Python os.path.join() Method
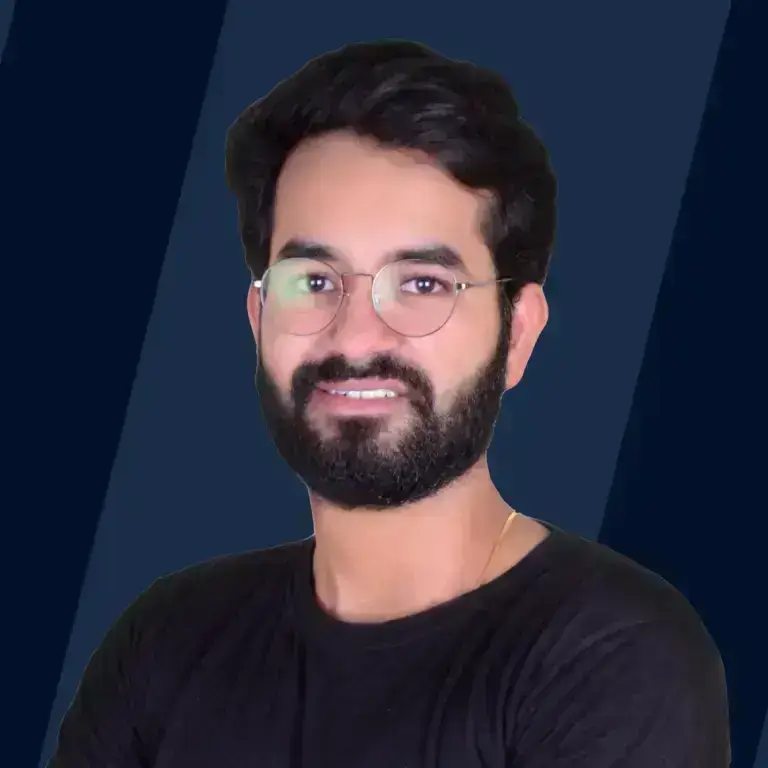
Overview
os.path.join() is a function provided by the Python os module, which works with operating system-related tasks. This function is specifically used to create a valid path by joining one or more directory or file names together using the appropriate separator based on the underlying operating system (i.e., "/" on Unix-like systems and "\" on Windows).
Getting Started with Python's os.path.join() Method
Syntax of os.path.join()
The format for utilizing os.path.join() is structured as follows:
- path:
Serving as the initial segment of the address for a file or directory, this component typically takes the form of a string containing characters, digits, or symbols. Alternatively, it can consist of other data types mimicking string behavior. - *paths:
These encompass any supplementary sections of the address for the file or directory that you wish to append to the initial part. The inclusion of multiple segments is feasible. Similar to the initial part, these sections can adopt the structure of strings or data types resembling strings.
Before diving into the specifics of os.path.join(), let's take a glance at the broader scope of the os module and its offerings.
Methods Available in the OS Module
The os module is a versatile toolkit, offering various functions to perform various tasks, ranging from managing files and directories to working with processes and environment variables. It bridges the gap between Python programs and the underlying operating system, making it an essential asset for system-related operations.
Here's a table of major methods available in the Python os module:
Method | Description |
---|---|
os.name | Provides the name of the operating system as a string. |
os.getcwd() | Returns the current working directory as a string. |
os.chdir(path) | Changes the current working directory to the given path. |
os.listdir(path) | Returns a list of filenames in the given directory. |
os.mkdir(path) | Creates a new directory at the specified path. |
os.makedirs(path) | Creates intermediate directories along with the final directory. |
os.remove(path) | Deletes a file at the specified path. |
os.rmdir(path) | Removes an empty directory at the specified path. |
os.removedirs(path) | Removes intermediate directories if they are empty along the given path. |
os.rename(src, dst) | Renames a file or directory from the source path to the destination path. |
os.stat(path) | Returns information about a file or directory as an object with various attributes. |
os.walk(top) | Generates the file names in a directory tree by walking through the directory and its subdirectories. |
os.path.join(path, *paths) | Joins specified paths using the appropriate separator to form a valid path. |
os.path.exists(path) | Checks if a path exists. |
os.path.isdir(path) | Checks if the given path is a directory. |
os.path.isfile(path) | Checks if the given path is a file. |
os.path.abspath(path) | Returns the absolute version of a path. |
os.path.basename(path) | Returns the base name (last component) of a path. |
os.path.dirname(path) | Returns the directory component of a path. |
os.path.split(path) | Splits a path into its directory and filename components. |
os.path.splitext(path) | Splits a path into its base name and extension components. |
os.path.getsize(path) | Returns the size of a file in bytes. |
os.path.isabs(path) | Checks if a path is an absolute path. |
os.path.join(path, *paths) | Joins specified paths using the appropriate separator to form a valid path. |
os.path.normpath(path) | Normalizes a path, eliminating double slashes and resolving ".." and "." components. |
os.environ | A dictionary containing the user's environment variables. |
os.getenv(var_name) | Retrieves the value of the specified environment variable. |
os.putenv(var_name, value) | Sets the value of an environment variable. |
os.system(command) | Executes the given shell command in a subshell. |
Note:
The os module's behavior can vary depending on the operating system you're using (Windows, Linux, macOS, etc.).
Python's os.path.join() Method
One notable sub-module within the os module is os.path. This sub-module specializes in providing tools for manipulating and working with file and directory paths. The os.path.join() method, in particular, is a standout feature of this sub-module. It's designed to construct valid paths by joining directory and file names while taking into account the appropriate path separator based on the operating system.
Examples of Python's os.path.join() Method with Absolute Paths
Let's dive into some examples to showcase the capabilities of os.path.join().
Example - 1: Joining an Absolute Path with a Directory and a File
Objective:
Combining an absolute path, a directory name, and a file name to form a complete file path.
Output:
Explanation of the example:
In this example, we begin with an absolute path (/home/user), add a directory name (documents), and finally append a file name (report.txt) using the os.path.join() method. This results in the creation of a valid and platform-appropriate file path.
Example - 2: Providing Absolute Path as a Second Argument
Objective:
Demonstrating the usage of os.path.join() with a directory name and an absolute path as its second argument.
Output:
Explanation of the example:
Here, we provide a directory name (downloads) and include an absolute path (/usr/local) as the second argument to os.path.join(). The method skillfully joins these components, leading to the formation of the desired file path.
Example - 3: Absolute Path as the Last Argument
Objective:
Illustrating how os.path.join() handles an absolute path provided as the last argument.
Output:
Explanation of the example:
In this example, we start with a directory name (photos), add a file name (vacation.jpg), and finally include an absolute path (/mnt/drive) as the last argument. os.path.join() adeptly combines these elements, resulting in a combined path structure.
Example - 4: Passing an Empty String
Objective:
Demonstrating how os.path.join() handles empty strings as arguments.
Output:
Explanation of the example:
In this example, we provide an empty string at the beginning, middle, and end of the arguments to os.path.join(). The method effectively ignores the empty strings while constructing the path, leading to the creation of a valid file path.
Example - 5: Using os.path.join() with an Empty List
Objective:
Demonstrating how to use os.path.join() with an empty list of path components.
Output:
Explanation of the example:
In this example, we utilize os.path.join() with an empty list containing path components. By unpacking the list using *, the method assembles the components into a cohesive path, resulting in a valid file path structure.
How to Utilize the os.path.join() Method for Listing Directories?
Beyond path construction, os.path.join() can be immensely useful for listing directories and files within them. By combining os.path.join() with other functions provided by the os module, you can traverse and manipulate directory structures with ease.
Example
Here's an example that demonstrates how to use the os.path.join() method along with other functions from the os module to list directories and files within them:
Code:
Explanation of the code:
In this example, the list_files_in_directory() function takes a directory path as input and uses os.walk() to traverse through the directory and its subdirectories. For each file encountered, it uses os.path.join() to create the complete file path, combining the current root directory with the file name. The print() statement then displays each file's full path.
Conclusion
- Python's os.path.join() method streamlines path construction, adapting to platform-specific variations seamlessly.
- This method enhances code robustness, enabling smooth interaction with the file system across diverse operating systems.
- os.path.join() is a valuable asset for managing file paths and directory structures in Python programming.
- os.path.join() ensures consistent paths across different operating systems, promoting code portability and reliability.
- By abstracting path construction complexities, the method enhances code clarity and reduces errors.
- Utilizing os.path.join() minimizes path-related discrepancies when collaborating on projects or sharing code.
- The method guards against path-related vulnerabilities and errors, bolstering code robustness.
- Embracing os.path.join() future-proofs code by accommodating evolving OS environments and platforms.
- Many Python libraries and frameworks integrate os.path.join(), promoting consistency and compatibility when working with external codebases.
- Embracing os.path.join() aligns with Pythonic coding standards, contributing to a cleaner and more idiomatic codebase.
- The method facilitates seamless collaboration by eliminating path discrepancies and reducing the need for manual adjustments.