What is the Difference Between Overloading and Overriding in Python?
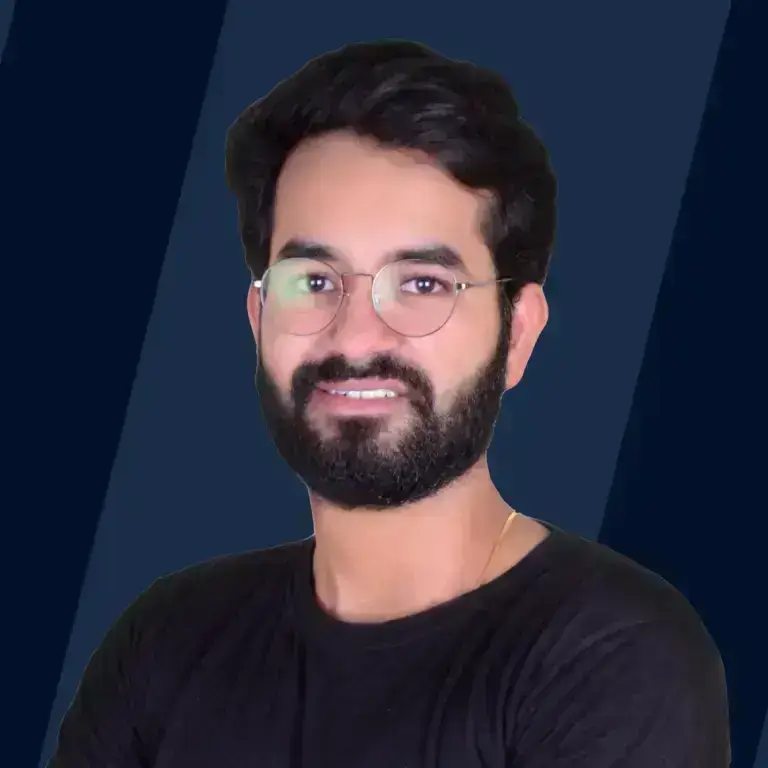
Overloading and Overriding in Python are the two main object-oriented concepts that allow programmers to write methods that can process a variety of different types of functionalities with the same name. This helps us to implement Polymorphism and achieve consistency in our code. Let's look at an example to understand the concept of overloading and overriding:
Consider a scenario where you must select arrows for shooting practice in archery. Here, the concept of overloading and overriding can be depicted as follows:
Consider a case where you have 3 similar-looking arrows with different functionalities or properties. Now, depending on the condition of shooting, the arrow is selected. This is known as overloading. Hence, overloading implies using numerous methods with the same name but accepting a different number of arguments.
Now, consider another case where your Dad has an arrow. You have access to this arrow, as you inherited it. But, you also bought the same type of arrow for yourself. Now, while shooting if you are choosing your arrow over your Dad's arrow, then it is known as overriding.
Now, let's look at overloading and overriding in Python from a technical point of view.
Method Overloading in Python
Method Overloading in Python is a type of Compile-time Polymorphism using which we can define two or more methods in the same class with the same name but with a different parameter list.
We cannot perform method overloading in the Python programming language as everything is considered an object in Python. Out of all the definitions with the same name, it uses the latest definition for the method. Hence, we can define numerous methods with the same name, but we can only use the latest defined method.
In Python, we can make our code have the same features as overloaded functions by defining a method in such a way that there exists more than one way to call it. For example:
Code:
Output:
Method Overriding in Python
Method Overriding is a type of Run-time Polymorphism. A child class method overrides (or provides its implementation) the parent class method of the same name, parameters, and return type. It is used to over-write (redefine) a parent class method in the derived class. Let's look at an example:
Code:
Output:
Diiference Between Overloading and Overriding in Python
Method Overloading | Method Overriding |
---|---|
Compile-time Polymorphism. | Run-time Polymorphism. |
Occurs in the same class. | Occurs in two classes via inheritance. |
Methods must have the same name and different parameters. | Methods must have the same name and same parameters. |
Parameters must differ in number, type, or both. | Parameters and return types must be the same. |
Return type can be different. | Return type must be the same or a subtype. |
Improves code readability. | Achieves runtime polymorphism and dynamic dispatch. |
Can occur within the same class or its subclasses. | Occurs in a subclass that inherits from a superclass. |
Allows same method name for similar operations. | Redefines a method in the subclass with a specific implementation. |
Determined at compile-time. | Determined at runtime. |
Multiple methods can have the same name with different parameters in the same class or its subclasses. | Provides a specific implementation of a method defined in the superclass in its subclass. |
Method overloading is used for creating methods with similar functionalities but different input parameters. | Method overriding is used for providing a specific implementation of a method in a subclass. |
The choice of which method to invoke is made at compile-time based on the method signature. | The choice of which method to invoke is made at runtime based on the object’s type. |
Method overloading is less related to inheritance and polymorphism. | Method overriding is a fundamental concept in object-oriented programming and is closely related to inheritance and polymorphism. |
Conclusion
- Overloading and overriding in Python are the two main concepts of Polymorphism.
- These help us achieve consistency in our code.
- Method Overloading is defining two or more methods with the same name but different parameters.
- Python does not support method overloading.
- Method Overriding is redefining a parent class method in the derived class.
- Overriding requires inheritance for implementation.