring a string variable octalString to receive the octal number, but here instead as an integer, we are receiving the number in the string form, after that, we are initializing the decimal value variable with the value as 0.
-
After that we are calling the stoi() function which is used to convert a string into an integer value, provided a valid numerical string has been passed, we are calling the stoi() function with the parameters as the octalString, the index from where we want the conversion to start, here, in this case, the index 0 and the base in which the number is, here it's an octal number, so the third parameter's value will be 8.
-
And then we are assigning the returned value from the stoi() function call to the decimalValue variable and are printing it.
Program to Deal with Invalid Octal Input
Example
Input:
Output:
Input
Output:
Explanation
-
Starting from the main() function, we are declaring the octalNumber variable and are taking the user input.
-
After that we are calling the octalToDecimal() function and are assigning the returned value to the value variable.
-
In the octalToDecima() function, we are firstly verifying if the entered octal number is a valid octal number or not, by checking each digit's value to be less than 8, if any of the digit's value is found to be 8 or greater than 8, we are returning the function with a value of -1 to denote that the entered input is an invalid octal number.
-
If all digits are from the set of 0-7 then we are initializing the value variable as 0 and the base variable with the value as 1, after that we are using the same loop as we used above in the first example to convert the given octal number into its decimal equivalent, and finally returning the calculated value.
Conclusion
-
We need different Number Systems to meet different needs.
-
The term octal to decimal is a process of converting an octal number with the base 8 to a decimal number with the base 10.
-
We do the conversion by multiplying each digit of the given octal number with the power of 8 starting from the right-most digit, with the first exponent as 0.
-
There are various approaches that we can use to convert any given octal number to its decimal equivalent.
-
We can also use the inbuilt function stoi() to easily convert any given number into any other number system, as per the requirement.