What is Ordered Dictionary in Python?
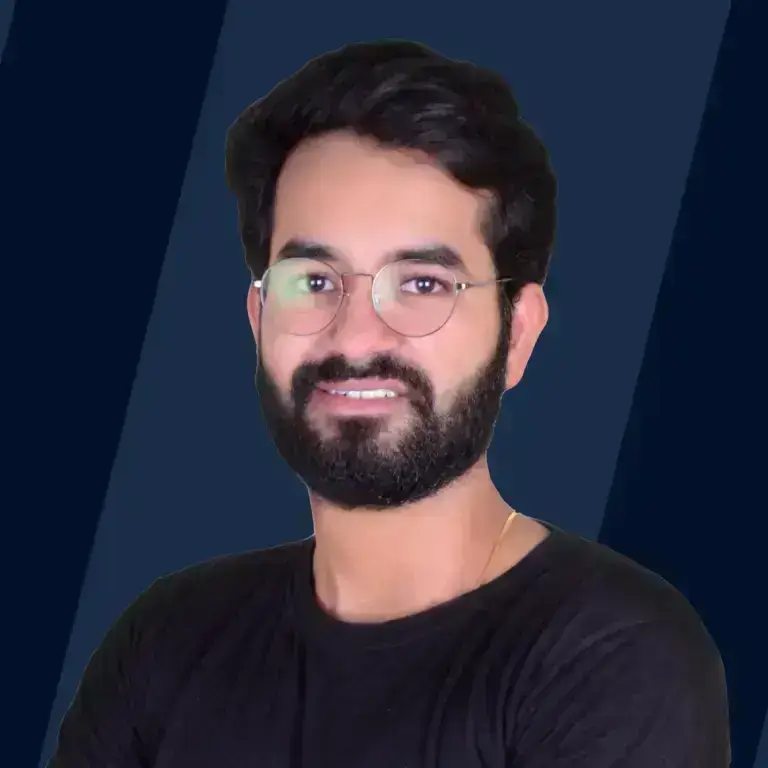
Before we understand what is an ordered dictionary in python, let us first recap a dictionary in python. A dictionary is a data structure in Python that is used to store keys and their corresponding values. Every item in the dictionary is a <k,v> pair where k is the key and v is the value. Her key k is unique and Python does not allow repetition of keys. However, the values can be repeated.
For example, consider the database of a departmental store, that wishes to store the prices of their products
A dictionary is essentially like a map, where each record in the dictionary is called an item
However, dictionaries do not remember the order of insertion of items. In the above example, we do not know the exact order in which items are stored in the dictionary. Let us try to print the items:
Output
The order of items in the output can be different than the order in which elements were stored. This is because items are stored by the hash of the keys
However, we would like to control the order of items, for various use cases some of which we shall see later in this article. For such use cases, Python provides us with ordered dictionary
Syntax of OrderedDict
The syntax of OrderedDict is the same as a regular dictionary. You need to import it first using:
To initialize the dict we use a different constructor:
All other operations are same as a regular dict
Python Program to Demonstrate Working of OrderedDict
Let us study the following code snippet to understand how an OrderedDict works
Output
We can also use a constructor with arguments to create an OrderedDict
OrderedDict has a method called fromKeys() to generate an OrderedDict from a list of keys
Key Points
Mutation operations
OrderedDict is a mutable data structure, hence we can perform the following operations on it:
- Insert
- Update
- Delete
Insertion of an item takes place at the end of the dictionary
If an item is deleted and re-inserted again, it shall be done at the end of the dictionary
Modifying the value of a key does it in-place, this means the position of the key is not affected
We can use update() method to update the value of a key as well. update() is also an in-place method
Iterating through an OrderedDict
For iteration we visit every key and print its value. In order to achieve this we need to write a loop; we can loop over the keys, or the values, or the <key,value> as a pair. Iteration remains the same as in a regular dictionary, just that the items are iterated in the order they were added to the OrderedDict
Output
In all the above cases the output is the same:
Output
Special features in OrderedDict
There are few special methods present in OrderedDict that are not present in a regular dictionary
The method move_to_end() can be used to shift an item at the end or at the start of a dictionary
Syntax
Example
Output
Similarly, bypassing the keyword last we can shift an item to the start
Output
The normal dictionary has a method popitem() to remove the item at the rightmost end of the dictionary. Thus popitem() empties the dictionary in a LIFO order (Last In First Out)
However OrderedDict allows passing a special keyword last that defaults to True. If we set it to False, then the leftmost item in the OrderedDict is popped. Thus a LIFO order of removal of items converts to a FIFO order (First in First Out)
Output
If we perform popitem on an empty dictionary, we shall get a KeyError
Choosing Between OrderedDict and dict
After studying an OrderedDict, the natural question that might be coming to your mind is when shall we use an OrderedDict over a regular Dict? Doesn't it make sense since an OrderedDict preserves the order of items, we should always use OrderedDict?
OrderedDict maintains the order of items inserted in the dictionary, however, which comes with a cost
- OrderedDict occupies 50% more memory to store items. An extra storage layer is required to preserve the ordering of items
- Iteration over an OrderedDict is 50% more costly than a regular dictionary. This is due to the way items are stored in an OrderedDict to achieve guarantee of order. We need to perform an extra hop on the additional data structure explained in the above point
Thus if the order of elements is not mandatory, and we need fast access to (key, value) pairs, we should go with a regular dictionary
If we want the order of elements to be preserved, for example
- If we want the items to be stored in chronological order (LIFO)
- If we wish to access items using an index, we need to deterministically know the position of an item. This is for use cases where we want to store the position of an item using an int for example, as a reference to the key
- If comparison between two dictionaries takes into account the order of sequence of items. Two regular dictionaries having the same items may not be called equal if the ordering of items is important
The following table summarizes the comparison between two types of dictionaries
Feature | OrderedDict | dict |
---|---|---|
Preserving order of items | Yes | No |
Time complexity of operations | High | Low |
Memory footprint | High | Low |
Equality comparison consider order of items | Yes | No |
To know more about Dictionary in Python you can visit this article
Conclusion
- Python has a subclass of dict called OrderedDict that is used to store (key,value) pairs in order
- The order in which items are stored is the order of insertion of items
- Insertion of items happens at the end of the OrderedDict
- If an item is deleted the sequence of the remaining items is maintained
- If an item is updated the update happens in place
- OrderedDict have higher time complexity of operations and higher memory footprint as compared to regular dicts