Palindrome in JavaScript
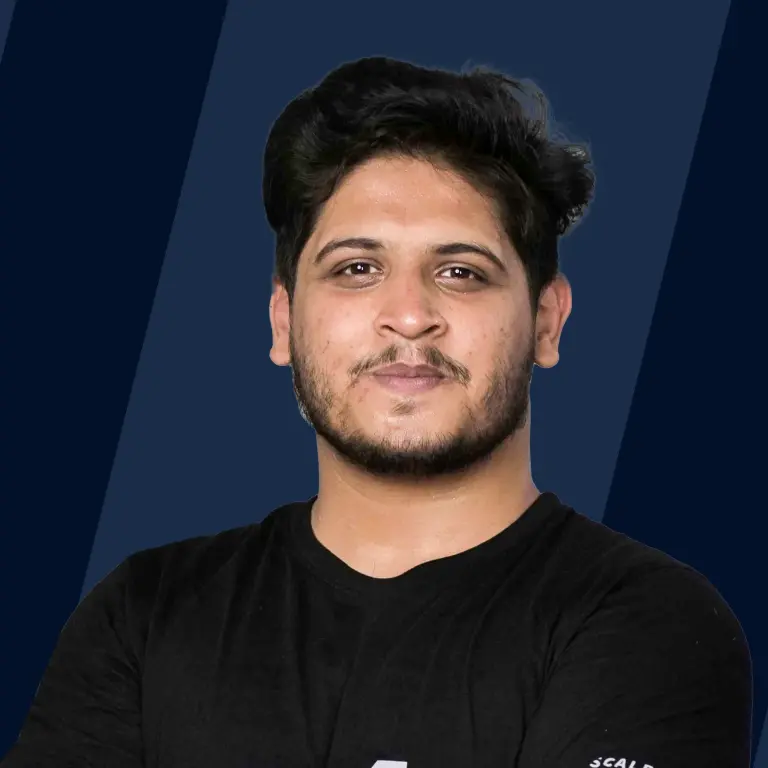
A sequence of characters that reads the same either from backwards or forward is known as Palindrome. Not necessarily characters, but words, sentences, numbers etc., can also be Palindrome. In this article, we will learn how can we code the Palindrome in Javascript. We will also learn various approaches to code Palindrome in JavaScript.
The Palindrome Problem
A palindrome is a word, phrase, number, or any other sequence of characters that is read (or spelt) the same backwards or forward.
For example, the above string in the image is a palindrome string. However, please note that palindrome also takes spaces, punctuations etc., into consideration. The above image is just an example of a palindromic string, which reads the same from backwards or forward.
Examples of palindrome words may include: radar, madam, level, refer, etc. Some examples of palindrome numbers may include 12321, 101, 5115, etc.
Consider below given is the Palindrome Problem --
Given a String, return "true" if the String is a palindrome, else return "false".
Below given is some input and output samples for our problem statement.
Input 1:
Output 1:
Input 2:
Output 2:
So, as stated in the problem, we will be given a string as the input, and we have to process the string and come up with the answer to whether the string is palindrome or not.
Palindrome Algorithm
There are different methods to code palindrome in javascript, and for each of them, we have a different algorithm. Going further, we will discuss all the approaches to code palindrome in javascript. However, let us first see the algorithm using the most basic and simplest approach to code palindrome in Javascript.
Palindrome Algorithm:
- Take the input from the user
- Use a temporary variable (say temp) to store the input
- Find the reverse of temp
- Compare the given input with temp
- If temp and the input is the same, return True
- If they are not the same, return False
The above algorithm works by reversing our given input and verifying whether the input and the reversed input are the same. If they are the same, then they are palindromes, and we will return true, otherwise, they are not palindromes, and false will be returned from our code.
Examples
We already learned our problem statement, and its algorithm. Let us now discuss the various logic to code it.
Check the Palindrome number and string by Accepting the Number through the Prompt Box
In this program, we will learn how we can check a palindrome number by accepting it through the prompt box.
Code:
Output:
Our prompt:
Our result:
Explanation: Here in the above code, we have tried to find out the palindrome of a number or a string by taking input through our prompt and returning our answer. We are finding out the palindrome of a string(or number) by dividing our given input into halves, and then we compare each of the halves, taking one character each from the front and end. The above method is also known as the two-pointer method, where we are pointing to the two ends of our string and comparing them. If, at any point, our characters of the string don't match, we will alert the message "Not palindrome" and return it from our function. Otherwise, if all the characters match till we reach the mid of the string, then we declare the given string as palindrome and alert the appropriate message.
Check Palindrome String Using Built-in Functions
Having seen a general approach to finding out whether a string is a palindrome in javascript, let us now also explore some built-in methods to check the same.
Using the split(), reverse() and join() Methods
Before determining how to find out palindrome in JavaScript using the split(), reverse(), and join() methods, let us get a small intro about these three methods in javascript.
1. Split() Method The split() method in javascript splits a string into an array of substrings and returns the new array(which is split). However, the split method does not modify the original string. If we use any separator (such as " "), then the string is split between the words.
Code:
Output:
Explanation: In the above code, we used the split method to split our given string on the basis of our separator: " ".
2. reverse() Method The reverse() method in javascript reverses the order of the elements in any given array. However, it is important to note that the reverse method overwrites(or modifies) the original array. Hence, we do not get any new array after applying the reverse() method, but the original array is reversed. Code:
Output:
Explanation: In the above example, we applied our reverse method to our array and we saw how it modified our original array.
3. join() Method The join() method in JavaScript, joins an array into a string and returns it. However, the join() method does not modify the original array. We can also provide any separator to our join method based on which it will join our array. By default, comma (,) is our separator for the join() method.
Code:
Output:
Explanation:
In the above examples, we joined an array of strings into a single string, based on the separator we provided for joining them.
After getting a brief introduction about what each of these methods can do, let us now get back to our code. We will be combining all the above methods, to find palindrome in Javascript. Let us look into the code below.
Code:
Output:
Explanation: In the above example, we have made a function that checks if the given string is a palindrome. Initially, we split() the string using our separator " ". Splitting the string gives us an array, over which we apply our reverse() function. And finally, we join the reversed array using the join() method. By doing this, we achieve our reversed string. Finally, we compare the original string with the reversed string. If they are the same, then the array is palindrome otherwise it is not a palindrome.
Check if a Number is a Palindrome in JavaScript
To this point, we have dealt with strings. We were given some strings as input, and we had to check whether they were palindrome or not. So, basically, our logic has been centred near strings. But now, we will explore the code when the input is a number.
Suppose, now, a number is given to us as an input. So here, we will learn how to verify if a given number is palindrome or not in javascript. Let us look into the code, after which we will do our deep analysis. Code:
Output:
Explanation: In our above code, initially, we assign our number (given as input) to a temporary variable temp. Going further, we also have 3 variables remainder, temp, and reversedNumber, which we use to calculate the reverse of our given number. After that, we write our reverse logic upon the number and finally store the reversed number into the variable reversedNumber. Finally, we check this reversedNumber with our input, and if both of them are equal, we declare the number as a palindrome.
Checking Palindrome Using Recursion.
Let’s go through how we can re-implement the isPalindrome() function using recursion.
Code:
Output:
Explanation: To check palindrome in a string recursively, we have first defined our base cases. For example, if a string has a length = 1, then obviously it is a palindrome. We have added one more base case if the length of the string is 2. In that case, we simply check if both the characters are the same, then only they can be palindromes. Finally, we write our recursive code.
In the recursive code, we have used the slice() method. The slice() method returns selected elements in an array as a new array. It selects from a given start up to a (not inclusive) given end. Every time, we check if the beginning character of our string is the same as the ending character. And then recursively pass the next character from the beginning and the previous character from the end of our string using the slice() method - str.slice(1,-1) to our recursive function checkPalindrome.
Check Whether the Entered String is Palindrome or Not in JavaScript
Let us now finally learn our last approach of checking whether a string, which we get through our input, is Palindrome or not. For this code, our algorithm will be as stated below:
- We will iterate through the string from the forward and the backward, till we reach the mid of the string.
- If during our iteration, all of our characters in the string match, then we will return True
- If any character doesn't match at any point, we will straightaway return false
- We will return our answer based on what we got after our iteration.
Let us also see the code for the algorithm we stated above.
Code:
Output:
Explanation:
In the above code, we are checking whether a string is a palindrome or not. For that, we are passing our string to the function test_palindrome. This function passes the string to check_palindrome. check_palindrome is the function that will check if the string is palindrome or not.
In check_palindrome, we first run a loop through half the length of the string. We do this because we are mainly checking if the characters from the front are equal to the character from the end. Hence, once we reach the mid of the string, it would be decided whether they are equal or not. We loop through each character from back and front and compare. If, at any point, the characters are unequal, we directly return false to our calling function. If our loop hasn't returned false, then we can conclude that our string is a palindrome and hence return true.
Conclusion
- Palindrome are strings, numbers or anything that reads the same from the front and backward.
- We can check for palindrome by comparing our input string with the reverse of the original string. If they are the same, then they are palindromes, otherwise not.
- We can also check Palindrome string using built-in Functions such as split(), reverse(), and join().
- split() divides (or breaks) the string and returns an array. After that, reverse() is applied over that array to reverse its order. Finally, we apply join() to join that array into a string again. We compare this result with our original string to determine whether it is palindrome or not.
- Palindrome can be checked using recursion, where we recursively slice the string and check if their beginning and end characters are equal.
- To check palindrome numbers, we can reverse the given number using the normal reverse operation we do for numbers. Later, we can compare the number with the original number for the result.
- Another efficient way to test whether a string is a palindrome is by running a loop through half the length of the string and comparing each character from the beginning and end of the string.