Python os.listdir() Method
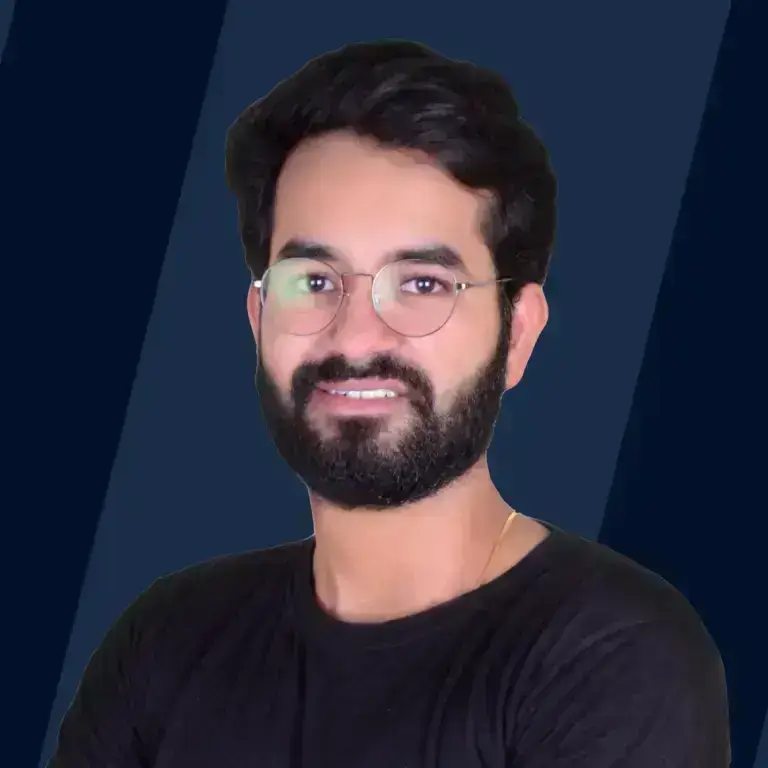
Overview
The Python os.listdir() method is a powerful tool that can retrieve a list of files and directories within a specified path. This method belongs to the os module. It offers a convenient means to interact with the underlying operating system, making it an essential tool for file management tasks in Python programming.
Syntax of Python os.listdir() Method
The syntax for the os.listdir() method is as follows:
We must import the' os' module to use the os.listdir() method in Python. This module is part of the Python standard library and provides a suite of functions for interacting with the operating system.
Parameters of Python os.listdir() Method
The os.listdir() method takes a single parameter:
- path (str): This parameter specifies the location in the file system from which you want to obtain the list of files and directories. It can be an absolute path (a complete path from the root directory) or a relative path (specified about the current working directory).
Return Value of Python os.listdir() Method
The method returns a list containing the names of the files and directories in the specified path.
Exceptions of Python os.listdir() Method
Exception | Description | Common Causes |
---|---|---|
OSError | Generic exception for I/O operations and file system-related errors. This can occur due to permission issues, missing files or directories, or unforeseen errors. | Insufficient permissions to access the specified path, Invalid or non-existent path, Hardware or system-related issues. |
TypeError | Raised when an object of inappropriate type is passed as an argument. In the context of os.listdir(), this occurs if the path argument is not a valid string. | Providing a non-string type (e.g., a list, integer) as the path. |
PermissionError | Specific instance of OSError related to permission issues. It occurs when the user lacks the necessary permissions to access the specified path. | Insufficient permissions to read the contents of the directory. |
How Does the Python os.listdir() Method Work?
Here are the steps showing the working of the os.listdir() method:
-
Path Specification:
- os.listdir() requires a file system path as input.
- This can be either an absolute path or a relative path.
-
Operating System Interaction:
- When os.listdir() is called, it sends a request to the underlying operating system.
- This request asks for a list of all files and directories at the specified path.
-
System-Level Retrieval:
- The operating system locates and accesses the specified directory in the file system.
- It then compiles a list of all the items in that directory.
-
List Compilation:
- The operating system returns this list of items to the Python program.
-
Return Value:
- os.listdir() then takes this list and returns it as the output of the method.
- The returned value is a Python list containing the names of all files and directories within the specified path.
- The list provided by os.listdir() consists only of the names of the files and directories.
- It doesn't include any additional information like file sizes, creation dates, or file types.
Note: The python os.listdir() method's behavior is consistent across different operating systems (Windows, macOS, Linux), making it a reliable tool for cross-platform development.
Examples
Let's consider a scenario with a directory named my_directory that contains the following files and subdirectories:
Let's explore a few examples to demonstrate the usage of the os.listdir() method
Example 1: List Files and Directories in the Current Directory
This example uses os.getcwd() to get the current working directory and then applies os.listdir() to list all files and directories in that location. The file and directory names are returned by os.listdir() in random order and this order can vary between operating systems.
You can use the Python sorted() method to list items in alphabetical or specific order.
Output:
Example 2: List Files in a Specific Directory
In this case, a specific directory path is provided, and os.listdir() is used to retrieve the names of files and subdirectories within that directory.
Output:
Example 3: List Files in a Subdirectory
By providing the path to a subdirectory, os.listdir() gives a list containing the files' names and subdirectories within that specific subdirectory.
Output:
Example 4: Using Relative Paths
This example changes the current directory to the target directory, then uses os.listdir('.') to list the contents. This demonstrates the use of relative paths.
Output:
Example 5: Using a Loop
By looping through the contents of a directory and checking whether each item is a file or directory, this example categorizes and prints them.
Output:
Conclusion
- The Python os.listdir() method is a part of the os module, used for listing files and directories in a specified path.
- It takes a path parameter, an absolute or relative path, and returns a list containing the names of files and directories at the specified location.
- Common exceptions associated with os.listdir() include OSError, TypeError, and PermissionError.
- The method works by interacting with the operating system, retrieving a list of items, and including only names in the specified directory.
See Also
The os module in Python provides a wide range of functions for interacting with the file and operating systems. Some related methods that you might find useful include:
- os.path.join(): For constructing file paths in a platform-independent manner.
- os.mkdir(): Used for creating directories.
- os.remove(): Deletes a file.
- os.rename(): Renames a file.
You can learn more about these methods in OS module in Python