How to Implement Pagination in JavaScript?
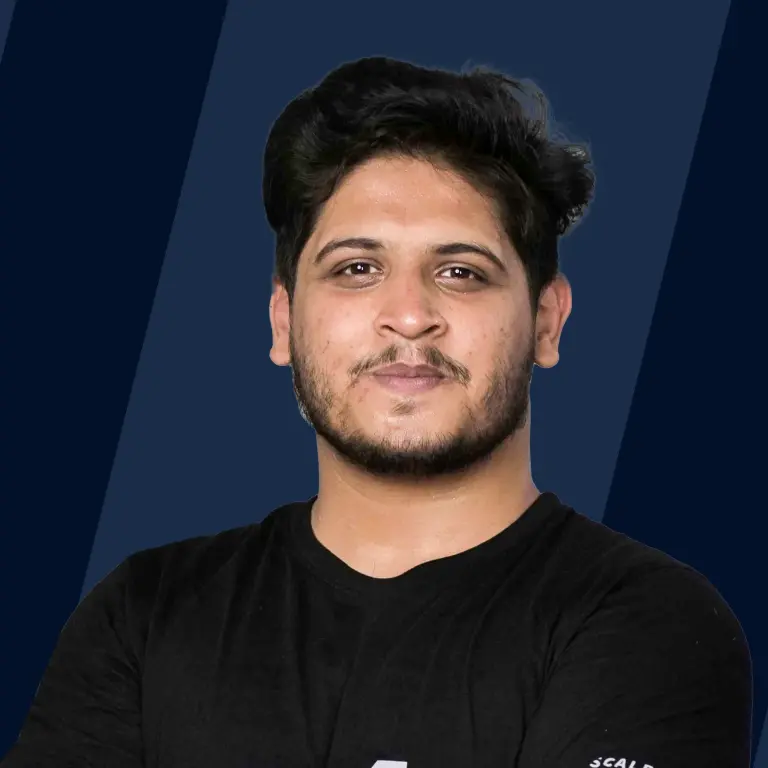
Overview
On a website, pagination divides a block of content into easily readable pages. It helps load data from a server and makes information simpler to see. You can choose only to load a specific amount of items each time the user chooses to view them by using pagination. This reduces the amount of information on a page and saves time.
What is Pagination in JavaScript?
First, Next, Previous, and Last buttons or links are used to navigate between pages when using the JavaScript pagination concept. The fundamental goal of pagination is to enable quick navigation through the information by means of links or buttons. Multiple links or buttons are available in the pagination to access the First, Next, Previous, and Last content. We used various JavaScript functions to create the First, Next, Previous, and Last buttons.
Need of Pagination
Real-Time Scenario:
Consider Amazon or Flipkart's websites for displaying products currently available in their databases. Let's say they have a million items available. The customer will have to wait longer, perhaps a day, to see all the product lists if they try to display all the goods at once.
Implementation of Pagination in JavaScript
Place the Content with HTML
In this demonstration, we'll use HTML to place the text that will be paginated. We'll also look at how the pagination logic may be applied to this while we're building our JavaScript code because in a real-world scenario, we might be getting our content as JSON from a server.
Our paginated content is first defined as a list:
We use the title attribute to display information in a tooltip and provide visual context for the button. The aria-label attribute is used by screen readers.
The pagination-numbers div is now empty because JavaScript will be used to calculate the page numbers.
CSS Styling
For this instance, we'll keep things simple by just styling the pagination numbers and the container.
Set an active class to indicate which page number is presently selected, position the pagination container at the bottom of the page, and override the pagination number's default button styling:
We’ve also added some simple
JavaScript Logic for Pagination
Now to make it work. Let’s define what we’re trying to achieve with pagination:
Only show a limited number of things per page Depending on how often the total items are divided, display page numbers. Change the display to the selected page when a page number is clicked. Permit access to the previous and next pages. First, gather all the materials we'll require:
We will then specify our global variables.
pageCount:
how many pages there will be based on the paginationLimit; and paginationLimit: how many items we want displayed on each page.
currentPage:
keep the currentPage's value.
By dividing the total number of items (listItems.length) by the paginationLimit and rounding to the nearest whole number with the Math.ceil function, you can determine the pageCount.
The Math.ceil() function always rounds a number up to the next largest integer.
Therefore, our page count will be 50/10 = 5 pages if we have 50 items and only want to display 10 items each page. The page count will be 55/10 = 5.5, which rounds up to 6 pages, if we have 55 items and want to display 10 of them each page.
Add Page Number
We can construct a method to create a new button for the page number now that we know how many pages we'll need, and then add the buttons to the paginationNumbers container.
And then we’ll call the getPaginationNumbers function when the web page loads using the window.load() event:
Display Active Pages
We want to develop a function that limits the number of items displayed on each page to the number specified by the paginationLimit. Here's how we'll put it into action:
Set the pageNum value as the currentPage variable's value:
Get the item range that will be displayed. According to the paginationLimit, we want to display items 1 through 10 if we are on page 1. Items 11 through 20 and so on should be displayed if we are on page 2.
Hide every item in the list of things to display in a loop. Then, display everything that is within the range.
Because we are using arrays, item 1 will be at position 0, and item 10 will be at position 9, our logic will be as follows:
We use this technique since our implementation merely covers HTML content. We could revise the logic to read something like this if we were attempting to attach content from a JSON object to the DOM:
Update the window.load() event to set the current page as page 1 once the webpage loads:
Adding Event Listeners
When a page number button is hit, use a click event listener to cause the setCurrentPage function to be called. Use the window to place this function. Consequently, the function now looks like:
Set Active Page Numbers
Additionally, we need to define a method that appends the current class to the page number that was just selected. By deleting the active class, all buttons on the page are reset. The active class is then added to that button if its page index matches the value of the global variable currentPage.
Include this function in the setCurrentPage function so the active page number is updated every time a new page is set:
Create Next and Previous Buttons
Let's add the next and previous buttons to this capability. The logic can be updated while still using the same implementation in the setCurrentPage() function.
Using setCurrentPage(currentPage - 1), the preceding button's current page is changed. To modify the page for the next button, we use setCurrentPage(currentPage + 1). Include event listeners in the window for the next and previous buttons.load():
Disable Page Navigation Buttons
We also want to provide a disable option on the next and previous buttons only:
- If the current page is the first one, disable the previous button.
- If this is the last page and there are no further pages, turn off the next button.
Finally, pass the handlePageButtonsStatus() function into the setCurrentPage() function so we check the navigation every time a new page is clicked:
FAQs
Q. What is pagination in JavaScript?
A. Pagination is a technique to break a large data set into smaller, more manageable pieces or pages. It is commonly used in web applications to display a limited number of items per page and allow users to navigate the pages.
Q. How do I implement basic pagination in JavaScript?
A. To implement basic pagination, you can follow these steps: Determine the total number of items and how many items you want to display per page. Calculate the total number of pages by dividing the total items by items per page. Create navigation controls (e.g., previous and next buttons) to switch between pages. Use JavaScript to load and display the items for the current page dynamically.
Q. Can I implement pagination without using a library or framework?
A. You can implement pagination without relying on external libraries or frameworks. Basic pagination can be achieved using plain JavaScript and HTML/CSS. However, pagination libraries (e.g., simplePagination.js, pagination.js) can simplify the process and offer additional features.
Q. How do I handle user interactions with pagination controls?
A. To detect user interactions, you can add event listeners to pagination controls (e.g., previous and next buttons). When a user clicks on a control, update the current page and reload the items accordingly.
Conclusion
To implement pagination in JavaScript, you can follow these steps:
- Create an array or retrieve data from an API containing the items to be paginated. Define the number of items to display per page and calculate the total number of pages.
- Dynamically generate pagination controls (e.g., previous, next, page numbers) in your HTML. Implement logic to display the relevant subset of items based on the current page.
- Update the UI and handle user interactions to navigate between pages, ensuring a seamless browsing experience for users.
- Pagination enhances user experience by breaking down content into manageable chunks, improving load times, and simplifying navigation through large datasets.